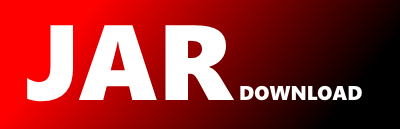
io.swagger.client.model.DisputeReport Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* DisputeReport
*/
public class DisputeReport {
@SerializedName("rec_id")
private Long recId = null;
@SerializedName("data_type")
private String dataType = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("incoming_date")
private String incomingDate = null;
@SerializedName("dba_name")
private String dbaName = null;
@SerializedName("batch_id")
private Long batchId = null;
@SerializedName("card_number")
private String cardNumber = null;
/**
* <strong>Format: </strong>Fixed length, 2 AN<br><strong>Description: </strong>The card brand of this dispute.
*/
@JsonAdapter(CardTypeEnum.Adapter.class)
public enum CardTypeEnum {
VS("VS"),
MC("MC"),
DS("DS"),
AM("AM");
private String value;
CardTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CardTypeEnum fromValue(String text) {
for (CardTypeEnum b : CardTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CardTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CardTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CardTypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("card_type")
private CardTypeEnum cardType = null;
@SerializedName("tran_date")
private String tranDate = null;
@SerializedName("amt_tran")
private String amtTran = null;
@SerializedName("amt_dispute")
private String amtDispute = null;
@SerializedName("tran_currency")
private String tranCurrency = null;
@SerializedName("purchase_id")
private String purchaseId = null;
@SerializedName("merch_ref_num")
private String merchRefNum = null;
@SerializedName("pg_id")
private String pgId = null;
@SerializedName("auth_code")
private String authCode = null;
@SerializedName("reason_code")
private String reasonCode = null;
@SerializedName("rec_id_linked")
private String recIdLinked = null;
@SerializedName("auth_avs_result")
private String authAvsResult = null;
@SerializedName("auth_cvv2_result")
private String authCvv2Result = null;
@SerializedName("card_number_original")
private String cardNumberOriginal = null;
@SerializedName("date_status_change")
private String dateStatusChange = null;
/**
* <strong>Format: </strong>Variable length, up to 18 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to this dispute.
* @return recId
**/
@ApiModelProperty(example = "1", required = true, value = "Format: Variable length, up to 18 N
Description: Unique ID assigned by Qualpay to this dispute.")
public Long getRecId() {
return recId;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>The type of dispute. For all types, please see <a href=\"/developer/api/reference#data_type\" target=\"_blank\">Dispute Types</a>.
* @return dataType
**/
@ApiModelProperty(example = "C", required = true, value = "Format: Fixed length, 1 AN
Description: The type of dispute. For all types, please see Dispute Types.")
public String getDataType() {
return dataType;
}
/**
* <strong>Format: </strong>Variable length, up to 16 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to a merchant.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", required = true, value = "Format: Variable length, up to 16 N
Description: Unique ID assigned by Qualpay to a merchant.")
public Long getMerchantId() {
return merchantId;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, in YYYY-MM-DD format<br><strong>Description: </strong>The date Qualpay received the dispute from the card issuer.
* @return incomingDate
**/
@ApiModelProperty(example = "2016-07-01", required = true, value = "Format: Variable length, up to 10 AN, in YYYY-MM-DD format
Description: The date Qualpay received the dispute from the card issuer.")
public String getIncomingDate() {
return incomingDate;
}
/**
* <strong>Format: </strong>Variable length, up to 25 AN<br><strong>Description: </strong>The doing business as name of the merchant.
* @return dbaName
**/
@ApiModelProperty(example = "Qualpay", required = true, value = "Format: Variable length, up to 25 AN
Description: The doing business as name of the merchant.")
public String getDbaName() {
return dbaName;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to this batch.
* @return batchId
**/
@ApiModelProperty(example = "1", required = true, value = "Format: Variable length, up to 10 N
Description: Unique ID assigned by Qualpay to this batch.")
public Long getBatchId() {
return batchId;
}
/**
* <strong>Format: </strong>Fixed length, 16 AN<br><strong>Description: </strong>The truncated card number of the dispute.
* @return cardNumber
**/
@ApiModelProperty(example = "411111xxxxxx1111", required = true, value = "Format: Fixed length, 16 AN
Description: The truncated card number of the dispute.")
public String getCardNumber() {
return cardNumber;
}
/**
* <strong>Format: </strong>Fixed length, 2 AN<br><strong>Description: </strong>The card brand of this dispute.
* @return cardType
**/
@ApiModelProperty(example = "VS", required = true, value = "Format: Fixed length, 2 AN
Description: The card brand of this dispute.")
public CardTypeEnum getCardType() {
return cardType;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, in YYYY-MM-DD format<br><strong>Description: </strong>The date the initial transaction occured.
* @return tranDate
**/
@ApiModelProperty(example = "2016-07-01", required = true, value = "Format: Variable length, up to 10 AN, in YYYY-MM-DD format
Description: The date the initial transaction occured.")
public String getTranDate() {
return tranDate;
}
/**
* <strong>Format: </strong>Variable length, up to 12,2 N<br><strong>Description: </strong>The amount of the original settled transaction.
* @return amtTran
**/
@ApiModelProperty(example = "1.00", required = true, value = "Format: Variable length, up to 12,2 N
Description: The amount of the original settled transaction.")
public String getAmtTran() {
return amtTran;
}
/**
* <strong>Format: </strong>Variable length, up to 12,2 N<br><strong>Description: </strong>The amount of the dispute: equal to, or less than the amt_tran (when in USD).
* @return amtDispute
**/
@ApiModelProperty(example = "1.00", required = true, value = "Format: Variable length, up to 12,2 N
Description: The amount of the dispute: equal to, or less than the amt_tran (when in USD).")
public String getAmtDispute() {
return amtDispute;
}
/**
* <strong>Format: </strong>Variable length, up to 3 AN<br><strong>Description: </strong>The ISO 4217 numeric currency code of the dispute.
* @return tranCurrency
**/
@ApiModelProperty(example = "840", required = true, value = "Format: Variable length, up to 3 AN
Description: The ISO 4217 numeric currency code of the dispute.")
public String getTranCurrency() {
return tranCurrency;
}
/**
* <strong>Format: </strong>Variable length, up to 25 AN<br><strong>Description: </strong>A merchant supplied tracking number, generally an invoice or purchase number. This number may be visible to the cardholder, depending on card issuer.
* @return purchaseId
**/
@ApiModelProperty(example = "1234567890", required = true, value = "Format: Variable length, up to 25 AN
Description: A merchant supplied tracking number, generally an invoice or purchase number. This number may be visible to the cardholder, depending on card issuer.")
public String getPurchaseId() {
return purchaseId;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>A merchant supplied tracking number which is stored by Qualpay, and does not pass to the card issuer.
* @return merchRefNum
**/
@ApiModelProperty(example = "1234567890", required = true, value = "Format: Variable length, up to 128 AN
Description: A merchant supplied tracking number which is stored by Qualpay, and does not pass to the card issuer.")
public String getMerchRefNum() {
return merchRefNum;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>If the transaction originated through the Qualpay Virtual Terminal, or Qualpay Payment Gateway, this is the tracking ID returned in the gateway response.
* @return pgId
**/
@ApiModelProperty(example = "7ea0ff0c9ba411e594f6063a1e626c0f", required = true, value = "Format: Fixed length, 32 AN
Description: If the transaction originated through the Qualpay Virtual Terminal, or Qualpay Payment Gateway, this is the tracking ID returned in the gateway response.")
public String getPgId() {
return pgId;
}
/**
* <strong>Format: </strong>Variable length, up to 6 AN<br><strong>Description: </strong>The authorization code provided by the card issuer when the card was approved.
* @return authCode
**/
@ApiModelProperty(example = "123456", required = true, value = "Format: Variable length, up to 6 AN
Description: The authorization code provided by the card issuer when the card was approved.")
public String getAuthCode() {
return authCode;
}
/**
* <strong>Format: </strong>Variable length, up to 8 AN<br><strong>Description: </strong>The card association reason why the dispute was issued.
* @return reasonCode
**/
@ApiModelProperty(example = "0083", required = true, value = "Format: Variable length, up to 8 AN
Description: The card association reason why the dispute was issued.")
public String getReasonCode() {
return reasonCode;
}
/**
* TODO
* @return recIdLinked
**/
@ApiModelProperty(example = "TODO", required = true, value = "TODO")
public String getRecIdLinked() {
return recIdLinked;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>The AVS (address validation service) match code of the original transaction.
* @return authAvsResult
**/
@ApiModelProperty(example = "M", required = true, value = "Format: Fixed length, 1 AN
Description: The AVS (address validation service) match code of the original transaction.")
public String getAuthAvsResult() {
return authAvsResult;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>The CVV2 match code of the original transaction.
* @return authCvv2Result
**/
@ApiModelProperty(example = "M", required = true, value = "Format: Fixed length, 1 AN
Description: The CVV2 match code of the original transaction.")
public String getAuthCvv2Result() {
return authCvv2Result;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>The truncated card number of the original captured transactions. In some rare cases a dispute may be issued on a different card than the original. This can occur when, for example, the card was was re-issued between the transaction date, and the dispute date.
* @return cardNumberOriginal
**/
@ApiModelProperty(example = "401288xxxxxx8882", required = true, value = "Format: Variable length, up to 16 AN
Description: The truncated card number of the original captured transactions. In some rare cases a dispute may be issued on a different card than the original. This can occur when, for example, the card was was re-issued between the transaction date, and the dispute date.")
public String getCardNumberOriginal() {
return cardNumberOriginal;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, in YYYY-MM-DD format<br><strong>Description: </strong>Records dispute status date. The dispute status of a dispute record will change over time and as a result of merchant actions.
* @return dateStatusChange
**/
@ApiModelProperty(example = "2016-08-01", value = "Format: Variable length, up to 10 AN, in YYYY-MM-DD format
Description: Records dispute status date. The dispute status of a dispute record will change over time and as a result of merchant actions.")
public String getDateStatusChange() {
return dateStatusChange;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DisputeReport disputeReport = (DisputeReport) o;
return Objects.equals(this.recId, disputeReport.recId) &&
Objects.equals(this.dataType, disputeReport.dataType) &&
Objects.equals(this.merchantId, disputeReport.merchantId) &&
Objects.equals(this.incomingDate, disputeReport.incomingDate) &&
Objects.equals(this.dbaName, disputeReport.dbaName) &&
Objects.equals(this.batchId, disputeReport.batchId) &&
Objects.equals(this.cardNumber, disputeReport.cardNumber) &&
Objects.equals(this.cardType, disputeReport.cardType) &&
Objects.equals(this.tranDate, disputeReport.tranDate) &&
Objects.equals(this.amtTran, disputeReport.amtTran) &&
Objects.equals(this.amtDispute, disputeReport.amtDispute) &&
Objects.equals(this.tranCurrency, disputeReport.tranCurrency) &&
Objects.equals(this.purchaseId, disputeReport.purchaseId) &&
Objects.equals(this.merchRefNum, disputeReport.merchRefNum) &&
Objects.equals(this.pgId, disputeReport.pgId) &&
Objects.equals(this.authCode, disputeReport.authCode) &&
Objects.equals(this.reasonCode, disputeReport.reasonCode) &&
Objects.equals(this.recIdLinked, disputeReport.recIdLinked) &&
Objects.equals(this.authAvsResult, disputeReport.authAvsResult) &&
Objects.equals(this.authCvv2Result, disputeReport.authCvv2Result) &&
Objects.equals(this.cardNumberOriginal, disputeReport.cardNumberOriginal) &&
Objects.equals(this.dateStatusChange, disputeReport.dateStatusChange);
}
@Override
public int hashCode() {
return Objects.hash(recId, dataType, merchantId, incomingDate, dbaName, batchId, cardNumber, cardType, tranDate, amtTran, amtDispute, tranCurrency, purchaseId, merchRefNum, pgId, authCode, reasonCode, recIdLinked, authAvsResult, authCvv2Result, cardNumberOriginal, dateStatusChange);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DisputeReport {\n");
sb.append(" recId: ").append(toIndentedString(recId)).append("\n");
sb.append(" dataType: ").append(toIndentedString(dataType)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" incomingDate: ").append(toIndentedString(incomingDate)).append("\n");
sb.append(" dbaName: ").append(toIndentedString(dbaName)).append("\n");
sb.append(" batchId: ").append(toIndentedString(batchId)).append("\n");
sb.append(" cardNumber: ").append(toIndentedString(cardNumber)).append("\n");
sb.append(" cardType: ").append(toIndentedString(cardType)).append("\n");
sb.append(" tranDate: ").append(toIndentedString(tranDate)).append("\n");
sb.append(" amtTran: ").append(toIndentedString(amtTran)).append("\n");
sb.append(" amtDispute: ").append(toIndentedString(amtDispute)).append("\n");
sb.append(" tranCurrency: ").append(toIndentedString(tranCurrency)).append("\n");
sb.append(" purchaseId: ").append(toIndentedString(purchaseId)).append("\n");
sb.append(" merchRefNum: ").append(toIndentedString(merchRefNum)).append("\n");
sb.append(" pgId: ").append(toIndentedString(pgId)).append("\n");
sb.append(" authCode: ").append(toIndentedString(authCode)).append("\n");
sb.append(" reasonCode: ").append(toIndentedString(reasonCode)).append("\n");
sb.append(" recIdLinked: ").append(toIndentedString(recIdLinked)).append("\n");
sb.append(" authAvsResult: ").append(toIndentedString(authAvsResult)).append("\n");
sb.append(" authCvv2Result: ").append(toIndentedString(authCvv2Result)).append("\n");
sb.append(" cardNumberOriginal: ").append(toIndentedString(cardNumberOriginal)).append("\n");
sb.append(" dateStatusChange: ").append(toIndentedString(dateStatusChange)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy