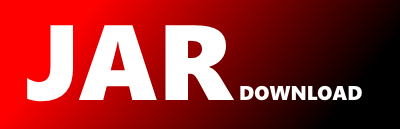
javaslang.Predicates Maven / Gradle / Ivy
/* / \____ _ _ ____ ______ / \ ____ __ _______
* / / \/ \ / \/ \ / /\__\/ // \/ \ // /\__\ JΛVΛSLΛNG
* _/ / /\ \ \/ / /\ \\__\\ \ // /\ \ /\\/ \ /__\ \ Copyright 2014-2016 Javaslang, http://javaslang.io
* /___/\_/ \_/\____/\_/ \_/\__\/__/\__\_/ \_// \__/\_____/ Licensed under the Apache License, Version 2.0
*/
package javaslang;
import javaslang.collection.List;
import java.util.Objects;
import java.util.function.Predicate;
/**
* Defines general-purpose predicates which are particularly useful when working with
* {@link javaslang.API.Match}.
*
* @author Daniel Dietrich, Grzegorz Piwowarek
* @since 2.0.0
*/
public final class Predicates {
// hidden
private Predicates() {
}
/**
* Creates a {@code Predicate} that tests, if an object is instance of the specified {@code type}.
*
* @param type A type
* @param Type of the given {@code type}
* @return A new {@code Predicate}
* @throws NullPointerException if {@code type} is null
*/
// DEV-NOTE: We need Class extends T> instead of Class, see {@link TryTest#shouldRecoverSuccessUsingCase()}
public static Predicate instanceOf(Class extends T> type) {
Objects.requireNonNull(type, "type is null");
return obj -> obj != null && type.isAssignableFrom(obj.getClass());
}
/**
* Creates a {@code Predicate} that tests, if an object is equal to the specified {@code value} using
* {@link Objects#equals(Object, Object)} for comparison.
*
* Hint: Use {@code is(null)} instead of introducing a new predicate {@code isNull()}
*
* @param value A value, may be null
* @param value type
* @return A new {@code Predicate}
*/
public static Predicate is(T value) {
return obj -> Objects.equals(obj, value);
}
/**
* Creates a {@code Predicate} that tests, if an object is equal to at least one of the specified {@code values}
* using {@link Objects#equals(Object, Object)} for comparison.
*
* @param values an array of values of type T
* @param value type
* @return A new {@code Predicate}
* @throws NullPointerException if {@code values} is null
*/
// JDK fails here without "unchecked", Eclipse complains that it is unnecessary
@SuppressWarnings({ "unchecked", "varargs" })
@SafeVarargs
public static Predicate isIn(T... values) {
Objects.requireNonNull(values, "values is null");
return obj -> List.of(values).find(value -> Objects.equals(value, obj)).isDefined();
}
// -- Predicate combinators
/**
* A combinator that checks if all of the given {@code predicates} are satisfied.
*
* By definition {@code allOf} is satisfied if the given {@code predicates} are empty.
*
* @param predicates An array of predicates
* @param clojure over tested object types
* @return A new {@code Predicate}
*/
// JDK fails here without "unchecked", Eclipse complains that it is unnecessary
@SuppressWarnings({ "unchecked", "varargs" })
@SafeVarargs
public static Predicate allOf(Predicate super T>... predicates) {
Objects.requireNonNull(predicates, "predicates is null");
return t -> List.of(predicates).foldLeft(true, (bool, pred) -> bool && pred.test(t));
}
/**
* A combinator that checks if at least one of the given {@code predicates} is satisfies.
*
* @param predicates An array of predicates
* @param clojure over tested object types
* @return A new {@code Predicate}
*/
// JDK fails here without "unchecked", Eclipse complains that it is unnecessary
@SuppressWarnings({ "unchecked", "varargs" })
@SafeVarargs
public static Predicate anyOf(Predicate super T>... predicates) {
Objects.requireNonNull(predicates, "predicates is null");
return t -> List.of(predicates).find(pred -> pred.test(t)).isDefined();
}
/**
* A combinator that checks if none of the given {@code predicates} is satisfied.
*
* Naturally {@code noneOf} is satisfied if the given {@code predicates} are empty.
*
* @param predicates An array of predicates
* @param clojure over tested object types
* @return A new {@code Predicate}
*/
// JDK fails here without "unchecked", Eclipse complains that it is unnecessary
@SuppressWarnings({ "unchecked", "varargs" })
@SafeVarargs
public static Predicate noneOf(Predicate super T>... predicates) {
Objects.requireNonNull(predicates, "predicates is null");
return anyOf(predicates).negate();
}
}