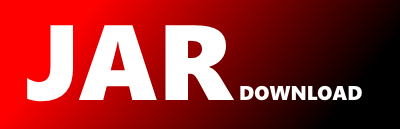
io.swagger.client.model.Contact Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Contact
*/
public class Contact {
@SerializedName("first_name")
private String firstName = null;
@SerializedName("last_name")
private String lastName = null;
@SerializedName("firm_name")
private String firmName = null;
@SerializedName("address1")
private String address1 = null;
@SerializedName("address2")
private String address2 = null;
@SerializedName("city")
private String city = null;
@SerializedName("state")
private String state = null;
@SerializedName("zip")
private String zip = null;
@SerializedName("zip4")
private String zip4 = null;
@SerializedName("phone")
private String phone = null;
@SerializedName("email_address")
private String emailAddress = null;
@SerializedName("country_code")
private String countryCode = null;
@SerializedName("country")
private String country = null;
public Contact firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>First name of the contact.<br><strong>Conditional Requirement: </strong>Required for billing_contact.
* @return firstName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: First name of the contact.
Conditional Requirement: Required for billing_contact.")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public Contact lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Last name of the contact.<br><strong>Conditional Requirement: </strong>Required for billing_contact.
* @return lastName
**/
@ApiModelProperty(example = "Doe", value = "Format: Variable length, up to 32 AN
Description: Last name of the contact.
Conditional Requirement: Required for billing_contact.")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public Contact firmName(String firmName) {
this.firmName = firmName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Contact business name, if applicable.<br><strong>Conditional Requirement: </strong>Required for from_contact.
* @return firmName
**/
@ApiModelProperty(example = "Qualpay", value = "Format: Variable length, up to 64 AN
Description: Contact business name, if applicable.
Conditional Requirement: Required for from_contact.")
public String getFirmName() {
return firmName;
}
public void setFirmName(String firmName) {
this.firmName = firmName;
}
public Contact address1(String address1) {
this.address1 = address1;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Contact address line item 1.
* @return address1
**/
@ApiModelProperty(example = "4W 4th Avenue", value = "Format: Variable length, up to 128 AN
Description: Contact address line item 1.")
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public Contact address2(String address2) {
this.address2 = address2;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Contact address line item 2.
* @return address2
**/
@ApiModelProperty(example = "#401", value = "Format: Variable length, up to 128 AN
Description: Contact address line item 2.")
public String getAddress2() {
return address2;
}
public void setAddress2(String address2) {
this.address2 = address2;
}
public Contact city(String city) {
this.city = city;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Contact city.
* @return city
**/
@ApiModelProperty(example = "San Mateo", value = "Format: Variable length, up to 64 AN
Description: Contact city.")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public Contact state(String state) {
this.state = state;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Contact state.
* @return state
**/
@ApiModelProperty(example = "CA", value = "Format: Variable length, up to 64 AN
Description: Contact state.")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public Contact zip(String zip) {
this.zip = zip;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN<br><strong>Description: </strong>Contact zip.
* @return zip
**/
@ApiModelProperty(example = "94404", value = "Format: Variable length, up to 10 AN
Description: Contact zip.")
public String getZip() {
return zip;
}
public void setZip(String zip) {
this.zip = zip;
}
public Contact zip4(String zip4) {
this.zip4 = zip4;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 4 N<br><strong>Description: </strong>Contact zip+4 code if applicable.
* @return zip4
**/
@ApiModelProperty(example = "1234", value = "Format: Variable length, up to 4 N
Description: Contact zip+4 code if applicable.")
public String getZip4() {
return zip4;
}
public void setZip4(String zip4) {
this.zip4 = zip4;
}
public Contact phone(String phone) {
this.phone = phone;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Contact's phone number.<br><strong>Conditional Requirement: </strong>Required for from_contact.
* @return phone
**/
@ApiModelProperty(example = "9999999999", value = "Format: Variable length, up to 32 AN
Description: Contact's phone number.
Conditional Requirement: Required for from_contact.")
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Contact emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Contact's email address.
* @return emailAddress
**/
@ApiModelProperty(example = "[email protected]", required = true, value = "Format: Variable length, up to 64 AN
Description: Contact's email address.")
public String getEmailAddress() {
return emailAddress;
}
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
public Contact countryCode(String countryCode) {
this.countryCode = countryCode;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 3 AN<br><strong>Description: </strong>ISO numeric country code for the contact. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of country codes.<br>
* @return countryCode
**/
@ApiModelProperty(example = "840", value = "Format: Variable length, up to 3 AN
Description: ISO numeric country code for the contact. Refer to Country Codes for a list of country codes.
")
public String getCountryCode() {
return countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
public Contact country(String country) {
this.country = country;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Country name.
* @return country
**/
@ApiModelProperty(example = "United States", value = "Format: Variable length, up to 128 AN
Description: Country name.")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Contact contact = (Contact) o;
return Objects.equals(this.firstName, contact.firstName) &&
Objects.equals(this.lastName, contact.lastName) &&
Objects.equals(this.firmName, contact.firmName) &&
Objects.equals(this.address1, contact.address1) &&
Objects.equals(this.address2, contact.address2) &&
Objects.equals(this.city, contact.city) &&
Objects.equals(this.state, contact.state) &&
Objects.equals(this.zip, contact.zip) &&
Objects.equals(this.zip4, contact.zip4) &&
Objects.equals(this.phone, contact.phone) &&
Objects.equals(this.emailAddress, contact.emailAddress) &&
Objects.equals(this.countryCode, contact.countryCode) &&
Objects.equals(this.country, contact.country);
}
@Override
public int hashCode() {
return Objects.hash(firstName, lastName, firmName, address1, address2, city, state, zip, zip4, phone, emailAddress, countryCode, country);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Contact {\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" firmName: ").append(toIndentedString(firmName)).append("\n");
sb.append(" address1: ").append(toIndentedString(address1)).append("\n");
sb.append(" address2: ").append(toIndentedString(address2)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" zip: ").append(toIndentedString(zip)).append("\n");
sb.append(" zip4: ").append(toIndentedString(zip4)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" emailAddress: ").append(toIndentedString(emailAddress)).append("\n");
sb.append(" countryCode: ").append(toIndentedString(countryCode)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy