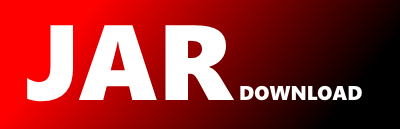
io.swagger.client.model.StatementReserveData Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.OffsetDateTime;
/**
* StatementReserveData
*/
public class StatementReserveData {
@SerializedName("rec_id_linked")
private Long recIdLinked = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("billing_month")
private String billingMonth = null;
@SerializedName("batch_date")
private OffsetDateTime batchDate = null;
@SerializedName("amt_Reserve")
private Double amtReserve = null;
@SerializedName("amt_released_reserve")
private Double amtReleasedReserve = null;
@SerializedName("amt_reserve_balance")
private Double amtReserveBalance = null;
public StatementReserveData recIdLinked(Long recIdLinked) {
this.recIdLinked = recIdLinked;
return this;
}
/**
* For INTERNAL USE ONLY.
* @return recIdLinked
**/
@ApiModelProperty(example = "0", value = "For INTERNAL USE ONLY.")
public Long getRecIdLinked() {
return recIdLinked;
}
public void setRecIdLinked(Long recIdLinked) {
this.recIdLinked = recIdLinked;
}
public StatementReserveData merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to a merchant.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Format: Variable length, up to 16 N
Description: Unique ID assigned by Qualpay to a merchant.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public StatementReserveData billingMonth(String billingMonth) {
this.billingMonth = billingMonth;
return this;
}
/**
* Identifies the date funds are posted to the depository account for either settled transactions, net of reserve or adjustments for release of reserves. in YYYY-MM-DD.
* @return billingMonth
**/
@ApiModelProperty(example = "2019-03-01", value = "Identifies the date funds are posted to the depository account for either settled transactions, net of reserve or adjustments for release of reserves. in YYYY-MM-DD.")
public String getBillingMonth() {
return billingMonth;
}
public void setBillingMonth(String billingMonth) {
this.billingMonth = billingMonth;
}
public StatementReserveData batchDate(OffsetDateTime batchDate) {
this.batchDate = batchDate;
return this;
}
/**
* Identifies the date funds are posted to the depository account for either settled transactions, net of reserve or adjustments for release of reserves. in YYYY-MM-DD.
* @return batchDate
**/
@ApiModelProperty(example = "2019-03-01", value = "Identifies the date funds are posted to the depository account for either settled transactions, net of reserve or adjustments for release of reserves. in YYYY-MM-DD.")
public OffsetDateTime getBatchDate() {
return batchDate;
}
public void setBatchDate(OffsetDateTime batchDate) {
this.batchDate = batchDate;
}
public StatementReserveData amtReserve(Double amtReserve) {
this.amtReserve = amtReserve;
return this;
}
/**
* Is the total dollar amount deducted from settled transactions prior to funding. The amount is calculated by applying an agreed upon percentage to the sales volume in a batch.
* @return amtReserve
**/
@ApiModelProperty(example = "10.1", value = "Is the total dollar amount deducted from settled transactions prior to funding. The amount is calculated by applying an agreed upon percentage to the sales volume in a batch.")
public Double getAmtReserve() {
return amtReserve;
}
public void setAmtReserve(Double amtReserve) {
this.amtReserve = amtReserve;
}
public StatementReserveData amtReleasedReserve(Double amtReleasedReserve) {
this.amtReleasedReserve = amtReleasedReserve;
return this;
}
/**
* Is the total dollar amount released as an adjustment to the depository account.
* @return amtReleasedReserve
**/
@ApiModelProperty(example = "10.1", value = "Is the total dollar amount released as an adjustment to the depository account.")
public Double getAmtReleasedReserve() {
return amtReleasedReserve;
}
public void setAmtReleasedReserve(Double amtReleasedReserve) {
this.amtReleasedReserve = amtReleasedReserve;
}
public StatementReserveData amtReserveBalance(Double amtReserveBalance) {
this.amtReserveBalance = amtReserveBalance;
return this;
}
/**
* Total amount of reserve held by Qualpay as of the ACH Post Date.
* @return amtReserveBalance
**/
@ApiModelProperty(example = "10.1", value = "Total amount of reserve held by Qualpay as of the ACH Post Date.")
public Double getAmtReserveBalance() {
return amtReserveBalance;
}
public void setAmtReserveBalance(Double amtReserveBalance) {
this.amtReserveBalance = amtReserveBalance;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
StatementReserveData statementReserveData = (StatementReserveData) o;
return Objects.equals(this.recIdLinked, statementReserveData.recIdLinked) &&
Objects.equals(this.merchantId, statementReserveData.merchantId) &&
Objects.equals(this.billingMonth, statementReserveData.billingMonth) &&
Objects.equals(this.batchDate, statementReserveData.batchDate) &&
Objects.equals(this.amtReserve, statementReserveData.amtReserve) &&
Objects.equals(this.amtReleasedReserve, statementReserveData.amtReleasedReserve) &&
Objects.equals(this.amtReserveBalance, statementReserveData.amtReserveBalance);
}
@Override
public int hashCode() {
return Objects.hash(recIdLinked, merchantId, billingMonth, batchDate, amtReserve, amtReleasedReserve, amtReserveBalance);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class StatementReserveData {\n");
sb.append(" recIdLinked: ").append(toIndentedString(recIdLinked)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" billingMonth: ").append(toIndentedString(billingMonth)).append("\n");
sb.append(" batchDate: ").append(toIndentedString(batchDate)).append("\n");
sb.append(" amtReserve: ").append(toIndentedString(amtReserve)).append("\n");
sb.append(" amtReleasedReserve: ").append(toIndentedString(amtReleasedReserve)).append("\n");
sb.append(" amtReserveBalance: ").append(toIndentedString(amtReserveBalance)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy