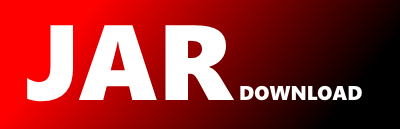
org.skife.config.ExactMatchEnumCoercible Maven / Gradle / Ivy
package org.skife.config;
import java.lang.reflect.Method;
public class ExactMatchEnumCoercible implements Coercible
© 2015 - 2025 Weber Informatics LLC | Privacy Policy
package org.skife.config;
import java.lang.reflect.Method;
public class ExactMatchEnumCoercible implements Coercible