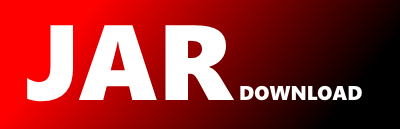
io.gsonfire.util.SimpleIterable Maven / Gradle / Ivy
package io.gsonfire.util;
import java.util.*;
/**
* Created by julio on 6/23/15.
*/
public final class SimpleIterable implements Iterable{
private final Iterable iterable;
private SimpleIterable(Iterable iterable) {
this.iterable = iterable;
}
@Override
public Iterator iterator() {
return iterable.iterator();
}
/**
* Copies the iterable into a new collection
* @return A new collection with the full content of the iterable
*/
public final Collection toCollection() {
List list = new ArrayList();
addTo(list);
return list;
}
/**
* Adds all the elements from the iterable to a collection
* @param collection
* @return
*/
private final void addTo(Collection collection) {
for(T v: this) {
collection.add(v);
}
}
/**
* Creates a {@link SimpleIterable} that iterates through the contents of the iterable passed as argument
* @param iterable
* @param
* @return
*/
public static SimpleIterable of(Iterable iterable) {
if(iterable == null) {
throw new NullPointerException("The iterable parameter cannot be null");
} else {
return new SimpleIterable(iterable);
}
}
/**
* Creates a {@link SimpleIterable} that iterates through the contents of the array passed as argument
* @param array
* @param
* @return
*/
public static SimpleIterable of(T... array) {
return of(Arrays.asList(array));
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SimpleIterable> that = (SimpleIterable>) o;
return !(iterable != null ? !iterable.equals(that.iterable) : that.iterable != null);
}
@Override
public int hashCode() {
return iterable != null ? iterable.hashCode() : 0;
}
@Override
public String toString() {
return iterable.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy