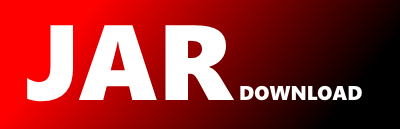
io.swagger.client.api.ReportingApi Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.api;
import qpPlatform.ApiCallback;
import qpPlatform.ApiClient;
import qpPlatform.ApiException;
import qpPlatform.ApiResponse;
import qpPlatform.Configuration;
import qpPlatform.Pair;
import qpPlatform.ProgressRequestBody;
import qpPlatform.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.swagger.client.model.AccountUpdaterResponse;
import io.swagger.client.model.AccountUpdaterSummaryResponse;
import io.swagger.client.model.BatchResponse;
import io.swagger.client.model.DepositResponse;
import io.swagger.client.model.DisputeResponse;
import io.swagger.client.model.PGTransactionResponse;
import io.swagger.client.model.QPApiResponse;
import io.swagger.client.model.SettledTransactionResponse;
import io.swagger.client.model.StatementDisputeResponse;
import io.swagger.client.model.StatementFeeResponse;
import io.swagger.client.model.StatementPlanResponse;
import io.swagger.client.model.StatementReserveResponse;
import io.swagger.client.model.StatementSettlementResponse;
import io.swagger.client.model.TransactionRequestResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ReportingApi {
private ApiClient apiClient;
public ReportingApi() {
this(Configuration.getDefaultApiClient());
}
public ReportingApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for browseAusRequests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseAusRequestsCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/aus/detail";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseAusRequestsValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseAusRequestsCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Account Updater Detail Report
* Browses a paginated list of Account updater requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return AccountUpdaterResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AccountUpdaterResponse browseAusRequests(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseAusRequestsWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Account Updater Detail Report
* Browses a paginated list of Account updater requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<AccountUpdaterResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseAusRequestsWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseAusRequestsValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Account Updater Detail Report (asynchronously)
* Browses a paginated list of Account updater requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseAusRequestsAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseAusRequestsValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseAusSummary
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseAusSummaryCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/aus/summary";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseAusSummaryValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseAusSummaryCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Account Updater Summary Report
* Browses a paginated list of account updater summary report
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return AccountUpdaterSummaryResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AccountUpdaterSummaryResponse browseAusSummary(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseAusSummaryWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Account Updater Summary Report
* Browses a paginated list of account updater summary report
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<AccountUpdaterSummaryResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseAusSummaryWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseAusSummaryValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Account Updater Summary Report (asynchronously)
* Browses a paginated list of account updater summary report
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseAusSummaryAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseAusSummaryValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseBatches
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseBatchesCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/batches";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseBatchesValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseBatchesCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Batch Report
* Browses a paginated list of merchant batches. These are whole batches which have been settled from the merchant's POS device, software, or gateway.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return BatchResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public BatchResponse browseBatches(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseBatchesWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Batch Report
* Browses a paginated list of merchant batches. These are whole batches which have been settled from the merchant's POS device, software, or gateway.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<BatchResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseBatchesWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseBatchesValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Batch Report (asynchronously)
* Browses a paginated list of merchant batches. These are whole batches which have been settled from the merchant's POS device, software, or gateway.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseBatchesAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseBatchesValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseDeposits
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseDepositsCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/deposits";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseDepositsValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseDepositsCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Deposit Report
* Browses a paginated list of bank deposits and withdrawls.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return DepositResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DepositResponse browseDeposits(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseDepositsWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Deposit Report
* Browses a paginated list of bank deposits and withdrawls.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<DepositResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseDepositsWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseDepositsValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Deposit Report (asynchronously)
* Browses a paginated list of bank deposits and withdrawls.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseDepositsAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseDepositsValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseDisputes
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseDisputesCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/disputes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseDisputesValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseDisputesCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Disputes Report
* Browses a paginated list of disputes.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return DisputeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DisputeResponse browseDisputes(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseDisputesWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Disputes Report
* Browses a paginated list of disputes.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<DisputeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseDisputesWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseDisputesValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Disputes Report (asynchronously)
* Browses a paginated list of disputes.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseDisputesAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseDisputesValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseStatementDisputeAdjustmentData
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseStatementDisputeAdjustmentDataCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/statement-report/disputeAdjustment";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseStatementDisputeAdjustmentDataValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseStatementDisputeAdjustmentDataCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Statement Dispute Adjustments Data
* Browses a paginated list of Statement Disputes Adjustment Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return StatementDisputeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public StatementDisputeResponse browseStatementDisputeAdjustmentData(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseStatementDisputeAdjustmentDataWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Statement Dispute Adjustments Data
* Browses a paginated list of Statement Disputes Adjustment Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<StatementDisputeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseStatementDisputeAdjustmentDataWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseStatementDisputeAdjustmentDataValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Statement Dispute Adjustments Data (asynchronously)
* Browses a paginated list of Statement Disputes Adjustment Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseStatementDisputeAdjustmentDataAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseStatementDisputeAdjustmentDataValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseStatementFeeData
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseStatementFeeDataCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/statement-report/fees";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseStatementFeeDataValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseStatementFeeDataCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Statement Fee Data
* Browses a paginated list of Statement Fee Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return StatementFeeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public StatementFeeResponse browseStatementFeeData(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseStatementFeeDataWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Statement Fee Data
* Browses a paginated list of Statement Fee Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<StatementFeeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseStatementFeeDataWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseStatementFeeDataValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Statement Fee Data (asynchronously)
* Browses a paginated list of Statement Fee Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseStatementFeeDataAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseStatementFeeDataValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseStatementPlanData
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseStatementPlanDataCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/statement-report/planType";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseStatementPlanDataValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseStatementPlanDataCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Statement Plan Type Data
* Browses a paginated list of Statement Plan Type Summary
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return StatementPlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public StatementPlanResponse browseStatementPlanData(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseStatementPlanDataWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Statement Plan Type Data
* Browses a paginated list of Statement Plan Type Summary
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<StatementPlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseStatementPlanDataWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseStatementPlanDataValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Statement Plan Type Data (asynchronously)
* Browses a paginated list of Statement Plan Type Summary
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseStatementPlanDataAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseStatementPlanDataValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseStatementReserveData
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseStatementReserveDataCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/statement-report/reserve";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseStatementReserveDataValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseStatementReserveDataCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Statement Reserve Data
* Browses a paginated list of Statement Reserve Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return StatementReserveResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public StatementReserveResponse browseStatementReserveData(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseStatementReserveDataWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Statement Reserve Data
* Browses a paginated list of Statement Reserve Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<StatementReserveResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseStatementReserveDataWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseStatementReserveDataValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Statement Reserve Data (asynchronously)
* Browses a paginated list of Statement Reserve Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseStatementReserveDataAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseStatementReserveDataValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseStatementSettlemetData
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseStatementSettlemetDataCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/statement-report/settlement";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseStatementSettlemetDataValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseStatementSettlemetDataCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Statement Settlemet Data
* Browses a paginated list of Statement Settlemet Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return StatementSettlementResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public StatementSettlementResponse browseStatementSettlemetData(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseStatementSettlemetDataWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Statement Settlemet Data
* Browses a paginated list of Statement Settlemet Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<StatementSettlementResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseStatementSettlemetDataWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseStatementSettlemetDataValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Statement Settlemet Data (asynchronously)
* Browses a paginated list of Statement Settlemet Data
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseStatementSettlemetDataAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseStatementSettlemetDataValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for browseTrans
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call browseTransCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/transactions";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call browseTransValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = browseTransCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Settled Transaction Report
* Browses a paginated list of settled transactions. Some additional text about deposits and stuff.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return SettledTransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SettledTransactionResponse browseTrans(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = browseTransWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Settled Transaction Report
* Browses a paginated list of settled transactions. Some additional text about deposits and stuff.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<SettledTransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse browseTransWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = browseTransValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Settled Transaction Report (asynchronously)
* Browses a paginated list of settled transactions. Some additional text about deposits and stuff.
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call browseTransAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = browseTransValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTransactionByPgId
* @param pgId PG ID (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getTransactionByPgIdCall(String pgId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/transactions/bypgid/{pg_id}"
.replaceAll("\\{" + "pg_id" + "\\}", apiClient.escapeString(pgId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getTransactionByPgIdValidateBeforeCall(String pgId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'pgId' is set
if (pgId == null) {
throw new ApiException("Missing the required parameter 'pgId' when calling getTransactionByPgId(Async)");
}
com.squareup.okhttp.Call call = getTransactionByPgIdCall(pgId, progressListener, progressRequestListener);
return call;
}
/**
* Get transaction by PG ID
* Gets a payment gateway transaction by Payment Gateway ID.
* @param pgId PG ID (required)
* @return PGTransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public PGTransactionResponse getTransactionByPgId(String pgId) throws ApiException {
ApiResponse resp = getTransactionByPgIdWithHttpInfo(pgId);
return resp.getData();
}
/**
* Get transaction by PG ID
* Gets a payment gateway transaction by Payment Gateway ID.
* @param pgId PG ID (required)
* @return ApiResponse<PGTransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTransactionByPgIdWithHttpInfo(String pgId) throws ApiException {
com.squareup.okhttp.Call call = getTransactionByPgIdValidateBeforeCall(pgId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get transaction by PG ID (asynchronously)
* Gets a payment gateway transaction by Payment Gateway ID.
* @param pgId PG ID (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getTransactionByPgIdAsync(String pgId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getTransactionByPgIdValidateBeforeCall(pgId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTransactionRequests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getTransactionRequestsCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reporting/transaction-requests";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (count != null)
localVarQueryParams.addAll(apiClient.parameterToPair("count", count));
if (orderOn != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_on", orderOn));
if (orderBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("order_by", orderBy));
if (page != null)
localVarQueryParams.addAll(apiClient.parameterToPair("page", page));
if (filter != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter", filter));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "basicAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getTransactionRequestsValidateBeforeCall(Integer count, String orderOn, String orderBy, Integer page, String filter, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = getTransactionRequestsCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
return call;
}
/**
* Transaction Report
* Browses a paginated list of transaction requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return TransactionRequestResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionRequestResponse getTransactionRequests(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
ApiResponse resp = getTransactionRequestsWithHttpInfo(count, orderOn, orderBy, page, filter);
return resp.getData();
}
/**
* Transaction Report
* Browses a paginated list of transaction requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @return ApiResponse<TransactionRequestResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTransactionRequestsWithHttpInfo(Integer count, String orderOn, String orderBy, Integer page, String filter) throws ApiException {
com.squareup.okhttp.Call call = getTransactionRequestsValidateBeforeCall(count, orderOn, orderBy, page, filter, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Transaction Report (asynchronously)
* Browses a paginated list of transaction requests
* @param count The number of records in the result. (optional, default to 10)
* @param orderOn The field on which the results will be sorted on. Refer to the response model for available fields. (optional, default to tran_time)
* @param orderBy Ascending or Descending Sort order of the result. Possible values are: asc (Ascending sort order), desc (Descending sort order) (optional, default to desc)
* @param page Zero-based page number, use this to choose a page when there are more results than the count parameter. (optional, default to 0)
* @param filter Results can be filtered by custom filter criteria. Refer to [Filter](/developer/api/reference#filters) to use the filter parameter. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getTransactionRequestsAsync(Integer count, String orderOn, String orderBy, Integer page, String filter, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getTransactionRequestsValidateBeforeCall(count, orderOn, orderBy, page, filter, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy