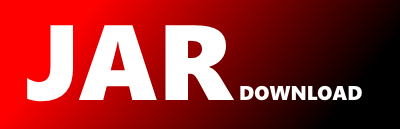
io.swagger.client.model.CheckoutLink Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* CheckoutLink
*/
public class CheckoutLink {
@SerializedName("checkout_id")
private String checkoutId = null;
@SerializedName("checkout_link")
private String checkoutLink = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("purchase_id")
private String purchaseId = null;
@SerializedName("amt_tran")
private Double amtTran = null;
@SerializedName("tran_currency")
private String tranCurrency = null;
@SerializedName("db_timestamp")
private String dbTimestamp = null;
@SerializedName("expiry_time")
private String expiryTime = null;
public CheckoutLink checkoutId(String checkoutId) {
this.checkoutId = checkoutId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>A unique identifier that is generated when a checkout record is created. It can be stored as a reference to the checkout and used to pull payment details.
* @return checkoutId
**/
@ApiModelProperty(example = "a393941797c811e6825102a019999999", value = "Format: Fixed length, 32 AN
Description: A unique identifier that is generated when a checkout record is created. It can be stored as a reference to the checkout and used to pull payment details.")
public String getCheckoutId() {
return checkoutId;
}
public void setCheckoutId(String checkoutId) {
this.checkoutId = checkoutId;
}
public CheckoutLink checkoutLink(String checkoutLink) {
this.checkoutLink = checkoutLink;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>A redirect link that will direct a customer to the Qualpay payment page. This link is unique for each checkout.
* @return checkoutLink
**/
@ApiModelProperty(example = "https://app.qualpay.com/checkout/a393941797c811e6825102a019999999", value = "Format: Variable length, up to 128 AN
Description: A redirect link that will direct a customer to the Qualpay payment page. This link is unique for each checkout.")
public String getCheckoutLink() {
return checkoutLink;
}
public void setCheckoutLink(String checkoutLink) {
this.checkoutLink = checkoutLink;
}
public CheckoutLink merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 20 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to a Merchant
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Format: Variable length, up to 20 N
Description: Unique ID assigned by Qualpay to a Merchant")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public CheckoutLink purchaseId(String purchaseId) {
this.purchaseId = purchaseId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 25 AN<br><strong>Description: </strong>The purchase identifier (also referred to as the invoice number) generated by the merchant.
* @return purchaseId
**/
@ApiModelProperty(example = "PID#2345", value = "Format: Variable length, up to 25 AN
Description: The purchase identifier (also referred to as the invoice number) generated by the merchant.")
public String getPurchaseId() {
return purchaseId;
}
public void setPurchaseId(String purchaseId) {
this.purchaseId = purchaseId;
}
public CheckoutLink amtTran(Double amtTran) {
this.amtTran = amtTran;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 12,2 N<br><strong>Description: </strong>Transaction amount from the request message.
* @return amtTran
**/
@ApiModelProperty(example = "4.56", value = "Format: Variable length, up to 12,2 N
Description: Transaction amount from the request message.")
public Double getAmtTran() {
return amtTran;
}
public void setAmtTran(Double amtTran) {
this.amtTran = amtTran;
}
public CheckoutLink tranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>Numeric currency code of the checkout transaction. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of currency codes.
* @return tranCurrency
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: Numeric currency code of the checkout transaction. Refer to Country Codes for a list of currency codes.")
public String getTranCurrency() {
return tranCurrency;
}
public void setTranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
}
public CheckoutLink dbTimestamp(String dbTimestamp) {
this.dbTimestamp = dbTimestamp;
return this;
}
/**
* <strong>Format: </strong>Variable length AN<br><strong>Description: </strong>This field contains the request creation timestamp.
* @return dbTimestamp
**/
@ApiModelProperty(example = "2016-02-21T12:57:47.000-0700", value = "Format: Variable length AN
Description: This field contains the request creation timestamp. ")
public String getDbTimestamp() {
return dbTimestamp;
}
public void setDbTimestamp(String dbTimestamp) {
this.dbTimestamp = dbTimestamp;
}
public CheckoutLink expiryTime(String expiryTime) {
this.expiryTime = expiryTime;
return this;
}
/**
* <strong>Format: </strong>Variable length AN<br><strong>Default: </strong>This field contains the timestamp when the checkout_link will expire. A link will expire once the complete payment is made or if the current date is past the expiry time. Once a link expires, redirects to the link will be forwarded to your failure URL if provided or to the Qualpay Checkout error page if failure URL is not provided.
* @return expiryTime
**/
@ApiModelProperty(example = "2016-02-21T14:37:47.000-0700", value = "Format: Variable length AN
Default: This field contains the timestamp when the checkout_link will expire. A link will expire once the complete payment is made or if the current date is past the expiry time. Once a link expires, redirects to the link will be forwarded to your failure URL if provided or to the Qualpay Checkout error page if failure URL is not provided. ")
public String getExpiryTime() {
return expiryTime;
}
public void setExpiryTime(String expiryTime) {
this.expiryTime = expiryTime;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckoutLink checkoutLink = (CheckoutLink) o;
return Objects.equals(this.checkoutId, checkoutLink.checkoutId) &&
Objects.equals(this.checkoutLink, checkoutLink.checkoutLink) &&
Objects.equals(this.merchantId, checkoutLink.merchantId) &&
Objects.equals(this.purchaseId, checkoutLink.purchaseId) &&
Objects.equals(this.amtTran, checkoutLink.amtTran) &&
Objects.equals(this.tranCurrency, checkoutLink.tranCurrency) &&
Objects.equals(this.dbTimestamp, checkoutLink.dbTimestamp) &&
Objects.equals(this.expiryTime, checkoutLink.expiryTime);
}
@Override
public int hashCode() {
return Objects.hash(checkoutId, checkoutLink, merchantId, purchaseId, amtTran, tranCurrency, dbTimestamp, expiryTime);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckoutLink {\n");
sb.append(" checkoutId: ").append(toIndentedString(checkoutId)).append("\n");
sb.append(" checkoutLink: ").append(toIndentedString(checkoutLink)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" purchaseId: ").append(toIndentedString(purchaseId)).append("\n");
sb.append(" amtTran: ").append(toIndentedString(amtTran)).append("\n");
sb.append(" tranCurrency: ").append(toIndentedString(tranCurrency)).append("\n");
sb.append(" dbTimestamp: ").append(toIndentedString(dbTimestamp)).append("\n");
sb.append(" expiryTime: ").append(toIndentedString(expiryTime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy