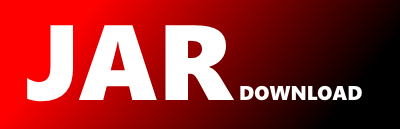
io.swagger.client.model.ApplicationModel Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.OffsetDateTime;
/**
* ApplicationModel
*/
public class ApplicationModel {
@SerializedName("app_id")
private Long appId = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("app_status")
private String appStatus = null;
@SerializedName("dba_name")
private String dbaName = null;
@SerializedName("db_timestamp")
private OffsetDateTime dbTimestamp = null;
@SerializedName("submit_timestamp")
private OffsetDateTime submitTimestamp = null;
@SerializedName("credit_timestamp")
private OffsetDateTime creditTimestamp = null;
/**
* Unique ID assigned by Qualpay to this application.
* @return appId
**/
@ApiModelProperty(example = "1", value = "Unique ID assigned by Qualpay to this application.")
public Long getAppId() {
return appId;
}
/**
* Unique ID assigned by Qualpay to a merchant.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Unique ID assigned by Qualpay to a merchant.")
public Long getMerchantId() {
return merchantId;
}
/**
* The current status of the application.
* @return appStatus
**/
@ApiModelProperty(example = "INCOMPLETE", value = "The current status of the application.")
public String getAppStatus() {
return appStatus;
}
/**
* The 'doing business as' name, as it is currently on the merchant application.
* @return dbaName
**/
@ApiModelProperty(example = "Ben's Bar", value = "The 'doing business as' name, as it is currently on the merchant application.")
public String getDbaName() {
return dbaName;
}
/**
* The timestamp the application was created.
* @return dbTimestamp
**/
@ApiModelProperty(example = "2016-07-01 22:22:22", value = "The timestamp the application was created.")
public OffsetDateTime getDbTimestamp() {
return dbTimestamp;
}
/**
* The timestamp the application was submitted as complete.
* @return submitTimestamp
**/
@ApiModelProperty(example = "2016-07-01 22:22:22", value = "The timestamp the application was submitted as complete.")
public OffsetDateTime getSubmitTimestamp() {
return submitTimestamp;
}
/**
* The timestamp the application's credit decision was made.
* @return creditTimestamp
**/
@ApiModelProperty(example = "2016-07-01 22:22:22", value = "The timestamp the application's credit decision was made.")
public OffsetDateTime getCreditTimestamp() {
return creditTimestamp;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ApplicationModel applicationModel = (ApplicationModel) o;
return Objects.equals(this.appId, applicationModel.appId) &&
Objects.equals(this.merchantId, applicationModel.merchantId) &&
Objects.equals(this.appStatus, applicationModel.appStatus) &&
Objects.equals(this.dbaName, applicationModel.dbaName) &&
Objects.equals(this.dbTimestamp, applicationModel.dbTimestamp) &&
Objects.equals(this.submitTimestamp, applicationModel.submitTimestamp) &&
Objects.equals(this.creditTimestamp, applicationModel.creditTimestamp);
}
@Override
public int hashCode() {
return Objects.hash(appId, merchantId, appStatus, dbaName, dbTimestamp, submitTimestamp, creditTimestamp);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApplicationModel {\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" appStatus: ").append(toIndentedString(appStatus)).append("\n");
sb.append(" dbaName: ").append(toIndentedString(dbaName)).append("\n");
sb.append(" dbTimestamp: ").append(toIndentedString(dbTimestamp)).append("\n");
sb.append(" submitTimestamp: ").append(toIndentedString(submitTimestamp)).append("\n");
sb.append(" creditTimestamp: ").append(toIndentedString(creditTimestamp)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy