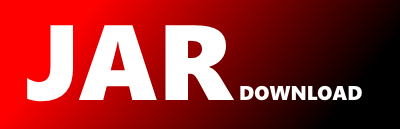
io.swagger.client.model.InvoicePayment Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* InvoicePayment
*/
public class InvoicePayment {
@SerializedName("payment_id")
private Long paymentId = null;
@SerializedName("merchant_id")
private Long merchantId = null;
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>The mode of payment. <br><ul><li>CARD Invoice checkout payment by customer using a card. A card payment cannot be manually added. </li><li>CASH Cash payment.</li><li>CHECK Check Payment.</li><li>OTHER Other modes of payment.</li></ul>
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
CARD("CARD"),
CASH("CASH"),
CHECK("CHECK"),
OTHER("OTHER");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String text) {
for (TypeEnum b : TypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("type")
private TypeEnum type = null;
@SerializedName("amt_paid")
private Double amtPaid = null;
@SerializedName("description")
private String description = null;
@SerializedName("pg_id")
private String pgId = null;
@SerializedName("invoice_id")
private Long invoiceId = null;
@SerializedName("date_payment")
private String datePayment = null;
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Status of an invoice. Possible values are <br><ul><li>SAVED Invoice is in draft state. An invoice in draft state can be updated. </li><li>OUTSTANDING Invoice has been mailed to the customer. Once an invoice is sent, only the billing_contact and from_contact can be updated. </li><li>PAID Invoice has been paid completely. A paid invoice cannot be updated. </li><li>CANCELED Invoice has been canceled. A canceled invoice cannot be updated. </li></ul>
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
SAVED("SAVED"),
OUTSTANDING("OUTSTANDING"),
PAID("PAID"),
CANCELED("CANCELED");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("status")
private StatusEnum status = null;
@SerializedName("tran_currency")
private String tranCurrency = null;
@SerializedName("tran_status")
private String tranStatus = null;
public InvoicePayment paymentId(Long paymentId) {
this.paymentId = paymentId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to an invoice payment. Save this id for managing invoice payments.
* @return paymentId
**/
@ApiModelProperty(example = "12457", value = "Format: Variable length, up to 10 N
Description: Unique ID assigned by Qualpay to an invoice payment. Save this id for managing invoice payments. ")
public Long getPaymentId() {
return paymentId;
}
public void setPaymentId(Long paymentId) {
this.paymentId = paymentId;
}
public InvoicePayment merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to a Merchant.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Format: Variable length, up to 16 N
Description: Unique ID assigned by Qualpay to a Merchant.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public InvoicePayment type(TypeEnum type) {
this.type = type;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>The mode of payment. <br><ul><li>CARD Invoice checkout payment by customer using a card. A card payment cannot be manually added. </li><li>CASH Cash payment.</li><li>CHECK Check Payment.</li><li>OTHER Other modes of payment.</li></ul>
* @return type
**/
@ApiModelProperty(example = "CASH", value = "Format: Variable length, up to 16 AN
Description: The mode of payment.
- CARD Invoice checkout payment by customer using a card. A card payment cannot be manually added.
- CASH Cash payment.
- CHECK Check Payment.
- OTHER Other modes of payment.
")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public InvoicePayment amtPaid(Double amtPaid) {
this.amtPaid = amtPaid;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>Amount paid.
* @return amtPaid
**/
@ApiModelProperty(example = "10.45", value = "Format: Variable length, up to 10,2 N
Description: Amount paid. ")
public Double getAmtPaid() {
return amtPaid;
}
public void setAmtPaid(Double amtPaid) {
this.amtPaid = amtPaid;
}
public InvoicePayment description(String description) {
this.description = description;
return this;
}
/**
* <strong>Format: </strong>Variable length AN<br><strong>Description: </strong>A short description of the payment.
* @return description
**/
@ApiModelProperty(example = "Check #3456", value = "Format: Variable length AN
Description: A short description of the payment.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public InvoicePayment pgId(String pgId) {
this.pgId = pgId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>Qualpay generated Payment gateway ID for the transaction. Applicable only to CARD type transaction.
* @return pgId
**/
@ApiModelProperty(example = "c0a3bead4a5911e6807e0a728c0d49c0", value = "Format: Fixed length, 32 AN
Description: Qualpay generated Payment gateway ID for the transaction. Applicable only to CARD type transaction.")
public String getPgId() {
return pgId;
}
public void setPgId(String pgId) {
this.pgId = pgId;
}
public InvoicePayment invoiceId(Long invoiceId) {
this.invoiceId = invoiceId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>A unique number generated by Qualpay to identify an invoice. Save this id to manage an invoice.
* @return invoiceId
**/
@ApiModelProperty(example = "1234", value = "Format: Variable length, up to 10 N
Description: A unique number generated by Qualpay to identify an invoice. Save this id to manage an invoice.")
public Long getInvoiceId() {
return invoiceId;
}
public void setInvoiceId(Long invoiceId) {
this.invoiceId = invoiceId;
}
public InvoicePayment datePayment(String datePayment) {
this.datePayment = datePayment;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 10 AN, in YYYY-MM-DD format<br><strong>Description: </strong>Date the payment was made
* @return datePayment
**/
@ApiModelProperty(example = "2018-01-01", value = "Format: Fixed length, 10 AN, in YYYY-MM-DD format
Description: Date the payment was made")
public String getDatePayment() {
return datePayment;
}
public void setDatePayment(String datePayment) {
this.datePayment = datePayment;
}
public InvoicePayment status(StatusEnum status) {
this.status = status;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Status of an invoice. Possible values are <br><ul><li>SAVED Invoice is in draft state. An invoice in draft state can be updated. </li><li>OUTSTANDING Invoice has been mailed to the customer. Once an invoice is sent, only the billing_contact and from_contact can be updated. </li><li>PAID Invoice has been paid completely. A paid invoice cannot be updated. </li><li>CANCELED Invoice has been canceled. A canceled invoice cannot be updated. </li></ul>
* @return status
**/
@ApiModelProperty(example = "PAID", value = "Format: Variable length, up to 16 AN
Description: Status of an invoice. Possible values are
- SAVED Invoice is in draft state. An invoice in draft state can be updated.
- OUTSTANDING Invoice has been mailed to the customer. Once an invoice is sent, only the billing_contact and from_contact can be updated.
- PAID Invoice has been paid completely. A paid invoice cannot be updated.
- CANCELED Invoice has been canceled. A canceled invoice cannot be updated.
")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public InvoicePayment tranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 3 AN<br><strong>Description: </strong>Numeric Currency Code. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of currency codes.
* @return tranCurrency
**/
@ApiModelProperty(example = "840", value = "Format: Variable length, up to 3 AN
Description: Numeric Currency Code. Refer to Country Codes for a list of currency codes. ")
public String getTranCurrency() {
return tranCurrency;
}
public void setTranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
}
public InvoicePayment tranStatus(String tranStatus) {
this.tranStatus = tranStatus;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>Transaction status for CARD payments. The status will be empty for non credit card payments.Possible values are <br><ul><li>A - Transaction is approved</li><li>H - Transaction Held</li><li>C - Transaction is captured</li><li>V - Transaction is voided by Merchant</li><li>v - Transaction is voided by System</li><li>K - Transaction is cancelled</li><li>S - Transaction Settled</li><li>P - Deposit Sent</li><li>N - Transaction Settled, but will not be funded by Qualpay</li><li>U - Transaction authorization failed or was declined by issuer</li><li>R - Transaction Rejected</li></ul>
* @return tranStatus
**/
@ApiModelProperty(example = "SETTLED", value = "Format: Fixed length, 1 AN
Description: Transaction status for CARD payments. The status will be empty for non credit card payments.Possible values are
- A - Transaction is approved
- H - Transaction Held
- C - Transaction is captured
- V - Transaction is voided by Merchant
- v - Transaction is voided by System
- K - Transaction is cancelled
- S - Transaction Settled
- P - Deposit Sent
- N - Transaction Settled, but will not be funded by Qualpay
- U - Transaction authorization failed or was declined by issuer
- R - Transaction Rejected
")
public String getTranStatus() {
return tranStatus;
}
public void setTranStatus(String tranStatus) {
this.tranStatus = tranStatus;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InvoicePayment invoicePayment = (InvoicePayment) o;
return Objects.equals(this.paymentId, invoicePayment.paymentId) &&
Objects.equals(this.merchantId, invoicePayment.merchantId) &&
Objects.equals(this.type, invoicePayment.type) &&
Objects.equals(this.amtPaid, invoicePayment.amtPaid) &&
Objects.equals(this.description, invoicePayment.description) &&
Objects.equals(this.pgId, invoicePayment.pgId) &&
Objects.equals(this.invoiceId, invoicePayment.invoiceId) &&
Objects.equals(this.datePayment, invoicePayment.datePayment) &&
Objects.equals(this.status, invoicePayment.status) &&
Objects.equals(this.tranCurrency, invoicePayment.tranCurrency) &&
Objects.equals(this.tranStatus, invoicePayment.tranStatus);
}
@Override
public int hashCode() {
return Objects.hash(paymentId, merchantId, type, amtPaid, description, pgId, invoiceId, datePayment, status, tranCurrency, tranStatus);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InvoicePayment {\n");
sb.append(" paymentId: ").append(toIndentedString(paymentId)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" amtPaid: ").append(toIndentedString(amtPaid)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" pgId: ").append(toIndentedString(pgId)).append("\n");
sb.append(" invoiceId: ").append(toIndentedString(invoiceId)).append("\n");
sb.append(" datePayment: ").append(toIndentedString(datePayment)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" tranCurrency: ").append(toIndentedString(tranCurrency)).append("\n");
sb.append(" tranStatus: ").append(toIndentedString(tranStatus)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy