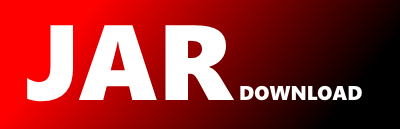
io.swagger.client.model.AddCustomerRequest Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.AddBillingCardRequest;
import io.swagger.client.model.AddShippingAddressRequest;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* AddCustomerRequest
*/
public class AddCustomerRequest {
@SerializedName("customer_id")
private String customerId = null;
@SerializedName("auto_generate_customer_id")
private Boolean autoGenerateCustomerId = null;
@SerializedName("customer_first_name")
private String customerFirstName = null;
@SerializedName("customer_last_name")
private String customerLastName = null;
@SerializedName("customer_firm_name")
private String customerFirmName = null;
@SerializedName("customer_phone")
private String customerPhone = null;
@SerializedName("customer_email")
private String customerEmail = null;
@SerializedName("comments")
private String comments = null;
@SerializedName("shipping_addresses")
private List shippingAddresses = null;
@SerializedName("billing_cards")
private List billingCards = null;
@SerializedName("merchant_id")
private Long merchantId = null;
public AddCustomerRequest customerId(String customerId) {
this.customerId = customerId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Unique ID established by merchant to identify a customer. The customer ID can be used to add subscriptions using the Recurring Billing API or make payments using the Payment Gateway API. Once established, this ID cannot be updated. This field is case sensitive. Only letters and numbers are allowed in a Customer ID.<br><strong>Conditional Requirement: </strong>Either customer_id or auto_generate_customer_id is required to add a new customer
* @return customerId
**/
@ApiModelProperty(example = "JOHNDOE", value = "Format: Variable length, up to 32 AN
Description: Unique ID established by merchant to identify a customer. The customer ID can be used to add subscriptions using the Recurring Billing API or make payments using the Payment Gateway API. Once established, this ID cannot be updated. This field is case sensitive. Only letters and numbers are allowed in a Customer ID.
Conditional Requirement: Either customer_id or auto_generate_customer_id is required to add a new customer")
public String getCustomerId() {
return customerId;
}
public void setCustomerId(String customerId) {
this.customerId = customerId;
}
public AddCustomerRequest autoGenerateCustomerId(Boolean autoGenerateCustomerId) {
this.autoGenerateCustomerId = autoGenerateCustomerId;
return this;
}
/**
* <br><strong>Description: </strong>Boolean flag that indicates if customer id should be auto generated. When set to true, Qualpay will generate a unique customer id. The generated customer_id will be returned in the response. Save the customer_id in the response to manage the customer through Vault API. <br><strong>Default: </strong>false<br><strong>Conditional Requirement: </strong>Either customer_id or auto_generate_customer_id is required to add a new customer
* @return autoGenerateCustomerId
**/
@ApiModelProperty(example = "true", value = "
Description: Boolean flag that indicates if customer id should be auto generated. When set to true, Qualpay will generate a unique customer id. The generated customer_id will be returned in the response. Save the customer_id in the response to manage the customer through Vault API.
Default: false
Conditional Requirement: Either customer_id or auto_generate_customer_id is required to add a new customer")
public Boolean isAutoGenerateCustomerId() {
return autoGenerateCustomerId;
}
public void setAutoGenerateCustomerId(Boolean autoGenerateCustomerId) {
this.autoGenerateCustomerId = autoGenerateCustomerId;
}
public AddCustomerRequest customerFirstName(String customerFirstName) {
this.customerFirstName = customerFirstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Customer's first name.<br><strong>Conditional Requirement: </strong>Either customer name or customer firm name is required.
* @return customerFirstName
**/
@ApiModelProperty(example = "JOHN", value = "Format: Variable length, up to 32 AN
Description: Customer's first name.
Conditional Requirement: Either customer name or customer firm name is required.")
public String getCustomerFirstName() {
return customerFirstName;
}
public void setCustomerFirstName(String customerFirstName) {
this.customerFirstName = customerFirstName;
}
public AddCustomerRequest customerLastName(String customerLastName) {
this.customerLastName = customerLastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Customer's last name.<br><strong>Conditional Requirement: </strong>Either customer name or customer firm name is required.
* @return customerLastName
**/
@ApiModelProperty(example = "Doe", value = "Format: Variable length, up to 32 AN
Description: Customer's last name.
Conditional Requirement: Either customer name or customer firm name is required.")
public String getCustomerLastName() {
return customerLastName;
}
public void setCustomerLastName(String customerLastName) {
this.customerLastName = customerLastName;
}
public AddCustomerRequest customerFirmName(String customerFirmName) {
this.customerFirmName = customerFirmName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Customer business name if applicable. <br><strong>Conditional Requirement: </strong>Either customer name or customer firm name is required.
* @return customerFirmName
**/
@ApiModelProperty(example = "Qualpay", value = "Format: Variable length, up to 64 AN
Description: Customer business name if applicable.
Conditional Requirement: Either customer name or customer firm name is required.")
public String getCustomerFirmName() {
return customerFirmName;
}
public void setCustomerFirmName(String customerFirmName) {
this.customerFirmName = customerFirmName;
}
public AddCustomerRequest customerPhone(String customerPhone) {
this.customerPhone = customerPhone;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Customer's phone number.
* @return customerPhone
**/
@ApiModelProperty(example = "999-999-9999", value = "Format: Variable length, up to 16 AN
Description: Customer's phone number.")
public String getCustomerPhone() {
return customerPhone;
}
public void setCustomerPhone(String customerPhone) {
this.customerPhone = customerPhone;
}
public AddCustomerRequest customerEmail(String customerEmail) {
this.customerEmail = customerEmail;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Customer's email address.
* @return customerEmail
**/
@ApiModelProperty(example = "[email protected]", value = "Format: Variable length, up to 64 AN
Description: Customer's email address. ")
public String getCustomerEmail() {
return customerEmail;
}
public void setCustomerEmail(String customerEmail) {
this.customerEmail = customerEmail;
}
public AddCustomerRequest comments(String comments) {
this.comments = comments;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Comment
* @return comments
**/
@ApiModelProperty(example = "Test comment", value = "Format: Variable length, up to 128 AN
Description: Comment")
public String getComments() {
return comments;
}
public void setComments(String comments) {
this.comments = comments;
}
public AddCustomerRequest shippingAddresses(List shippingAddresses) {
this.shippingAddresses = shippingAddresses;
return this;
}
public AddCustomerRequest addShippingAddressesItem(AddShippingAddressRequest shippingAddressesItem) {
if (this.shippingAddresses == null) {
this.shippingAddresses = new ArrayList();
}
this.shippingAddresses.add(shippingAddressesItem);
return this;
}
/**
* <br><strong>Description: </strong>An array of shipping addresses. One of the addresses should be designated as the default shipping address, if not, the system will choose one of the address to be the default.
* @return shippingAddresses
**/
@ApiModelProperty(value = "
Description: An array of shipping addresses. One of the addresses should be designated as the default shipping address, if not, the system will choose one of the address to be the default. ")
public List getShippingAddresses() {
return shippingAddresses;
}
public void setShippingAddresses(List shippingAddresses) {
this.shippingAddresses = shippingAddresses;
}
public AddCustomerRequest billingCards(List billingCards) {
this.billingCards = billingCards;
return this;
}
public AddCustomerRequest addBillingCardsItem(AddBillingCardRequest billingCardsItem) {
if (this.billingCards == null) {
this.billingCards = new ArrayList();
}
this.billingCards.add(billingCardsItem);
return this;
}
/**
* <br><strong>Description: </strong>An array of billing cards. One of the cards should be designated as the default billing card, if not, the system will choose one of the cards to be the default.
* @return billingCards
**/
@ApiModelProperty(value = "
Description: An array of billing cards. One of the cards should be designated as the default billing card, if not, the system will choose one of the cards to be the default. ")
public List getBillingCards() {
return billingCards;
}
public void setBillingCards(List billingCards) {
this.billingCards = billingCards;
}
public AddCustomerRequest merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Identifies the merchant to whom this request applies. Optional field, applicable only if the request is sent on behalf of another merchant.<br><strong>Conditional Requirement: </strong>Required if this request is on behalf of another merchant.
* @return merchantId
**/
@ApiModelProperty(example = "210000000289", value = "Format: Variable length, up to 16 AN
Description: Identifies the merchant to whom this request applies. Optional field, applicable only if the request is sent on behalf of another merchant.
Conditional Requirement: Required if this request is on behalf of another merchant.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AddCustomerRequest addCustomerRequest = (AddCustomerRequest) o;
return Objects.equals(this.customerId, addCustomerRequest.customerId) &&
Objects.equals(this.autoGenerateCustomerId, addCustomerRequest.autoGenerateCustomerId) &&
Objects.equals(this.customerFirstName, addCustomerRequest.customerFirstName) &&
Objects.equals(this.customerLastName, addCustomerRequest.customerLastName) &&
Objects.equals(this.customerFirmName, addCustomerRequest.customerFirmName) &&
Objects.equals(this.customerPhone, addCustomerRequest.customerPhone) &&
Objects.equals(this.customerEmail, addCustomerRequest.customerEmail) &&
Objects.equals(this.comments, addCustomerRequest.comments) &&
Objects.equals(this.shippingAddresses, addCustomerRequest.shippingAddresses) &&
Objects.equals(this.billingCards, addCustomerRequest.billingCards) &&
Objects.equals(this.merchantId, addCustomerRequest.merchantId);
}
@Override
public int hashCode() {
return Objects.hash(customerId, autoGenerateCustomerId, customerFirstName, customerLastName, customerFirmName, customerPhone, customerEmail, comments, shippingAddresses, billingCards, merchantId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AddCustomerRequest {\n");
sb.append(" customerId: ").append(toIndentedString(customerId)).append("\n");
sb.append(" autoGenerateCustomerId: ").append(toIndentedString(autoGenerateCustomerId)).append("\n");
sb.append(" customerFirstName: ").append(toIndentedString(customerFirstName)).append("\n");
sb.append(" customerLastName: ").append(toIndentedString(customerLastName)).append("\n");
sb.append(" customerFirmName: ").append(toIndentedString(customerFirmName)).append("\n");
sb.append(" customerPhone: ").append(toIndentedString(customerPhone)).append("\n");
sb.append(" customerEmail: ").append(toIndentedString(customerEmail)).append("\n");
sb.append(" comments: ").append(toIndentedString(comments)).append("\n");
sb.append(" shippingAddresses: ").append(toIndentedString(shippingAddresses)).append("\n");
sb.append(" billingCards: ").append(toIndentedString(billingCards)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy