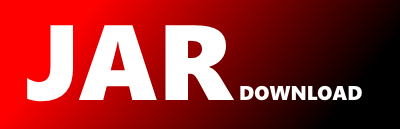
org.skife.config.DefaultCoercibles Maven / Gradle / Ivy
package org.skife.config;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.net.URI;
import java.util.HashMap;
import java.util.Map;
final class DefaultCoercibles
{
private DefaultCoercibles()
{
}
public static final Coercible> CASE_INSENSITIVE_ENUM_COERCIBLE = new CaseInsensitiveEnumCoercible();
static final Coercible BOOLEAN_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Boolean.class.isAssignableFrom(clazz) || Boolean.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.BOOLEAN_COERCER;
}
return null;
}
};
static final Coercible BYTE_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Byte.class.isAssignableFrom(clazz) || Byte.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.BYTE_COERCER;
}
return null;
}
};
static final Coercible SHORT_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Short.class.isAssignableFrom(clazz) || Short.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.SHORT_COERCER;
}
return null;
}
};
static final Coercible INTEGER_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Integer.class.isAssignableFrom(clazz) || Integer.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.INTEGER_COERCER;
}
return null;
}
};
static final Coercible LONG_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Long.class.isAssignableFrom(clazz) || Long.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.LONG_COERCER;
}
return null;
}
};
static final Coercible FLOAT_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Float.class.isAssignableFrom(clazz) || Float.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.FLOAT_COERCER;
}
return null;
}
};
static final Coercible DOUBLE_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (Double.class.isAssignableFrom(clazz) || Double.TYPE.isAssignableFrom(clazz)) {
return DefaultCoercibles.DOUBLE_COERCER;
}
return null;
}
};
static final Coercible STRING_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (String.class.equals(clazz)) {
return DefaultCoercibles.STRING_COERCER;
}
return null;
}
};
static final Coercible URI_COERCIBLE = new Coercible() {
public Coercer accept(final Class> clazz) {
if (URI.class.equals(clazz)) {
return DefaultCoercibles.URI_COERCER;
}
return null;
}
};
/**
* A Coercible that accepts any type with a static valueOf(String)
method.
*/
static final Coercible> VALUE_OF_COERCIBLE = new Coercible
© 2015 - 2025 Weber Informatics LLC | Privacy Policy