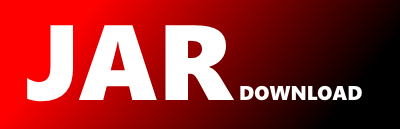
javaslang.control.Either Maven / Gradle / Ivy
/* / \____ _ _ ____ ______ / \ ____ __ _______
* / / \/ \ / \/ \ / /\__\/ // \/ \ // /\__\ JΛVΛSLΛNG
* _/ / /\ \ \/ / /\ \\__\\ \ // /\ \ /\\/ \ /__\ \ Copyright 2014-2016 Javaslang, http://javaslang.io
* /___/\_/ \_/\____/\_/ \_/\__\/__/\__\_/ \_// \__/\_____/ Licensed under the Apache License, Version 2.0
*/
package javaslang.control;
import javaslang.API;
import javaslang.Value;
import javaslang.collection.Iterator;
import java.io.Serializable;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* Either represents a value of two possible types. An Either is either a {@link Left} or a
* {@link Right}.
*
* If the given Either is a Right and projected to a Left, the Left operations have no effect on the Right value.
* If the given Either is a Left and projected to a Right, the Right operations have no effect on the Left value.
* If a Left is projected to a Left or a Right is projected to a Right, the operations have an effect.
*
* Example: A compute() function, which results either in an Integer value (in the case of success) or
* in an error message of type String (in the case of failure). By convention the success case is Right and the failure
* is Left.
*
*
*
* Either<String,Integer> value = compute().right().map(i -> i * 2).toEither();
*
*
*
* If the result of compute() is Right(1), the value is Right(2).
* If the result of compute() is Left("error), the value is Left("error").
*
* @param The type of the Left value of an Either.
* @param The type of the Right value of an Either.
* @author Daniel Dietrich
* @since 1.0.0
*/
public interface Either extends Value {
/**
* Constructs a {@link Right}
*
* @param right The value.
* @param Type of left value.
* @param Type of right value.
* @return A new {@code Right} instance.
*/
static Either right(R right) {
return new Right<>(right);
}
/**
* Constructs a {@link Left}
*
* @param left The value.
* @param Type of left value.
* @param Type of right value.
* @return A new {@code Left} instance.
*/
static Either left(L left) {
return new Left<>(left);
}
/**
* Narrows a widened {@code Either extends L, ? extends R>} to {@code Either}
* by performing a type safe-cast. This is eligible because immutable/read-only
* collections are covariant.
*
* @param either A {@code Either}.
* @param Type of left value.
* @param Type of right value.
* @return the given {@code either} instance as narrowed type {@code Either}.
*/
@SuppressWarnings("unchecked")
static Either narrow(Either extends L, ? extends R> either) {
return (Either) either;
}
/**
* Returns the left value.
*
* @return The left value.
* @throws NoSuchElementException if this is a {@code Right}.
*/
L getLeft();
/**
* Returns whether this Either is a Left.
*
* @return true, if this is a Left, false otherwise
*/
boolean isLeft();
/**
* Returns whether this Either is a Right.
*
* @return true, if this is a Right, false otherwise
*/
boolean isRight();
/**
* Returns a LeftProjection of this Either.
*
* @return a new LeftProjection of this
*/
default LeftProjection left() {
return new LeftProjection<>(this);
}
/**
* Returns a RightProjection of this Either.
*
* @return a new RightProjection of this
*/
default RightProjection right() {
return new RightProjection<>(this);
}
/**
* Maps either the left or the right side of this disjunction.
*
* @param leftMapper maps the left value if this is a Left
* @param rightMapper maps the right value if this is a Right
* @param The new left type of the resulting Either
* @param The new right type of the resulting Either
* @return A new Either instance
*/
default Either bimap(Function super L, ? extends X> leftMapper, Function super R, ? extends Y> rightMapper) {
Objects.requireNonNull(leftMapper, "leftMapper is null");
Objects.requireNonNull(rightMapper, "rightMapper is null");
if (isRight()) {
return new Right<>(rightMapper.apply(get()));
} else {
return new Left<>(leftMapper.apply(getLeft()));
}
}
/**
* Folds either the left or the right side of this disjunction.
*
* @param leftMapper maps the left value if this is a Left
* @param rightMapper maps the right value if this is a Right
* @param type of the folded value
* @return A value of type U
*/
default U fold(Function super L, ? extends U> leftMapper, Function super R, ? extends U> rightMapper) {
Objects.requireNonNull(leftMapper, "leftMapper is null");
Objects.requireNonNull(rightMapper, "rightMapper is null");
if (isRight()) {
return rightMapper.apply(get());
} else {
return leftMapper.apply(getLeft());
}
}
/**
* Gets the Right value or an alternate value, if the projected Either is a Left.
*
* @param other a function which converts a Left value to an alternative Right value
* @return the right value, if the underlying Either is a Right or else the alternative Right value provided by
* {@code other} by applying the Left value.
*/
default R getOrElseGet(Function super L, ? extends R> other) {
Objects.requireNonNull(other, "other is null");
if (isRight()) {
return get();
} else {
return other.apply(getLeft());
}
}
/**
* Runs an action in the case this is a projection on a Left value.
*
* @param action an action which consumes a Left value
*/
default void orElseRun(Consumer super L> action) {
Objects.requireNonNull(action, "action is null");
if (isLeft()) {
action.accept(getLeft());
}
}
/**
* Gets the Right value or throws, if the projected Either is a Left.
*
* @param a throwable type
* @param exceptionFunction a function which creates an exception based on a Left value
* @return the right value, if the underlying Either is a Right or else throws the exception provided by
* {@code exceptionFunction} by applying the Left value.
* @throws X if the projected Either is a Left
*/
default R getOrElseThrow(Function super L, X> exceptionFunction) throws X {
Objects.requireNonNull(exceptionFunction, "exceptionFunction is null");
if (isRight()) {
return get();
} else {
throw exceptionFunction.apply(getLeft());
}
}
/**
* Converts a {@code Left} to a {@code Right} vice versa by wrapping the value in a new type.
*
* @return a new {@code Either}
*/
default Either swap() {
if (isRight()) {
return new Left<>(get());
} else {
return new Right<>(getLeft());
}
}
// -- Adjusted return types of Monad methods
/**
* FlatMaps this right-biased Either.
*
* @param mapper A mapper
* @param Component type of the mapped right value
* @return this as {@code Either} if this is a Left, otherwise the right mapping result
* @throws NullPointerException if {@code mapper} is null
*/
@SuppressWarnings("unchecked")
default Either flatMap(Function super R, ? extends Either> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (isRight()) {
return (Either) mapper.apply(get());
} else {
return (Either) this;
}
}
/**
* Maps this right-biased Either.
*
* @param mapper A mapper
* @param Component type of the mapped right value
* @return a mapped {@code Monad}
* @throws NullPointerException if {@code mapper} is null
*/
@SuppressWarnings("unchecked")
@Override
default Either map(Function super R, ? extends U> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (isRight()) {
return Either.right(mapper.apply(get()));
} else {
return (Either) this;
}
}
/**
* Maps this right-biased Either.
*
* @param leftMapper A mapper
* @param Component type of the mapped right value
* @return a mapped {@code Monad}
* @throws NullPointerException if {@code mapper} is null
*/
@SuppressWarnings("unchecked")
default Either mapLeft(Function super L, ? extends U> leftMapper) {
Objects.requireNonNull(leftMapper, "mapper is null");
if (isLeft()) {
return Either.left(leftMapper.apply(getLeft()));
} else {
return (Either) this;
}
}
// -- Adjusted return types of Value methods
/**
* Filters this right-biased {@code Either} by testing a predicate.
*
*
* @param predicate A predicate
* @return a new {@code Option} instance
* @throws NullPointerException if {@code predicate} is null
*/
default Option> filter(Predicate super R> predicate) {
Objects.requireNonNull(predicate, "predicate is null");
return isLeft() || predicate.test(get()) ? Option.some(this) : Option.none();
}
/**
* Gets the right value if this is a {@code Right} or throws if this is a {@code Left}.
*
* @return the right value
* @throws NoSuchElementException if this is a Left.
*/
@Override
R get();
@Override
default boolean isEmpty() {
return isLeft();
}
@SuppressWarnings("unchecked")
default Either orElse(Either extends L, ? extends R> other) {
Objects.requireNonNull(other, "other is null");
return isRight() ? this : (Either) other;
}
@SuppressWarnings("unchecked")
default Either orElse(Supplier extends Either extends L, ? extends R>> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return isRight() ? this : (Either) supplier.get();
}
/**
* A right-biased {@code Either} is single-valued.
*
* @return {@code true}
*/
@Override
default boolean isSingleValued() {
return true;
}
@Override
default Iterator iterator() {
if (isRight()) {
return Iterator.of(get());
} else {
return Iterator.empty();
}
}
@Override
default Either peek(Consumer super R> action) {
Objects.requireNonNull(action, "action is null");
if (isRight()) {
action.accept(get());
}
return this;
}
// -- Object.*
@Override
boolean equals(Object o);
@Override
int hashCode();
@Override
String toString();
// -- Left/Right projections
/**
* A left projection of an Either.
*
* @param The type of the Left value of an Either.
* @param The type of the Right value of an Either.
* @since 1.0.0
*/
final class LeftProjection implements Value {
private final Either either;
private LeftProjection(Either either) {
this.either = either;
}
public LeftProjection bimap(Function super L, ? extends L2> leftMapper, Function super R, ? extends R2> rightMapper) {
return either. bimap(leftMapper, rightMapper).left();
}
@Override
public boolean isEmpty() {
return either.isRight();
}
/**
* A {@code LeftProjection} is single-valued.
*
* @return {@code true}
*/
@Override
public boolean isSingleValued() {
return true;
}
/**
* Gets the Left value or throws.
*
* @return the left value, if the underlying Either is a Left
* @throws NoSuchElementException if the underlying either of this LeftProjection is a Right
*/
@Override
public L get() {
if (either.isLeft()) {
return either.getLeft();
} else {
throw new NoSuchElementException("LeftProjection.get() on Right");
}
}
@SuppressWarnings("unchecked")
public LeftProjection orElse(LeftProjection extends L, ? extends R> other) {
Objects.requireNonNull(other, "other is null");
return either.isLeft() ? this : (LeftProjection) other;
}
@SuppressWarnings("unchecked")
public LeftProjection orElse(Supplier extends LeftProjection extends L, ? extends R>> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return either.isLeft() ? this : (LeftProjection) supplier.get();
}
/**
* Gets the Left value or an alternate value, if the projected Either is a Right.
*
* @param other an alternative value
* @return the left value, if the underlying Either is a Left or else {@code other}
* @throws NoSuchElementException if the underlying either of this LeftProjection is a Right
*/
@Override
public L getOrElse(L other) {
return either.isLeft() ? either.getLeft() : other;
}
/**
* Gets the Left value or an alternate value, if the projected Either is a Right.
*
* @param other a function which converts a Right value to an alternative Left value
* @return the left value, if the underlying Either is a Left or else the alternative Left value provided by
* {@code other} by applying the Right value.
*/
public L getOrElseGet(Function super R, ? extends L> other) {
Objects.requireNonNull(other, "other is null");
if (either.isLeft()) {
return either.getLeft();
} else {
return other.apply(either.get());
}
}
/**
* Runs an action in the case this is a projection on a Right value.
*
* @param action an action which consumes a Right value
*/
public void orElseRun(Consumer super R> action) {
Objects.requireNonNull(action, "action is null");
if (either.isRight()) {
action.accept(either.get());
}
}
/**
* Gets the Left value or throws, if the projected Either is a Right.
*
* @param a throwable type
* @param exceptionFunction a function which creates an exception based on a Right value
* @return the left value, if the underlying Either is a Left or else throws the exception provided by
* {@code exceptionFunction} by applying the Right value.
* @throws X if the projected Either is a Right
*/
public L getOrElseThrow(Function super R, X> exceptionFunction) throws X {
Objects.requireNonNull(exceptionFunction, "exceptionFunction is null");
if (either.isLeft()) {
return either.getLeft();
} else {
throw exceptionFunction.apply(either.get());
}
}
/**
* Returns the underlying either of this projection.
*
* @return the underlying either
*/
public Either toEither() {
return either;
}
/**
* Returns {@code Some} value of type L if this is a left projection of a Left value and the predicate
* applies to the underlying value.
*
* @param predicate A predicate
* @return A new Option
*/
public Option> filter(Predicate super L> predicate) {
Objects.requireNonNull(predicate, "predicate is null");
return either.isRight() || predicate.test(either.getLeft()) ? Option.some(this) : Option.none();
}
/**
* FlatMaps this LeftProjection.
*
* @param mapper A mapper
* @param Component type of the mapped left value
* @return this as {@code LeftProjection} if a Right is underlying, otherwise a the mapping result of the left value.
* @throws NullPointerException if {@code mapper} is null
*/
@SuppressWarnings("unchecked")
public LeftProjection flatMap(Function super L, ? extends LeftProjection extends U, R>> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (either.isLeft()) {
return (LeftProjection) mapper.apply(either.getLeft());
} else {
return (LeftProjection) this;
}
}
/**
* Maps the left value if the projected Either is a Left.
*
* @param mapper A mapper which takes a left value and returns a value of type U
* @param The new type of a Left value
* @return A new LeftProjection
*/
@SuppressWarnings("unchecked")
@Override
public LeftProjection map(Function super L, ? extends U> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (either.isLeft()) {
return either.mapLeft((Function) mapper).left();
} else {
return (LeftProjection) this;
}
}
/**
* Applies the given action to the value if the projected either is a Left. Otherwise nothing happens.
*
* @param action An action which takes a left value
* @return this LeftProjection
*/
@Override
public LeftProjection peek(Consumer super L> action) {
Objects.requireNonNull(action, "action is null");
if (either.isLeft()) {
action.accept(either.getLeft());
}
return this;
}
/**
* Transforms this {@code LeftProjection}.
*
* @param f A transformation
* @param Type of transformation result
* @return An instance of type {@code U}
* @throws NullPointerException if {@code f} is null
*/
public U transform(Function super LeftProjection, ? extends U> f) {
Objects.requireNonNull(f, "f is null");
return f.apply(this);
}
@Override
public Iterator iterator() {
if (either.isLeft()) {
return Iterator.of(either.getLeft());
} else {
return Iterator.empty();
}
}
@Override
public boolean equals(Object obj) {
return (obj == this) || (obj instanceof LeftProjection && Objects.equals(either, ((LeftProjection, ?>) obj).either));
}
@Override
public int hashCode() {
return either.hashCode();
}
@Override
public String stringPrefix() {
return "LeftProjection";
}
@Override
public String toString() {
return stringPrefix() + "(" + either + ")";
}
}
/**
* A right projection of an Either.
*
* @param The type of the Left value of an Either.
* @param The type of the Right value of an Either.
* @since 1.0.0
*/
final class RightProjection implements Value {
private final Either either;
private RightProjection(Either either) {
this.either = either;
}
public RightProjection bimap(Function super L, ? extends L2> leftMapper, Function super R, ? extends R2> rightMapper) {
return either. bimap(leftMapper, rightMapper).right();
}
@Override
public boolean isEmpty() {
return either.isLeft();
}
/**
* A {@code RightProjection} is single-valued.
*
* @return {@code true}
*/
@Override
public boolean isSingleValued() {
return true;
}
/**
* Gets the Right value or throws.
*
* @return the left value, if the underlying Either is a Right
* @throws NoSuchElementException if the underlying either of this RightProjection is a Left
*/
@Override
public R get() {
if (either.isRight()) {
return either.get();
} else {
throw new NoSuchElementException("RightProjection.get() on Left");
}
}
@SuppressWarnings("unchecked")
public RightProjection orElse(RightProjection extends L, ? extends R> other) {
Objects.requireNonNull(other, "other is null");
return either.isRight() ? this : (RightProjection) other;
}
@SuppressWarnings("unchecked")
public RightProjection orElse(Supplier extends RightProjection extends L, ? extends R>> supplier) {
Objects.requireNonNull(supplier, "supplier is null");
return either.isRight() ? this : (RightProjection) supplier.get();
}
/**
* Gets the Right value or an alternate value, if the projected Either is a Left.
*
* @param other an alternative value
* @return the right value, if the underlying Either is a Right or else {@code other}
* @throws NoSuchElementException if the underlying either of this RightProjection is a Left
*/
@Override
public R getOrElse(R other) {
return either.getOrElse(other);
}
/**
* Gets the Right value or an alternate value, if the projected Either is a Left.
*
* @param other a function which converts a Left value to an alternative Right value
* @return the right value, if the underlying Either is a Right or else the alternative Right value provided by
* {@code other} by applying the Left value.
*/
public R getOrElseGet(Function super L, ? extends R> other) {
Objects.requireNonNull(other, "other is null");
return either.getOrElseGet(other);
}
/**
* Runs an action in the case this is a projection on a Left value.
*
* @param action an action which consumes a Left value
*/
public void orElseRun(Consumer super L> action) {
Objects.requireNonNull(action, "action is null");
either.orElseRun(action);
}
/**
* Gets the Right value or throws, if the projected Either is a Left.
*
* @param a throwable type
* @param exceptionFunction a function which creates an exception based on a Left value
* @return the right value, if the underlying Either is a Right or else throws the exception provided by
* {@code exceptionFunction} by applying the Left value.
* @throws X if the projected Either is a Left
*/
public R getOrElseThrow(Function super L, X> exceptionFunction) throws X {
Objects.requireNonNull(exceptionFunction, "exceptionFunction is null");
return either.getOrElseThrow(exceptionFunction);
}
/**
* Returns the underlying either of this projection.
*
* @return the underlying either
*/
public Either toEither() {
return either;
}
/**
* Returns {@code Some} value of type R if this is a right projection of a Right value and the predicate
* applies to the underlying value.
*
* @param predicate A predicate
* @return A new Option
*/
public Option> filter(Predicate super R> predicate) {
Objects.requireNonNull(predicate, "predicate is null");
return either.isLeft() || predicate.test(either.get()) ? Option.some(this) : Option.none();
}
/**
* FlatMaps this RightProjection.
*
* @param mapper A mapper
* @param Component type of the mapped right value
* @return this as {@code RightProjection} if a Left is underlying, otherwise a the mapping result of the right value.
* @throws NullPointerException if {@code mapper} is null
*/
@SuppressWarnings("unchecked")
public RightProjection flatMap(Function super R, ? extends RightProjection> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (either.isRight()) {
return (RightProjection) mapper.apply(either.get());
} else {
return (RightProjection) this;
}
}
/**
* Maps the right value if the projected Either is a Right.
*
* @param mapper A mapper which takes a right value and returns a value of type U
* @param The new type of a Right value
* @return A new RightProjection
*/
@SuppressWarnings("unchecked")
@Override
public RightProjection map(Function super R, ? extends U> mapper) {
Objects.requireNonNull(mapper, "mapper is null");
if (either.isRight()) {
return either.map((Function) mapper).right();
} else {
return (RightProjection) this;
}
}
/**
* Applies the given action to the value if the projected either is a Right. Otherwise nothing happens.
*
* @param action An action which takes a right value
* @return this {@code Either} instance
*/
@Override
public RightProjection peek(Consumer super R> action) {
Objects.requireNonNull(action, "action is null");
if (either.isRight()) {
action.accept(either.get());
}
return this;
}
/**
* Transforms this {@code RightProjection}.
*
* @param f A transformation
* @param Type of transformation result
* @return An instance of type {@code U}
* @throws NullPointerException if {@code f} is null
*/
public U transform(Function super RightProjection, ? extends U> f) {
Objects.requireNonNull(f, "f is null");
return f.apply(this);
}
@Override
public Iterator iterator() {
return either.iterator();
}
@Override
public boolean equals(Object obj) {
return (obj == this) || (obj instanceof RightProjection && Objects.equals(either, ((RightProjection, ?>) obj).either));
}
@Override
public int hashCode() {
return either.hashCode();
}
@Override
public String stringPrefix() {
return "RightProjection";
}
@Override
public String toString() {
return stringPrefix() + "(" + either + ")";
}
}
/**
* The {@code Left} version of an {@code Either}.
*
* @param left component type
* @param right component type
* @author Daniel Dietrich
* @since 1.0.0
*/
final class Left implements Either, Serializable {
private static final long serialVersionUID = 1L;
private final L value;
/**
* Constructs a {@code Left}.
*
* @param value a left value
*/
private Left(L value) {
this.value = value;
}
@Override
public R get() {
throw new NoSuchElementException("get() on Left");
}
@Override
public L getLeft() {
return value;
}
@Override
public boolean isLeft() {
return true;
}
@Override
public boolean isRight() {
return false;
}
@Override
public boolean equals(Object obj) {
return (obj == this) || (obj instanceof Left && Objects.equals(value, ((Left, ?>) obj).value));
}
@Override
public int hashCode() {
return Objects.hashCode(value);
}
@Override
public String stringPrefix() {
return "Left";
}
@Override
public String toString() {
return stringPrefix() + "(" + value + ")";
}
}
/**
* The {@code Right} version of an {@code Either}.
*
* @param left component type
* @param right component type
* @author Daniel Dietrich
* @since 1.0.0
*/
final class Right implements Either, Serializable {
private static final long serialVersionUID = 1L;
private final R value;
/**
* Constructs a {@code Right}.
*
* @param value a right value
*/
private Right(R value) {
this.value = value;
}
@Override
public R get() {
return value;
}
@Override
public L getLeft() {
throw new NoSuchElementException("getLeft() on Right");
}
@Override
public boolean isLeft() {
return false;
}
@Override
public boolean isRight() {
return true;
}
@Override
public boolean equals(Object obj) {
return (obj == this) || (obj instanceof Right && Objects.equals(value, ((Right, ?>) obj).value));
}
@Override
public int hashCode() {
return Objects.hashCode(value);
}
@Override
public String stringPrefix() {
return "Right";
}
@Override
public String toString() {
return stringPrefix() + "(" + value + ")";
}
}
}