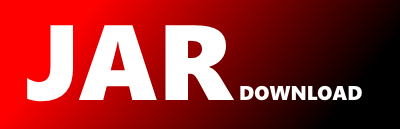
io.swagger.client.model.ShippingAddress Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* ShippingAddress
*/
public class ShippingAddress {
@SerializedName("shipping_id")
private Long shippingId = null;
@SerializedName("shipping_first_name")
private String shippingFirstName = null;
@SerializedName("shipping_last_name")
private String shippingLastName = null;
@SerializedName("shipping_firm_name")
private String shippingFirmName = null;
@SerializedName("shipping_addr1")
private String shippingAddr1 = null;
@SerializedName("shipping_addr2")
private String shippingAddr2 = null;
@SerializedName("shipping_city")
private String shippingCity = null;
@SerializedName("shipping_state")
private String shippingState = null;
@SerializedName("shipping_zip")
private String shippingZip = null;
@SerializedName("shipping_zip4")
private String shippingZip4 = null;
@SerializedName("shipping_country")
private String shippingCountry = null;
@SerializedName("shipping_country_code")
private String shippingCountryCode = null;
@SerializedName("primary")
private Boolean primary = null;
public ShippingAddress shippingId(Long shippingId) {
this.shippingId = shippingId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>Unique id generated by Qualpay to identify the shipping address for a customer.
* @return shippingId
**/
@ApiModelProperty(example = "12345", value = "Format: Variable length, up to 10 N
Description: Unique id generated by Qualpay to identify the shipping address for a customer.")
public Long getShippingId() {
return shippingId;
}
public void setShippingId(Long shippingId) {
this.shippingId = shippingId;
}
public ShippingAddress shippingFirstName(String shippingFirstName) {
this.shippingFirstName = shippingFirstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Shipping first name.
* @return shippingFirstName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: Shipping first name.")
public String getShippingFirstName() {
return shippingFirstName;
}
public void setShippingFirstName(String shippingFirstName) {
this.shippingFirstName = shippingFirstName;
}
public ShippingAddress shippingLastName(String shippingLastName) {
this.shippingLastName = shippingLastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Shipping last name.
* @return shippingLastName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: Shipping last name.")
public String getShippingLastName() {
return shippingLastName;
}
public void setShippingLastName(String shippingLastName) {
this.shippingLastName = shippingLastName;
}
public ShippingAddress shippingFirmName(String shippingFirmName) {
this.shippingFirmName = shippingFirmName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Shipping address business name, if applicable.
* @return shippingFirmName
**/
@ApiModelProperty(example = "Qualpay", value = "Format: Variable length, up to 64 AN
Description: Shipping address business name, if applicable. ")
public String getShippingFirmName() {
return shippingFirmName;
}
public void setShippingFirmName(String shippingFirmName) {
this.shippingFirmName = shippingFirmName;
}
public ShippingAddress shippingAddr1(String shippingAddr1) {
this.shippingAddr1 = shippingAddr1;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Shipping Address line item 1.
* @return shippingAddr1
**/
@ApiModelProperty(example = "123 Main Street", value = "Format: Variable length, up to 128 AN
Description: Shipping Address line item 1.")
public String getShippingAddr1() {
return shippingAddr1;
}
public void setShippingAddr1(String shippingAddr1) {
this.shippingAddr1 = shippingAddr1;
}
public ShippingAddress shippingAddr2(String shippingAddr2) {
this.shippingAddr2 = shippingAddr2;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Shipping Address line item 2.
* @return shippingAddr2
**/
@ApiModelProperty(example = "#1234", value = "Format: Variable length, up to 128 AN
Description: Shipping Address line item 2.")
public String getShippingAddr2() {
return shippingAddr2;
}
public void setShippingAddr2(String shippingAddr2) {
this.shippingAddr2 = shippingAddr2;
}
public ShippingAddress shippingCity(String shippingCity) {
this.shippingCity = shippingCity;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Shipping city.
* @return shippingCity
**/
@ApiModelProperty(example = "San Mateo", value = "Format: Variable length, up to 64 AN
Description: Shipping city.")
public String getShippingCity() {
return shippingCity;
}
public void setShippingCity(String shippingCity) {
this.shippingCity = shippingCity;
}
public ShippingAddress shippingState(String shippingState) {
this.shippingState = shippingState;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 3 AN<br><strong>Description: </strong>Shipping state.
* @return shippingState
**/
@ApiModelProperty(example = "CA", value = "Format: Variable length, up to 3 AN
Description: Shipping state.")
public String getShippingState() {
return shippingState;
}
public void setShippingState(String shippingState) {
this.shippingState = shippingState;
}
public ShippingAddress shippingZip(String shippingZip) {
this.shippingZip = shippingZip;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN<br><strong>Description: </strong>Shipping zip.
* @return shippingZip
**/
@ApiModelProperty(example = "94402", value = "Format: Variable length, up to 10 AN
Description: Shipping zip.")
public String getShippingZip() {
return shippingZip;
}
public void setShippingZip(String shippingZip) {
this.shippingZip = shippingZip;
}
public ShippingAddress shippingZip4(String shippingZip4) {
this.shippingZip4 = shippingZip4;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 4 N<br><strong>Description: </strong>Shipping zip+4 code, if applicable.
* @return shippingZip4
**/
@ApiModelProperty(example = "1234", value = "Format: Fixed length, 4 N
Description: Shipping zip+4 code, if applicable.")
public String getShippingZip4() {
return shippingZip4;
}
public void setShippingZip4(String shippingZip4) {
this.shippingZip4 = shippingZip4;
}
public ShippingAddress shippingCountry(String shippingCountry) {
this.shippingCountry = shippingCountry;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Shipping Country.
* @return shippingCountry
**/
@ApiModelProperty(example = "United States", value = "Format: Variable length, up to 128 AN
Description: Shipping Country.")
public String getShippingCountry() {
return shippingCountry;
}
public void setShippingCountry(String shippingCountry) {
this.shippingCountry = shippingCountry;
}
public ShippingAddress shippingCountryCode(String shippingCountryCode) {
this.shippingCountryCode = shippingCountryCode;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>ISO numeric country code for the shipping address. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of country codes.
* @return shippingCountryCode
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: ISO numeric country code for the shipping address. Refer to Country Codes for a list of country codes.")
public String getShippingCountryCode() {
return shippingCountryCode;
}
public void setShippingCountryCode(String shippingCountryCode) {
this.shippingCountryCode = shippingCountryCode;
}
public ShippingAddress primary(Boolean primary) {
this.primary = primary;
return this;
}
/**
* <br><strong>Description: </strong>If set to true, this is the default shipping address. <br><strong>Default: </strong>false
* @return primary
**/
@ApiModelProperty(example = "true", value = "
Description: If set to true, this is the default shipping address.
Default: false")
public Boolean isPrimary() {
return primary;
}
public void setPrimary(Boolean primary) {
this.primary = primary;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ShippingAddress shippingAddress = (ShippingAddress) o;
return Objects.equals(this.shippingId, shippingAddress.shippingId) &&
Objects.equals(this.shippingFirstName, shippingAddress.shippingFirstName) &&
Objects.equals(this.shippingLastName, shippingAddress.shippingLastName) &&
Objects.equals(this.shippingFirmName, shippingAddress.shippingFirmName) &&
Objects.equals(this.shippingAddr1, shippingAddress.shippingAddr1) &&
Objects.equals(this.shippingAddr2, shippingAddress.shippingAddr2) &&
Objects.equals(this.shippingCity, shippingAddress.shippingCity) &&
Objects.equals(this.shippingState, shippingAddress.shippingState) &&
Objects.equals(this.shippingZip, shippingAddress.shippingZip) &&
Objects.equals(this.shippingZip4, shippingAddress.shippingZip4) &&
Objects.equals(this.shippingCountry, shippingAddress.shippingCountry) &&
Objects.equals(this.shippingCountryCode, shippingAddress.shippingCountryCode) &&
Objects.equals(this.primary, shippingAddress.primary);
}
@Override
public int hashCode() {
return Objects.hash(shippingId, shippingFirstName, shippingLastName, shippingFirmName, shippingAddr1, shippingAddr2, shippingCity, shippingState, shippingZip, shippingZip4, shippingCountry, shippingCountryCode, primary);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ShippingAddress {\n");
sb.append(" shippingId: ").append(toIndentedString(shippingId)).append("\n");
sb.append(" shippingFirstName: ").append(toIndentedString(shippingFirstName)).append("\n");
sb.append(" shippingLastName: ").append(toIndentedString(shippingLastName)).append("\n");
sb.append(" shippingFirmName: ").append(toIndentedString(shippingFirmName)).append("\n");
sb.append(" shippingAddr1: ").append(toIndentedString(shippingAddr1)).append("\n");
sb.append(" shippingAddr2: ").append(toIndentedString(shippingAddr2)).append("\n");
sb.append(" shippingCity: ").append(toIndentedString(shippingCity)).append("\n");
sb.append(" shippingState: ").append(toIndentedString(shippingState)).append("\n");
sb.append(" shippingZip: ").append(toIndentedString(shippingZip)).append("\n");
sb.append(" shippingZip4: ").append(toIndentedString(shippingZip4)).append("\n");
sb.append(" shippingCountry: ").append(toIndentedString(shippingCountry)).append("\n");
sb.append(" shippingCountryCode: ").append(toIndentedString(shippingCountryCode)).append("\n");
sb.append(" primary: ").append(toIndentedString(primary)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy