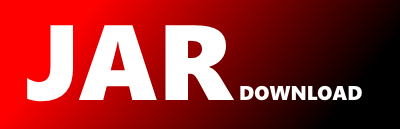
javaslang.collection.Stack Maven / Gradle / Ivy
/* / \____ _ _ ____ ______ / \ ____ __ _______
* / / \/ \ / \/ \ / /\__\/ // \/ \ // /\__\ JΛVΛSLΛNG
* _/ / /\ \ \/ / /\ \\__\\ \ // /\ \ /\\/ \ /__\ \ Copyright 2014-2016 Javaslang, http://javaslang.io
* /___/\_/ \_/\____/\_/ \_/\__\/__/\__\_/ \_// \__/\_____/ Licensed under the Apache License, Version 2.0
*/
package javaslang.collection;
import javaslang.Tuple2;
import javaslang.control.Option;
/**
* An immutable {@code Stack} stores elements allowing a last-in-first-out (LIFO) retrieval.
*
* {@link List} extends {@code Stack}, so use one of {@code List}'s static factory methods to obtain a {@code Stack}.
*
* Stack API:
*
*
* - {@link #peek()}
* - {@link #peekOption()}
* - {@link #pop()}
* - {@link #popOption()}
* - {@link #pop2()}
* - {@link #pop2Option()}
* - {@link #push(Object)}
* - {@link #push(Object[])}
* - {@link #pushAll(Iterable)}
*
*
* See Okasaki, Chris: Purely Functional Data Structures (p. 7 ff.). Cambridge, 2003.
*
* @param component type
* @author Daniel Dietrich
* @since 2.0.0
*/
public interface Stack {
/**
* Narrows a widened {@code Stack extends T>} to {@code Stack}
* by performing a type safe-cast. This is eligible because immutable/read-only
* collections are covariant.
*
* @param stack A {@code Stack}.
* @param Component type of the {@code Stack}.
* @return the given {@code stack} instance as narrowed type {@code Stack}.
*/
@SuppressWarnings("unchecked")
static Stack narrow(Stack extends T> stack) {
return (Stack) stack;
}
/**
* Checks if this Stack is empty.
*
* @return true, if this is empty, false otherwise.
*/
boolean isEmpty();
/**
* Returns the head element without modifying the Stack.
*
* @return the first element
* @throws java.util.NoSuchElementException if this Stack is empty
*/
T peek();
/**
* Returns the head element without modifying the Stack.
*
* @return {@code None} if this Stack is empty, otherwise a {@code Some} containing the head element
*/
Option peekOption();
/**
* Removes the head element from this Stack.
*
* @return the elements of this Stack without the head element
* @throws java.util.NoSuchElementException if this Stack is empty
*/
Stack pop();
/**
* Removes the head element from this Stack.
*
* @return {@code None} if this Stack is empty, otherwise a {@code Some} containing the elements of this Stack without the head element
*/
Option extends Stack> popOption();
/**
* Removes the head element from this Stack.
*
* @return a tuple containing the head element and the remaining elements of this Stack
* @throws java.util.NoSuchElementException if this Stack is empty
*/
Tuple2> pop2();
/**
* Removes the head element from this Stack.
*
* @return {@code None} if this Stack is empty, otherwise {@code Some} {@code Tuple} containing the head element and the remaining elements of this Stack
*/
Option extends Tuple2>> pop2Option();
/**
* Pushes a new element on top of this Stack.
*
* @param element The new element
* @return a new {@code Stack} instance, containing the new element on top of this Stack
*/
Stack push(T element);
/**
* Pushes the given elements on top of this Stack. A Stack has LIFO order, i.e. the last of the given elements is
* the first which will be retrieved.
*
* @param elements Elements, may be empty
* @return a new {@code Stack} instance, containing the new elements on top of this Stack
* @throws NullPointerException if elements is null
*/
@SuppressWarnings("unchecked")
Stack push(T... elements);
/**
* Pushes the given elements on top of this Stack. A Stack has LIFO order, i.e. the last of the given elements is
* the first which will be retrieved.
*
* @param elements An Iterable of elements, may be empty
* @return a new {@code Stack} instance, containing the new elements on top of this Stack
* @throws NullPointerException if elements is null
*/
Stack pushAll(Iterable elements);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy