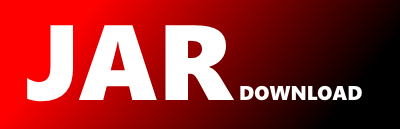
io.swagger.client.model.CheckoutSettings Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* CheckoutSettings
*/
public class CheckoutSettings {
@SerializedName("allow_partial_payments")
private Boolean allowPartialPayments = null;
@SerializedName("email_receipt")
private Boolean emailReceipt = null;
@SerializedName("allow_ach_payment")
private Boolean allowAchPayment = null;
public CheckoutSettings allowPartialPayments(Boolean allowPartialPayments) {
this.allowPartialPayments = allowPartialPayments;
return this;
}
/**
* <br><strong>Description: </strong>If set to true, the customer can edit the transaction amount when making payment on the Invoice checkout page. Set this flag to true if you want to allow the customer to make partial payments.
* @return allowPartialPayments
**/
@ApiModelProperty(example = "false", value = "
Description: If set to true, the customer can edit the transaction amount when making payment on the Invoice checkout page. Set this flag to true if you want to allow the customer to make partial payments. ")
public Boolean isAllowPartialPayments() {
return allowPartialPayments;
}
public void setAllowPartialPayments(Boolean allowPartialPayments) {
this.allowPartialPayments = allowPartialPayments;
}
public CheckoutSettings emailReceipt(Boolean emailReceipt) {
this.emailReceipt = emailReceipt;
return this;
}
/**
* <br><strong>Description: </strong>If set to true and the customer email address is provided, Qualpay generates and sends a receipt to the customer once a payment is made on the invoice checkout page.
* @return emailReceipt
**/
@ApiModelProperty(example = "false", value = "
Description: If set to true and the customer email address is provided, Qualpay generates and sends a receipt to the customer once a payment is made on the invoice checkout page. ")
public Boolean isEmailReceipt() {
return emailReceipt;
}
public void setEmailReceipt(Boolean emailReceipt) {
this.emailReceipt = emailReceipt;
}
public CheckoutSettings allowAchPayment(Boolean allowAchPayment) {
this.allowAchPayment = allowAchPayment;
return this;
}
/**
* <br><strong>Description: </strong>Applicable only if your merchant account is configured to take ACH payments. If set to true, the checkout page will present ACH as one of the payment modes to the customer. <br><strong>Default: </strong>false
* @return allowAchPayment
**/
@ApiModelProperty(example = "true", value = "
Description: Applicable only if your merchant account is configured to take ACH payments. If set to true, the checkout page will present ACH as one of the payment modes to the customer.
Default: false")
public Boolean isAllowAchPayment() {
return allowAchPayment;
}
public void setAllowAchPayment(Boolean allowAchPayment) {
this.allowAchPayment = allowAchPayment;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckoutSettings checkoutSettings = (CheckoutSettings) o;
return Objects.equals(this.allowPartialPayments, checkoutSettings.allowPartialPayments) &&
Objects.equals(this.emailReceipt, checkoutSettings.emailReceipt) &&
Objects.equals(this.allowAchPayment, checkoutSettings.allowAchPayment);
}
@Override
public int hashCode() {
return Objects.hash(allowPartialPayments, emailReceipt, allowAchPayment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckoutSettings {\n");
sb.append(" allowPartialPayments: ").append(toIndentedString(allowPartialPayments)).append("\n");
sb.append(" emailReceipt: ").append(toIndentedString(emailReceipt)).append("\n");
sb.append(" allowAchPayment: ").append(toIndentedString(allowAchPayment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy