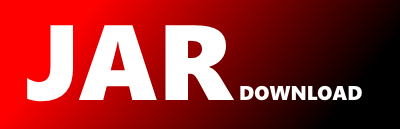
io.swagger.client.model.Settings Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.Payment;
import io.swagger.client.model.PaymentProfile;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Settings
*/
public class Settings {
@SerializedName("app_id")
private Long appId = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("dba_name")
private String dbaName = null;
@SerializedName("payments_accepted")
private List paymentsAccepted = null;
@SerializedName("payment_profiles")
private List paymentProfiles = null;
@SerializedName("user_login")
private String userLogin = null;
@SerializedName("reset_url")
private String resetUrl = null;
public Settings appId(Long appId) {
this.appId = appId;
return this;
}
/**
* The application ID for this merchant account. Only returned if an application exists.
* @return appId
**/
@ApiModelProperty(example = "501722", value = "The application ID for this merchant account. Only returned if an application exists.")
public Long getAppId() {
return appId;
}
public void setAppId(Long appId) {
this.appId = appId;
}
public Settings merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* The merchant ID this merchant account.
* @return merchantId
**/
@ApiModelProperty(example = "212000010001", value = "The merchant ID this merchant account.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public Settings dbaName(String dbaName) {
this.dbaName = dbaName;
return this;
}
/**
* The DBA name for the merchant account.
* @return dbaName
**/
@ApiModelProperty(example = "My Test Account", value = "The DBA name for the merchant account.")
public String getDbaName() {
return dbaName;
}
public void setDbaName(String dbaName) {
this.dbaName = dbaName;
}
public Settings paymentsAccepted(List paymentsAccepted) {
this.paymentsAccepted = paymentsAccepted;
return this;
}
public Settings addPaymentsAcceptedItem(Payment paymentsAcceptedItem) {
if (this.paymentsAccepted == null) {
this.paymentsAccepted = new ArrayList();
}
this.paymentsAccepted.add(paymentsAcceptedItem);
return this;
}
/**
* An array of the card types and currency accepted by the merchant account.
* @return paymentsAccepted
**/
@ApiModelProperty(value = "An array of the card types and currency accepted by the merchant account.")
public List getPaymentsAccepted() {
return paymentsAccepted;
}
public void setPaymentsAccepted(List paymentsAccepted) {
this.paymentsAccepted = paymentsAccepted;
}
public Settings paymentProfiles(List paymentProfiles) {
this.paymentProfiles = paymentProfiles;
return this;
}
public Settings addPaymentProfilesItem(PaymentProfile paymentProfilesItem) {
if (this.paymentProfiles == null) {
this.paymentProfiles = new ArrayList();
}
this.paymentProfiles.add(paymentProfilesItem);
return this;
}
/**
* An array payment profiles available for the merchant account.
* @return paymentProfiles
**/
@ApiModelProperty(value = "An array payment profiles available for the merchant account.")
public List getPaymentProfiles() {
return paymentProfiles;
}
public void setPaymentProfiles(List paymentProfiles) {
this.paymentProfiles = paymentProfiles;
}
public Settings userLogin(String userLogin) {
this.userLogin = userLogin;
return this;
}
/**
* The user login created for the merchant account. Only returned if a new user was requested during account creation.
* @return userLogin
**/
@ApiModelProperty(example = "testuser", value = "The user login created for the merchant account. Only returned if a new user was requested during account creation.")
public String getUserLogin() {
return userLogin;
}
public void setUserLogin(String userLogin) {
this.userLogin = userLogin;
}
public Settings resetUrl(String resetUrl) {
this.resetUrl = resetUrl;
return this;
}
/**
* The URL to establish a new password for the created user. Only returned if a new user was requested during account creation.
* @return resetUrl
**/
@ApiModelProperty(example = "https://app-test.qualpay.com/login/recover/b8b1e2cf5f7811e8bf8bacde48001122", value = "The URL to establish a new password for the created user. Only returned if a new user was requested during account creation.")
public String getResetUrl() {
return resetUrl;
}
public void setResetUrl(String resetUrl) {
this.resetUrl = resetUrl;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Settings settings = (Settings) o;
return Objects.equals(this.appId, settings.appId) &&
Objects.equals(this.merchantId, settings.merchantId) &&
Objects.equals(this.dbaName, settings.dbaName) &&
Objects.equals(this.paymentsAccepted, settings.paymentsAccepted) &&
Objects.equals(this.paymentProfiles, settings.paymentProfiles) &&
Objects.equals(this.userLogin, settings.userLogin) &&
Objects.equals(this.resetUrl, settings.resetUrl);
}
@Override
public int hashCode() {
return Objects.hash(appId, merchantId, dbaName, paymentsAccepted, paymentProfiles, userLogin, resetUrl);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Settings {\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" dbaName: ").append(toIndentedString(dbaName)).append("\n");
sb.append(" paymentsAccepted: ").append(toIndentedString(paymentsAccepted)).append("\n");
sb.append(" paymentProfiles: ").append(toIndentedString(paymentProfiles)).append("\n");
sb.append(" userLogin: ").append(toIndentedString(userLogin)).append("\n");
sb.append(" resetUrl: ").append(toIndentedString(resetUrl)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy