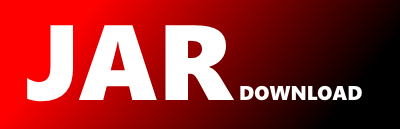
com.bpodgursky.jbool_expressions.parsers.BooleanExprLexer Maven / Gradle / Ivy
// $ANTLR 3.3 Nov 30, 2010 12:46:29 com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g 2014-02-09 14:45:32
package com.bpodgursky.jbool_expressions.parsers;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
public class BooleanExprLexer extends Lexer {
public static final int EOF=-1;
public static final int LPAREN=4;
public static final int RPAREN=5;
public static final int AND=6;
public static final int OR=7;
public static final int NOT=8;
public static final int NAME=9;
public static final int QUOTED_NAME=10;
public static final int WS=11;
public static final int TRUE=12;
public static final int FALSE=13;
// delegates
// delegators
public BooleanExprLexer() {;}
public BooleanExprLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public BooleanExprLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g"; }
// $ANTLR start "LPAREN"
public final void mLPAREN() throws RecognitionException {
try {
int _type = LPAREN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:17:8: ( '(' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:17:10: '('
{
match('(');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "LPAREN"
// $ANTLR start "RPAREN"
public final void mRPAREN() throws RecognitionException {
try {
int _type = RPAREN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:18:8: ( ')' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:18:10: ')'
{
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "RPAREN"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:19:5: ( '&' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:19:7: '&'
{
match('&');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "AND"
// $ANTLR start "OR"
public final void mOR() throws RecognitionException {
try {
int _type = OR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:20:4: ( '|' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:20:6: '|'
{
match('|');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "OR"
// $ANTLR start "NOT"
public final void mNOT() throws RecognitionException {
try {
int _type = NOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:21:5: ( '!' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:21:7: '!'
{
match('!');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "NOT"
// $ANTLR start "NAME"
public final void mNAME() throws RecognitionException {
try {
int _type = NAME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:22:6: ( ( 'A' .. 'Z' )+ )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:22:8: ( 'A' .. 'Z' )+
{
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:22:8: ( 'A' .. 'Z' )+
int cnt1=0;
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:22:9: 'A' .. 'Z'
{
matchRange('A','Z');
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee =
new EarlyExitException(1, input);
throw eee;
}
cnt1++;
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "NAME"
// $ANTLR start "QUOTED_NAME"
public final void mQUOTED_NAME() throws RecognitionException {
try {
int _type = QUOTED_NAME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:23:13: ( '\"' (~ ( '\\r' | '\\n' | '\"' ) )+ '\"' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:23:15: '\"' (~ ( '\\r' | '\\n' | '\"' ) )+ '\"'
{
match('\"');
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:23:18: (~ ( '\\r' | '\\n' | '\"' ) )+
int cnt2=0;
loop2:
do {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0>='\u0000' && LA2_0<='\t')||(LA2_0>='\u000B' && LA2_0<='\f')||(LA2_0>='\u000E' && LA2_0<='!')||(LA2_0>='#' && LA2_0<='\uFFFF')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:23:18: ~ ( '\\r' | '\\n' | '\"' )
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='\t')||(input.LA(1)>='\u000B' && input.LA(1)<='\f')||(input.LA(1)>='\u000E' && input.LA(1)<='!')||(input.LA(1)>='#' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
if ( cnt2 >= 1 ) break loop2;
EarlyExitException eee =
new EarlyExitException(2, input);
throw eee;
}
cnt2++;
} while (true);
match('\"');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "QUOTED_NAME"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:24:4: ( ( ' ' | '\\t' | '\\r' | '\\n' )+ )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:24:6: ( ' ' | '\\t' | '\\r' | '\\n' )+
{
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:24:6: ( ' ' | '\\t' | '\\r' | '\\n' )+
int cnt3=0;
loop3:
do {
int alt3=2;
switch ( input.LA(1) ) {
case '\t':
case '\n':
case '\r':
case ' ':
{
alt3=1;
}
break;
}
switch (alt3) {
case 1 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:
{
if ( (input.LA(1)>='\t' && input.LA(1)<='\n')||input.LA(1)=='\r'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee =
new EarlyExitException(3, input);
throw eee;
}
cnt3++;
} while (true);
_channel = HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "WS"
// $ANTLR start "TRUE"
public final void mTRUE() throws RecognitionException {
try {
int _type = TRUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:25:6: ( 'true' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:25:8: 'true'
{
match("true");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "TRUE"
// $ANTLR start "FALSE"
public final void mFALSE() throws RecognitionException {
try {
int _type = FALSE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:26:7: ( 'false' )
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:26:9: 'false'
{
match("false");
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "FALSE"
public void mTokens() throws RecognitionException {
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:8: ( LPAREN | RPAREN | AND | OR | NOT | NAME | QUOTED_NAME | WS | TRUE | FALSE )
int alt4=10;
switch ( input.LA(1) ) {
case '(':
{
alt4=1;
}
break;
case ')':
{
alt4=2;
}
break;
case '&':
{
alt4=3;
}
break;
case '|':
{
alt4=4;
}
break;
case '!':
{
alt4=5;
}
break;
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
{
alt4=6;
}
break;
case '\"':
{
alt4=7;
}
break;
case '\t':
case '\n':
case '\r':
case ' ':
{
alt4=8;
}
break;
case 't':
{
alt4=9;
}
break;
case 'f':
{
alt4=10;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:10: LPAREN
{
mLPAREN();
}
break;
case 2 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:17: RPAREN
{
mRPAREN();
}
break;
case 3 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:24: AND
{
mAND();
}
break;
case 4 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:28: OR
{
mOR();
}
break;
case 5 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:31: NOT
{
mNOT();
}
break;
case 6 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:35: NAME
{
mNAME();
}
break;
case 7 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:40: QUOTED_NAME
{
mQUOTED_NAME();
}
break;
case 8 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:52: WS
{
mWS();
}
break;
case 9 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:55: TRUE
{
mTRUE();
}
break;
case 10 :
// com/bpodgursky/jbool_expressions/parsers/BooleanExpr.g:1:60: FALSE
{
mFALSE();
}
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy