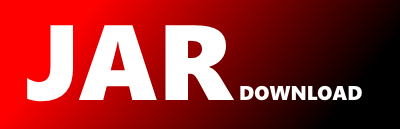
io.swagger.client.api.AccountUpdaterApi Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.api;
import qpPlatform.ApiCallback;
import qpPlatform.ApiClient;
import qpPlatform.ApiException;
import qpPlatform.ApiResponse;
import qpPlatform.Configuration;
import qpPlatform.Pair;
import qpPlatform.ProgressRequestBody;
import qpPlatform.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.swagger.client.model.AusHoldRequest;
import io.swagger.client.model.AusReleaseRequest;
import io.swagger.client.model.AusRequest;
import io.swagger.client.model.AusRequestResponse;
import io.swagger.client.model.AusUpdatedResponse;
import java.io.File;
import io.swagger.client.model.QPApiResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AccountUpdaterApi {
private ApiClient apiClient;
public AccountUpdaterApi() {
this(Configuration.getDefaultApiClient());
}
public AccountUpdaterApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for addAusFileRequest
* @param file The file to upload. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addAusFileRequestCall(File file, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/aus/add/file";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (file != null)
localVarFormParams.put("file", file);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"multipart/form-data"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addAusFileRequestValidateBeforeCall(File file, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'file' is set
if (file == null) {
throw new ApiException("Missing the required parameter 'file' when calling addAusFileRequest(Async)");
}
com.squareup.okhttp.Call call = addAusFileRequestCall(file, progressListener, progressRequestListener);
return call;
}
/**
* Submit AUS CSV File Request
* Submit an Account Updater request using full card number and expiration date using csv file
* @param file The file to upload. (required)
* @return AusRequestResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AusRequestResponse addAusFileRequest(File file) throws ApiException {
ApiResponse resp = addAusFileRequestWithHttpInfo(file);
return resp.getData();
}
/**
* Submit AUS CSV File Request
* Submit an Account Updater request using full card number and expiration date using csv file
* @param file The file to upload. (required)
* @return ApiResponse<AusRequestResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addAusFileRequestWithHttpInfo(File file) throws ApiException {
com.squareup.okhttp.Call call = addAusFileRequestValidateBeforeCall(file, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Submit AUS CSV File Request (asynchronously)
* Submit an Account Updater request using full card number and expiration date using csv file
* @param file The file to upload. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addAusFileRequestAsync(File file, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addAusFileRequestValidateBeforeCall(file, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for addAusJsonRequest
* @param body aus request (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addAusJsonRequestCall(AusRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/aus/add";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addAusJsonRequestValidateBeforeCall(AusRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling addAusJsonRequest(Async)");
}
com.squareup.okhttp.Call call = addAusJsonRequestCall(body, progressListener, progressRequestListener);
return call;
}
/**
* Submit AUS Request
* Submit an Account Updater request using full card number and expiration date.
* @param body aus request (required)
* @return AusRequestResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AusRequestResponse addAusJsonRequest(AusRequest body) throws ApiException {
ApiResponse resp = addAusJsonRequestWithHttpInfo(body);
return resp.getData();
}
/**
* Submit AUS Request
* Submit an Account Updater request using full card number and expiration date.
* @param body aus request (required)
* @return ApiResponse<AusRequestResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addAusJsonRequestWithHttpInfo(AusRequest body) throws ApiException {
com.squareup.okhttp.Call call = addAusJsonRequestValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Submit AUS Request (asynchronously)
* Submit an Account Updater request using full card number and expiration date.
* @param body aus request (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addAusJsonRequestAsync(AusRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addAusJsonRequestValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAusResponse
* @param requestId Request ID (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getAusResponseCall(Long requestId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/aus/{requestId}"
.replaceAll("\\{" + "requestId" + "\\}", apiClient.escapeString(requestId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getAusResponseValidateBeforeCall(Long requestId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'requestId' is set
if (requestId == null) {
throw new ApiException("Missing the required parameter 'requestId' when calling getAusResponse(Async)");
}
com.squareup.okhttp.Call call = getAusResponseCall(requestId, progressListener, progressRequestListener);
return call;
}
/**
* Get AUS Response
* Get updated AUS response using requestId
* @param requestId Request ID (required)
* @return AusUpdatedResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AusUpdatedResponse getAusResponse(Long requestId) throws ApiException {
ApiResponse resp = getAusResponseWithHttpInfo(requestId);
return resp.getData();
}
/**
* Get AUS Response
* Get updated AUS response using requestId
* @param requestId Request ID (required)
* @return ApiResponse<AusUpdatedResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAusResponseWithHttpInfo(Long requestId) throws ApiException {
com.squareup.okhttp.Call call = getAusResponseValidateBeforeCall(requestId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get AUS Response (asynchronously)
* Get updated AUS response using requestId
* @param requestId Request ID (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getAusResponseAsync(Long requestId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getAusResponseValidateBeforeCall(requestId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for putOnAusHold
* @param body AusOnHold (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call putOnAusHoldCall(AusHoldRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/aus/hold";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call putOnAusHoldValidateBeforeCall(AusHoldRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling putOnAusHold(Async)");
}
com.squareup.okhttp.Call call = putOnAusHoldCall(body, progressListener, progressRequestListener);
return call;
}
/**
* Put on Account Updater Harvest Hold
* Put a card id or all payment cards belonging to a customer on Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards. Account updater service cannot process ACH payments or foreign issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusOnHold (required)
* @return QPApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public QPApiResponse putOnAusHold(AusHoldRequest body) throws ApiException {
ApiResponse resp = putOnAusHoldWithHttpInfo(body);
return resp.getData();
}
/**
* Put on Account Updater Harvest Hold
* Put a card id or all payment cards belonging to a customer on Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards. Account updater service cannot process ACH payments or foreign issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusOnHold (required)
* @return ApiResponse<QPApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse putOnAusHoldWithHttpInfo(AusHoldRequest body) throws ApiException {
com.squareup.okhttp.Call call = putOnAusHoldValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Put on Account Updater Harvest Hold (asynchronously)
* Put a card id or all payment cards belonging to a customer on Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards. Account updater service cannot process ACH payments or foreign issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusOnHold (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call putOnAusHoldAsync(AusHoldRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = putOnAusHoldValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for releaseFromAusHold
* @param body AusRelease (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call releaseFromAusHoldCall(AusReleaseRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/aus/release";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call releaseFromAusHoldValidateBeforeCall(AusReleaseRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling releaseFromAusHold(Async)");
}
com.squareup.okhttp.Call call = releaseFromAusHoldCall(body, progressListener, progressRequestListener);
return call;
}
/**
* Release from Account Updater Harvest Hold
* Release a card id or all payment methods belonging to a customer from Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusRelease (required)
* @return QPApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public QPApiResponse releaseFromAusHold(AusReleaseRequest body) throws ApiException {
ApiResponse resp = releaseFromAusHoldWithHttpInfo(body);
return resp.getData();
}
/**
* Release from Account Updater Harvest Hold
* Release a card id or all payment methods belonging to a customer from Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusRelease (required)
* @return ApiResponse<QPApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse releaseFromAusHoldWithHttpInfo(AusReleaseRequest body) throws ApiException {
com.squareup.okhttp.Call call = releaseFromAusHoldValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Release from Account Updater Harvest Hold (asynchronously)
* Release a card id or all payment methods belonging to a customer from Account updater harvest hold. Account updater service cannot process ACH payments or non U.S. issued cards, hence this request is applicable to only U.S. issued credit cards.
* @param body AusRelease (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call releaseFromAusHoldAsync(AusReleaseRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = releaseFromAusHoldValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy