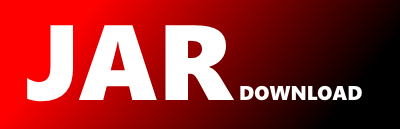
javaslang.$ Maven / Gradle / Ivy
/* / \____ _ _ ____ ______ / \ ____ __ _______
* / / \/ \ / \/ \ / /\__\/ // \/ \ // /\__\ JΛVΛSLΛNG
* _/ / /\ \ \/ / /\ \\__\\ \ // /\ \ /\\/ \ /__\ \ Copyright 2014-2016 Javaslang, http://javaslang.io
* /___/\_/ \_/\____/\_/ \_/\__\/__/\__\_/ \_// \__/\_____/ Licensed under the Apache License, Version 2.0
*/
package javaslang;
import javaslang.collection.List;
import javaslang.collection.Stream;
import javaslang.concurrent.Future;
import javaslang.control.Either;
import javaslang.control.Option;
import javaslang.control.Try;
import javaslang.control.Validation;
import javaslang.match.annotation.Unapply;
import javaslang.match.annotation.Patterns;
@Patterns class $ {
// -- javaslang
// Tuple0-N
@Unapply static Tuple0 Tuple0(Tuple0 tuple0) { return tuple0; }
@Unapply static Tuple1 Tuple1(Tuple1 tuple1) { return tuple1; }
@Unapply static Tuple2 Tuple2(Tuple2 tuple2) { return tuple2; }
@Unapply static Tuple3 Tuple3(Tuple3 tuple3) { return tuple3; }
@Unapply static Tuple4 Tuple4(Tuple4 tuple4) { return tuple4; }
@Unapply static Tuple5 Tuple5(Tuple5 tuple5) { return tuple5; }
@Unapply static Tuple6 Tuple6(Tuple6 tuple6) { return tuple6; }
@Unapply static Tuple7 Tuple7(Tuple7 tuple7) { return tuple7; }
@Unapply static Tuple8 Tuple8(Tuple8 tuple8) { return tuple8; }
// -- javaslang.collection
// List
@Unapply static Tuple2> List(List.Cons cons) { return Tuple.of(cons.head(), cons.tail()); }
@Unapply static Tuple0 List(List.Nil nil) { return Tuple.empty(); }
// Stream
@Unapply static Tuple2> Stream(Stream.Cons cons) { return Tuple.of(cons.head(), cons.tail()); }
@Unapply static Tuple0 Stream(Stream.Empty empty) { return Tuple.empty(); }
// TODO: Tree
// -- javaslang.concurrent
@Unapply static Tuple1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy