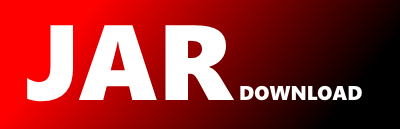
io.swagger.client.model.CheckoutPreferences Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* CheckoutPreferences
*/
public class CheckoutPreferences {
@SerializedName("success_url")
private String successUrl = null;
@SerializedName("failure_url")
private String failureUrl = null;
@SerializedName("notification_url")
private String notificationUrl = null;
@SerializedName("allow_partial_payments")
private Boolean allowPartialPayments = null;
@SerializedName("allow_save_card")
private Boolean allowSaveCard = null;
@SerializedName("allow_ach_payment")
private Boolean allowAchPayment = null;
@SerializedName("email_receipt")
private Boolean emailReceipt = null;
/**
* <strong>Format: </strong>Variable length, up to 10 AN<br><strong>Description: </strong>Identifies the type of request when the customer submits the payment data on the checkout page.
*/
@JsonAdapter(RequestTypeEnum.Adapter.class)
public enum RequestTypeEnum {
AUTH("auth"),
SALE("sale");
private String value;
RequestTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static RequestTypeEnum fromValue(String text) {
for (RequestTypeEnum b : RequestTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final RequestTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public RequestTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return RequestTypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("request_type")
private RequestTypeEnum requestType = null;
@SerializedName("expire_in_secs")
private Integer expireInSecs = null;
public CheckoutPreferences successUrl(String successUrl) {
this.successUrl = successUrl;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>A URL to which the customer will be directed after a successful payment. If not defined in the API or in the checkout settings page, the customer will be directed to the default Qualpay receipt page. Must be formatted as http://www.domain.com/ or https://www.domain.com/.
* @return successUrl
**/
@ApiModelProperty(example = "https:/yourcompany.com/payment/success", value = "Format: Variable length, up to 128 AN
Description: A URL to which the customer will be directed after a successful payment. If not defined in the API or in the checkout settings page, the customer will be directed to the default Qualpay receipt page. Must be formatted as http://www.domain.com/ or https://www.domain.com/. ")
public String getSuccessUrl() {
return successUrl;
}
public void setSuccessUrl(String successUrl) {
this.successUrl = successUrl;
}
public CheckoutPreferences failureUrl(String failureUrl) {
this.failureUrl = failureUrl;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>A URL to which the customer will be directed if a transaction is declined. If not defined in the API or in the checkout settings page, the customer will be directed to the default Qualpay receipt page. Must be formatted as http://www.domain.com/ or https://www.domain.com/.
* @return failureUrl
**/
@ApiModelProperty(example = "https://yourcompany.com.com/payment/fail", value = "Format: Variable length, up to 128 AN
Description: A URL to which the customer will be directed if a transaction is declined. If not defined in the API or in the checkout settings page, the customer will be directed to the default Qualpay receipt page. Must be formatted as http://www.domain.com/ or https://www.domain.com/. ")
public String getFailureUrl() {
return failureUrl;
}
public void setFailureUrl(String failureUrl) {
this.failureUrl = failureUrl;
}
public CheckoutPreferences notificationUrl(String notificationUrl) {
this.notificationUrl = notificationUrl;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>A merchant provided callback service that will be notified whenever a hosted checkout payment is made. Qualpay will send a POST message to the URI. <strong>Deprecated. Use Qualpay webhooks for callbacks.</strong>
* @return notificationUrl
**/
@ApiModelProperty(example = "https://www.qualpay.com/checkout/service", value = "Format: Variable length, up to 128 AN
Description: A merchant provided callback service that will be notified whenever a hosted checkout payment is made. Qualpay will send a POST message to the URI. Deprecated. Use Qualpay webhooks for callbacks. ")
public String getNotificationUrl() {
return notificationUrl;
}
public void setNotificationUrl(String notificationUrl) {
this.notificationUrl = notificationUrl;
}
public CheckoutPreferences allowPartialPayments(Boolean allowPartialPayments) {
this.allowPartialPayments = allowPartialPayments;
return this;
}
/**
* <br><strong>Description: </strong>If set to true, the customer can make changes to the transaction amount.
* @return allowPartialPayments
**/
@ApiModelProperty(example = "false", value = "
Description: If set to true, the customer can make changes to the transaction amount.")
public Boolean isAllowPartialPayments() {
return allowPartialPayments;
}
public void setAllowPartialPayments(Boolean allowPartialPayments) {
this.allowPartialPayments = allowPartialPayments;
}
public CheckoutPreferences allowSaveCard(Boolean allowSaveCard) {
this.allowSaveCard = allowSaveCard;
return this;
}
/**
* <br><strong>Description: </strong>Applicable only for checkouts associated with a Qualpay customer. If set to true, the checkout page will display the 'Save Card' checkbox that lets the customer decide if the card information can be saved. If set to false, the 'Save Card' box is not displayed and the customer card information is always updated.<br><strong>Default: </strong>false
* @return allowSaveCard
**/
@ApiModelProperty(example = "false", value = "
Description: Applicable only for checkouts associated with a Qualpay customer. If set to true, the checkout page will display the 'Save Card' checkbox that lets the customer decide if the card information can be saved. If set to false, the 'Save Card' box is not displayed and the customer card information is always updated.
Default: false")
public Boolean isAllowSaveCard() {
return allowSaveCard;
}
public void setAllowSaveCard(Boolean allowSaveCard) {
this.allowSaveCard = allowSaveCard;
}
public CheckoutPreferences allowAchPayment(Boolean allowAchPayment) {
this.allowAchPayment = allowAchPayment;
return this;
}
/**
* <br><strong>Description: </strong>Applicable only if your merchant account is configured to take ACH payments. If set to true, the checkout page will present ACH as one of the payment modes to the customer. <br><strong>Default: </strong>false
* @return allowAchPayment
**/
@ApiModelProperty(example = "true", value = "
Description: Applicable only if your merchant account is configured to take ACH payments. If set to true, the checkout page will present ACH as one of the payment modes to the customer.
Default: false")
public Boolean isAllowAchPayment() {
return allowAchPayment;
}
public void setAllowAchPayment(Boolean allowAchPayment) {
this.allowAchPayment = allowAchPayment;
}
public CheckoutPreferences emailReceipt(Boolean emailReceipt) {
this.emailReceipt = emailReceipt;
return this;
}
/**
* <br><strong>Description: </strong>If set to true and the customer email address is provided, a receipt is sent to the customer.
* @return emailReceipt
**/
@ApiModelProperty(example = "false", value = "
Description: If set to true and the customer email address is provided, a receipt is sent to the customer. ")
public Boolean isEmailReceipt() {
return emailReceipt;
}
public void setEmailReceipt(Boolean emailReceipt) {
this.emailReceipt = emailReceipt;
}
public CheckoutPreferences requestType(RequestTypeEnum requestType) {
this.requestType = requestType;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN<br><strong>Description: </strong>Identifies the type of request when the customer submits the payment data on the checkout page.
* @return requestType
**/
@ApiModelProperty(example = "sale", value = "Format: Variable length, up to 10 AN
Description: Identifies the type of request when the customer submits the payment data on the checkout page. ")
public RequestTypeEnum getRequestType() {
return requestType;
}
public void setRequestType(RequestTypeEnum requestType) {
this.requestType = requestType;
}
public CheckoutPreferences expireInSecs(Integer expireInSecs) {
this.expireInSecs = expireInSecs;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 7 N<br><strong>Description: </strong>The time period for which the checkout_link will be valid in seconds. The minimum value for this field is 900 seconds (5 minutes), the maximum value is 7776000 (90 days).
* @return expireInSecs
**/
@ApiModelProperty(example = "6000", value = "Format: Variable length, up to 7 N
Description: The time period for which the checkout_link will be valid in seconds. The minimum value for this field is 900 seconds (5 minutes), the maximum value is 7776000 (90 days).")
public Integer getExpireInSecs() {
return expireInSecs;
}
public void setExpireInSecs(Integer expireInSecs) {
this.expireInSecs = expireInSecs;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckoutPreferences checkoutPreferences = (CheckoutPreferences) o;
return Objects.equals(this.successUrl, checkoutPreferences.successUrl) &&
Objects.equals(this.failureUrl, checkoutPreferences.failureUrl) &&
Objects.equals(this.notificationUrl, checkoutPreferences.notificationUrl) &&
Objects.equals(this.allowPartialPayments, checkoutPreferences.allowPartialPayments) &&
Objects.equals(this.allowSaveCard, checkoutPreferences.allowSaveCard) &&
Objects.equals(this.allowAchPayment, checkoutPreferences.allowAchPayment) &&
Objects.equals(this.emailReceipt, checkoutPreferences.emailReceipt) &&
Objects.equals(this.requestType, checkoutPreferences.requestType) &&
Objects.equals(this.expireInSecs, checkoutPreferences.expireInSecs);
}
@Override
public int hashCode() {
return Objects.hash(successUrl, failureUrl, notificationUrl, allowPartialPayments, allowSaveCard, allowAchPayment, emailReceipt, requestType, expireInSecs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckoutPreferences {\n");
sb.append(" successUrl: ").append(toIndentedString(successUrl)).append("\n");
sb.append(" failureUrl: ").append(toIndentedString(failureUrl)).append("\n");
sb.append(" notificationUrl: ").append(toIndentedString(notificationUrl)).append("\n");
sb.append(" allowPartialPayments: ").append(toIndentedString(allowPartialPayments)).append("\n");
sb.append(" allowSaveCard: ").append(toIndentedString(allowSaveCard)).append("\n");
sb.append(" allowAchPayment: ").append(toIndentedString(allowAchPayment)).append("\n");
sb.append(" emailReceipt: ").append(toIndentedString(emailReceipt)).append("\n");
sb.append(" requestType: ").append(toIndentedString(requestType)).append("\n");
sb.append(" expireInSecs: ").append(toIndentedString(expireInSecs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy