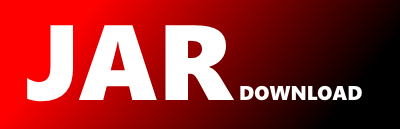
io.swagger.client.api.InvoicingApi Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.api;
import qpPlatform.ApiCallback;
import qpPlatform.ApiClient;
import qpPlatform.ApiException;
import qpPlatform.ApiResponse;
import qpPlatform.Configuration;
import qpPlatform.Pair;
import qpPlatform.ProgressRequestBody;
import qpPlatform.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.swagger.client.model.CopyInvoiceRequest;
import io.swagger.client.model.CreateInvoiceRequest;
import io.swagger.client.model.InvoiceBouncedResponse;
import io.swagger.client.model.InvoiceListResponse;
import io.swagger.client.model.InvoicePaymentListResponse;
import io.swagger.client.model.InvoicePaymentRequest;
import io.swagger.client.model.InvoicePaymentResponse;
import io.swagger.client.model.InvoiceResponse;
import io.swagger.client.model.QPApiResponse;
import io.swagger.client.model.ResendInvoiceRequest;
import io.swagger.client.model.SendInvoiceRequest;
import io.swagger.client.model.UpdateDraftRequest;
import io.swagger.client.model.UpdateOutstandingRequest;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class InvoicingApi {
private ApiClient apiClient;
public InvoicingApi() {
this(Configuration.getDefaultApiClient());
}
public InvoicingApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for addInvoicePayment
* @param invoiceId (required)
* @param body Invoice Payment (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addInvoicePaymentCall(Long invoiceId, InvoicePaymentRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/payments"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addInvoicePaymentValidateBeforeCall(Long invoiceId, InvoicePaymentRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling addInvoicePayment(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling addInvoicePayment(Async)");
}
com.squareup.okhttp.Call call = addInvoicePaymentCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Add Payment to an Invoice
* Adds a payment to an invoice. A check or cash payment can be added to a saved or outstanding invoice. Credit card payments cannot be added manually to an invoice.
* @param invoiceId (required)
* @param body Invoice Payment (required)
* @return InvoicePaymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoicePaymentResponse addInvoicePayment(Long invoiceId, InvoicePaymentRequest body) throws ApiException {
ApiResponse resp = addInvoicePaymentWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Add Payment to an Invoice
* Adds a payment to an invoice. A check or cash payment can be added to a saved or outstanding invoice. Credit card payments cannot be added manually to an invoice.
* @param invoiceId (required)
* @param body Invoice Payment (required)
* @return ApiResponse<InvoicePaymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addInvoicePaymentWithHttpInfo(Long invoiceId, InvoicePaymentRequest body) throws ApiException {
com.squareup.okhttp.Call call = addInvoicePaymentValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add Payment to an Invoice (asynchronously)
* Adds a payment to an invoice. A check or cash payment can be added to a saved or outstanding invoice. Credit card payments cannot be added manually to an invoice.
* @param invoiceId (required)
* @param body Invoice Payment (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addInvoicePaymentAsync(Long invoiceId, InvoicePaymentRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addInvoicePaymentValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseBouncedInvoices
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to db_timestamp)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseBouncedInvoicesCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/bounced";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseBouncedInvoicesValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseBouncedInvoicesCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Get Undelivered Invoices
* Browse all undelivered invoices. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to db_timestamp)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return InvoiceBouncedResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceBouncedResponse browseBouncedInvoices(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseBouncedInvoicesWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Get Undelivered Invoices
* Browse all undelivered invoices. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to db_timestamp)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<InvoiceBouncedResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseBouncedInvoicesWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseBouncedInvoicesValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Undelivered Invoices (asynchronously)
* Browse all undelivered invoices. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to db_timestamp)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseBouncedInvoicesAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseBouncedInvoicesValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseInvoicePayments
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseInvoicePaymentsCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/payments";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseInvoicePaymentsValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseInvoicePaymentsCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Get Invoice Payments
* Browse all invoice payments. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return InvoicePaymentListResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoicePaymentListResponse browseInvoicePayments(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseInvoicePaymentsWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Get Invoice Payments
* Browse all invoice payments. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<InvoicePaymentListResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseInvoicePaymentsWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseInvoicePaymentsValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Invoice Payments (asynchronously)
* Browse all invoice payments. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseInvoicePaymentsAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseInvoicePaymentsValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseInvoicePaymentsById
* @param invoiceId (required)
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseInvoicePaymentsByIdCall(Long invoiceId, Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/payments"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseInvoicePaymentsByIdValidateBeforeCall(Long invoiceId, Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling browseInvoicePaymentsById(Async)");
}
com.squareup.okhttp.Call call = browseInvoicePaymentsByIdCall(invoiceId, count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Get invoice payments by id
* Browse all invoice payments made to an invoice. Optional query parameters determines, size and sort order of returned array.
* @param invoiceId (required)
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return InvoicePaymentListResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoicePaymentListResponse browseInvoicePaymentsById(Long invoiceId, Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseInvoicePaymentsByIdWithHttpInfo(invoiceId, count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Get invoice payments by id
* Browse all invoice payments made to an invoice. Optional query parameters determines, size and sort order of returned array.
* @param invoiceId (required)
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<InvoicePaymentListResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseInvoicePaymentsByIdWithHttpInfo(Long invoiceId, Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseInvoicePaymentsByIdValidateBeforeCall(invoiceId, count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get invoice payments by id (asynchronously)
* Browse all invoice payments made to an invoice. Optional query parameters determines, size and sort order of returned array.
* @param invoiceId (required)
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to date_payment)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseInvoicePaymentsByIdAsync(Long invoiceId, Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseInvoicePaymentsByIdValidateBeforeCall(invoiceId, count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseInvoices
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to invoice_number)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseInvoicesCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseInvoicesValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseInvoicesCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Get all Invoices
* Gets an array of invoice objects. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to invoice_number)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return InvoiceListResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceListResponse browseInvoices(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseInvoicesWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Get all Invoices
* Gets an array of invoice objects. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to invoice_number)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<InvoiceListResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseInvoicesWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseInvoicesValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all Invoices (asynchronously)
* Gets an array of invoice objects. Optional query parameters determines, size and sort order of returned array.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to invoice_number)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseInvoicesAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseInvoicesValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for cancelInvoice
* @param invoiceId (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call cancelInvoiceCall(Long invoiceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/cancel"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call cancelInvoiceValidateBeforeCall(Long invoiceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling cancelInvoice(Async)");
}
com.squareup.okhttp.Call call = cancelInvoiceCall(invoiceId, progressListener, progressRequestListener);
return call;
}
/**
* Cancel an Invoice
* Cancels an invoice. A canceled invoice cannot be edited. If your customer clicks on the pay now button in the invoice e-mail an error message will be displayed.
* @param invoiceId (required)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse cancelInvoice(Long invoiceId) throws ApiException {
ApiResponse resp = cancelInvoiceWithHttpInfo(invoiceId);
return resp.getData();
}
/**
* Cancel an Invoice
* Cancels an invoice. A canceled invoice cannot be edited. If your customer clicks on the pay now button in the invoice e-mail an error message will be displayed.
* @param invoiceId (required)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse cancelInvoiceWithHttpInfo(Long invoiceId) throws ApiException {
com.squareup.okhttp.Call call = cancelInvoiceValidateBeforeCall(invoiceId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Cancel an Invoice (asynchronously)
* Cancels an invoice. A canceled invoice cannot be edited. If your customer clicks on the pay now button in the invoice e-mail an error message will be displayed.
* @param invoiceId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call cancelInvoiceAsync(Long invoiceId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = cancelInvoiceValidateBeforeCall(invoiceId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for copyInvoice
* @param invoiceId (required)
* @param body Invoice (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call copyInvoiceCall(Long invoiceId, CopyInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/copy"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call copyInvoiceValidateBeforeCall(Long invoiceId, CopyInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling copyInvoice(Async)");
}
com.squareup.okhttp.Call call = copyInvoiceCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Copy an Invoice
* Makes a copy of an invoice. The invoice date will be set to today's date and due date will be adjusted based on the invoice date and the payment terms. Optionally, include an invoice_number in the POST body to make a copy of the invoice with a different invoice number. Invoice payments from the original invoice will not be copied.
* @param invoiceId (required)
* @param body Invoice (optional)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse copyInvoice(Long invoiceId, CopyInvoiceRequest body) throws ApiException {
ApiResponse resp = copyInvoiceWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Copy an Invoice
* Makes a copy of an invoice. The invoice date will be set to today's date and due date will be adjusted based on the invoice date and the payment terms. Optionally, include an invoice_number in the POST body to make a copy of the invoice with a different invoice number. Invoice payments from the original invoice will not be copied.
* @param invoiceId (required)
* @param body Invoice (optional)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse copyInvoiceWithHttpInfo(Long invoiceId, CopyInvoiceRequest body) throws ApiException {
com.squareup.okhttp.Call call = copyInvoiceValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Copy an Invoice (asynchronously)
* Makes a copy of an invoice. The invoice date will be set to today's date and due date will be adjusted based on the invoice date and the payment terms. Optionally, include an invoice_number in the POST body to make a copy of the invoice with a different invoice number. Invoice payments from the original invoice will not be copied.
* @param invoiceId (required)
* @param body Invoice (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call copyInvoiceAsync(Long invoiceId, CopyInvoiceRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = copyInvoiceValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createInvoice
* @param body Invoice (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call createInvoiceCall(CreateInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call createInvoiceValidateBeforeCall(CreateInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling createInvoice(Async)");
}
com.squareup.okhttp.Call call = createInvoiceCall(body, progressListener, progressRequestListener);
return call;
}
/**
* Create an invoice
* Creates a draft invoice that you can send later using the Send Invoice API.
* @param body Invoice (required)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse createInvoice(CreateInvoiceRequest body) throws ApiException {
ApiResponse resp = createInvoiceWithHttpInfo(body);
return resp.getData();
}
/**
* Create an invoice
* Creates a draft invoice that you can send later using the Send Invoice API.
* @param body Invoice (required)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createInvoiceWithHttpInfo(CreateInvoiceRequest body) throws ApiException {
com.squareup.okhttp.Call call = createInvoiceValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create an invoice (asynchronously)
* Creates a draft invoice that you can send later using the Send Invoice API.
* @param body Invoice (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call createInvoiceAsync(CreateInvoiceRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = createInvoiceValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getInvoice
* @param invoiceId Invoice ID (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getInvoiceCall(Long invoiceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/detail"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getInvoiceValidateBeforeCall(Long invoiceId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling getInvoice(Async)");
}
com.squareup.okhttp.Call call = getInvoiceCall(invoiceId, progressListener, progressRequestListener);
return call;
}
/**
* Get by Invoice ID
* Gets an invoice by invoice_id.
* @param invoiceId Invoice ID (required)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse getInvoice(Long invoiceId) throws ApiException {
ApiResponse resp = getInvoiceWithHttpInfo(invoiceId);
return resp.getData();
}
/**
* Get by Invoice ID
* Gets an invoice by invoice_id.
* @param invoiceId Invoice ID (required)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getInvoiceWithHttpInfo(Long invoiceId) throws ApiException {
com.squareup.okhttp.Call call = getInvoiceValidateBeforeCall(invoiceId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get by Invoice ID (asynchronously)
* Gets an invoice by invoice_id.
* @param invoiceId Invoice ID (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getInvoiceAsync(Long invoiceId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getInvoiceValidateBeforeCall(invoiceId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for removeInvoicePayment
* @param invoiceId (required)
* @param paymentId (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call removeInvoicePaymentCall(Long invoiceId, Long paymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/payments/{payment_id}"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()))
.replaceAll("\\{" + "payment_id" + "\\}", apiClient.escapeString(paymentId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call removeInvoicePaymentValidateBeforeCall(Long invoiceId, Long paymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling removeInvoicePayment(Async)");
}
// verify the required parameter 'paymentId' is set
if (paymentId == null) {
throw new ApiException("Missing the required parameter 'paymentId' when calling removeInvoicePayment(Async)");
}
com.squareup.okhttp.Call call = removeInvoicePaymentCall(invoiceId, paymentId, progressListener, progressRequestListener);
return call;
}
/**
* Remove an Invoice Payment
* Removes an invoice payment. A payment can be removed on a saved or an outstanding invoice. CARD type payments cannot be removed. Payments made via a credit card cannot be removed. Payments can be deleted only from SAVED or OUTSTANDING invoices.
* @param invoiceId (required)
* @param paymentId (required)
* @return QPApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public QPApiResponse removeInvoicePayment(Long invoiceId, Long paymentId) throws ApiException {
ApiResponse resp = removeInvoicePaymentWithHttpInfo(invoiceId, paymentId);
return resp.getData();
}
/**
* Remove an Invoice Payment
* Removes an invoice payment. A payment can be removed on a saved or an outstanding invoice. CARD type payments cannot be removed. Payments made via a credit card cannot be removed. Payments can be deleted only from SAVED or OUTSTANDING invoices.
* @param invoiceId (required)
* @param paymentId (required)
* @return ApiResponse<QPApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse removeInvoicePaymentWithHttpInfo(Long invoiceId, Long paymentId) throws ApiException {
com.squareup.okhttp.Call call = removeInvoicePaymentValidateBeforeCall(invoiceId, paymentId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove an Invoice Payment (asynchronously)
* Removes an invoice payment. A payment can be removed on a saved or an outstanding invoice. CARD type payments cannot be removed. Payments made via a credit card cannot be removed. Payments can be deleted only from SAVED or OUTSTANDING invoices.
* @param invoiceId (required)
* @param paymentId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call removeInvoicePaymentAsync(Long invoiceId, Long paymentId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = removeInvoicePaymentValidateBeforeCall(invoiceId, paymentId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for resendInvoice
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call resendInvoiceCall(Long invoiceId, ResendInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/resend"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call resendInvoiceValidateBeforeCall(Long invoiceId, ResendInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling resendInvoice(Async)");
}
com.squareup.okhttp.Call call = resendInvoiceCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Resend an Invoice
* Resends an invoice to the customer. An outstanding or paid invoice can be resent.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @return QPApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public QPApiResponse resendInvoice(Long invoiceId, ResendInvoiceRequest body) throws ApiException {
ApiResponse resp = resendInvoiceWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Resend an Invoice
* Resends an invoice to the customer. An outstanding or paid invoice can be resent.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @return ApiResponse<QPApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse resendInvoiceWithHttpInfo(Long invoiceId, ResendInvoiceRequest body) throws ApiException {
com.squareup.okhttp.Call call = resendInvoiceValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Resend an Invoice (asynchronously)
* Resends an invoice to the customer. An outstanding or paid invoice can be resent.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call resendInvoiceAsync(Long invoiceId, ResendInvoiceRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = resendInvoiceValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for sendInvoice
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call sendInvoiceCall(Long invoiceId, SendInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/send"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call sendInvoiceValidateBeforeCall(Long invoiceId, SendInvoiceRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling sendInvoice(Async)");
}
com.squareup.okhttp.Call call = sendInvoiceCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Send an Invoice
* Sends an invoice to the customer. Sending an invoice changes the status of the invoice to outstanding. Once sent, only the from_contact and business_contact of the invoice can be updated.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse sendInvoice(Long invoiceId, SendInvoiceRequest body) throws ApiException {
ApiResponse resp = sendInvoiceWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Send an Invoice
* Sends an invoice to the customer. Sending an invoice changes the status of the invoice to outstanding. Once sent, only the from_contact and business_contact of the invoice can be updated.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendInvoiceWithHttpInfo(Long invoiceId, SendInvoiceRequest body) throws ApiException {
com.squareup.okhttp.Call call = sendInvoiceValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Send an Invoice (asynchronously)
* Sends an invoice to the customer. Sending an invoice changes the status of the invoice to outstanding. Once sent, only the from_contact and business_contact of the invoice can be updated.
* @param invoiceId (required)
* @param body Email Addresses (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call sendInvoiceAsync(Long invoiceId, SendInvoiceRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = sendInvoiceValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateDraftInvoice
* @param invoiceId (required)
* @param body Invoice (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateDraftInvoiceCall(Long invoiceId, UpdateDraftRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/draft"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateDraftInvoiceValidateBeforeCall(Long invoiceId, UpdateDraftRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling updateDraftInvoice(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling updateDraftInvoice(Async)");
}
com.squareup.okhttp.Call call = updateDraftInvoiceCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Update a Draft Invoice
* Updates a draft invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse updateDraftInvoice(Long invoiceId, UpdateDraftRequest body) throws ApiException {
ApiResponse resp = updateDraftInvoiceWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Update a Draft Invoice
* Updates a draft invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateDraftInvoiceWithHttpInfo(Long invoiceId, UpdateDraftRequest body) throws ApiException {
com.squareup.okhttp.Call call = updateDraftInvoiceValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update a Draft Invoice (asynchronously)
* Updates a draft invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateDraftInvoiceAsync(Long invoiceId, UpdateDraftRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateDraftInvoiceValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateInvoicePayment
* @param invoiceId (required)
* @param paymentId (required)
* @param body Invoice Payment (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateInvoicePaymentCall(Long invoiceId, Long paymentId, InvoicePaymentRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/payments/{payment_id}"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()))
.replaceAll("\\{" + "payment_id" + "\\}", apiClient.escapeString(paymentId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateInvoicePaymentValidateBeforeCall(Long invoiceId, Long paymentId, InvoicePaymentRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling updateInvoicePayment(Async)");
}
// verify the required parameter 'paymentId' is set
if (paymentId == null) {
throw new ApiException("Missing the required parameter 'paymentId' when calling updateInvoicePayment(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling updateInvoicePayment(Async)");
}
com.squareup.okhttp.Call call = updateInvoicePaymentCall(invoiceId, paymentId, body, progressListener, progressRequestListener);
return call;
}
/**
* Update an Invoice Payment
* Updates an invoice payment. A payment can be updated on a saved or an outstanding invoice. Payments made via credit card using the “Pay Now” button cannot be updated.
* @param invoiceId (required)
* @param paymentId (required)
* @param body Invoice Payment (required)
* @return InvoicePaymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoicePaymentResponse updateInvoicePayment(Long invoiceId, Long paymentId, InvoicePaymentRequest body) throws ApiException {
ApiResponse resp = updateInvoicePaymentWithHttpInfo(invoiceId, paymentId, body);
return resp.getData();
}
/**
* Update an Invoice Payment
* Updates an invoice payment. A payment can be updated on a saved or an outstanding invoice. Payments made via credit card using the “Pay Now” button cannot be updated.
* @param invoiceId (required)
* @param paymentId (required)
* @param body Invoice Payment (required)
* @return ApiResponse<InvoicePaymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateInvoicePaymentWithHttpInfo(Long invoiceId, Long paymentId, InvoicePaymentRequest body) throws ApiException {
com.squareup.okhttp.Call call = updateInvoicePaymentValidateBeforeCall(invoiceId, paymentId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update an Invoice Payment (asynchronously)
* Updates an invoice payment. A payment can be updated on a saved or an outstanding invoice. Payments made via credit card using the “Pay Now” button cannot be updated.
* @param invoiceId (required)
* @param paymentId (required)
* @param body Invoice Payment (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateInvoicePaymentAsync(Long invoiceId, Long paymentId, InvoicePaymentRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateInvoicePaymentValidateBeforeCall(invoiceId, paymentId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateOutstandingInvoice
* @param invoiceId (required)
* @param body Invoice (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateOutstandingInvoiceCall(Long invoiceId, UpdateOutstandingRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/invoice/{invoice_id}/outstanding"
.replaceAll("\\{" + "invoice_id" + "\\}", apiClient.escapeString(invoiceId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateOutstandingInvoiceValidateBeforeCall(Long invoiceId, UpdateOutstandingRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'invoiceId' is set
if (invoiceId == null) {
throw new ApiException("Missing the required parameter 'invoiceId' when calling updateOutstandingInvoice(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling updateOutstandingInvoice(Async)");
}
com.squareup.okhttp.Call call = updateOutstandingInvoiceCall(invoiceId, body, progressListener, progressRequestListener);
return call;
}
/**
* Update an Outstanding Invoice
* Updates an outstanding invoice. Only the from_contact and business_contact fields can be updated on an outstanding invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @return InvoiceResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InvoiceResponse updateOutstandingInvoice(Long invoiceId, UpdateOutstandingRequest body) throws ApiException {
ApiResponse resp = updateOutstandingInvoiceWithHttpInfo(invoiceId, body);
return resp.getData();
}
/**
* Update an Outstanding Invoice
* Updates an outstanding invoice. Only the from_contact and business_contact fields can be updated on an outstanding invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @return ApiResponse<InvoiceResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateOutstandingInvoiceWithHttpInfo(Long invoiceId, UpdateOutstandingRequest body) throws ApiException {
com.squareup.okhttp.Call call = updateOutstandingInvoiceValidateBeforeCall(invoiceId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update an Outstanding Invoice (asynchronously)
* Updates an outstanding invoice. Only the from_contact and business_contact fields can be updated on an outstanding invoice. Only the fields that need updating can be sent in the request body. If updating JSON object fields, the complete JSON should be sent in the request body.
* @param invoiceId (required)
* @param body Invoice (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateOutstandingInvoiceAsync(Long invoiceId, UpdateOutstandingRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateOutstandingInvoiceValidateBeforeCall(invoiceId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy