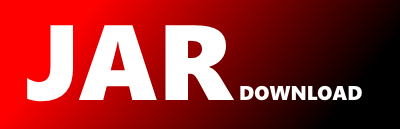
io.swagger.client.model.Subscription Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.CustomerVault;
import io.swagger.client.model.GatewayResponse;
import java.io.IOException;
import java.math.BigDecimal;
/**
* Subscription
*/
public class Subscription {
@SerializedName("subscription_id")
private Long subscriptionId = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("customer_id")
private String customerId = null;
@SerializedName("card_id")
private String cardId = null;
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong> Status of the subscription. Following are possible statuses: <ul> <li>A - Active</li> <li>D - Complete</li> <li>P - Paused</li> <li>C - Cancelled</li> <li>S - Suspended</li> </ul>
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
A("A"),
D("D"),
P("P"),
C("C"),
S("S");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("status")
private StatusEnum status = null;
@SerializedName("profile_id")
private String profileId = null;
@SerializedName("subscription_on_plan")
private Boolean subscriptionOnPlan = null;
@SerializedName("plan_id")
private Long planId = null;
@SerializedName("plan_name")
private String planName = null;
@SerializedName("plan_code")
private String planCode = null;
@SerializedName("plan_desc")
private String planDesc = null;
/**
* <strong>Format: </strong>Variable length, up to 1 N<br><strong>Description: </strong>This field identifies the frequency of billing. Use one of the following codes for frequency. <ul> <li>0 - Weekly</li> <li>1 - Bi-Weekly</li> <li>3 - Monthly</li> <li>4 - Quarterly</li> <li>5 - Bi-Annually</li> <li>6 - Annually</li> <li>7 - Daily</li> </ul>
*/
@JsonAdapter(PlanFrequencyEnum.Adapter.class)
public enum PlanFrequencyEnum {
NUMBER_0(0),
NUMBER_1(1),
NUMBER_3(3),
NUMBER_4(4),
NUMBER_5(5),
NUMBER_6(6),
NUMBER_7(7);
private Integer value;
PlanFrequencyEnum(Integer value) {
this.value = value;
}
public Integer getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PlanFrequencyEnum fromValue(String text) {
for (PlanFrequencyEnum b : PlanFrequencyEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PlanFrequencyEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PlanFrequencyEnum read(final JsonReader jsonReader) throws IOException {
Integer value = jsonReader.nextInt();
return PlanFrequencyEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("plan_frequency")
private PlanFrequencyEnum planFrequency = null;
@SerializedName("plan_duration")
private Integer planDuration = null;
@SerializedName("interval")
private Integer interval = null;
@SerializedName("customer_first_name")
private String customerFirstName = null;
@SerializedName("customer_last_name")
private String customerLastName = null;
@SerializedName("date_start")
private String dateStart = null;
@SerializedName("date_next")
private String dateNext = null;
@SerializedName("date_end")
private String dateEnd = null;
@SerializedName("amt_setup")
private BigDecimal amtSetup = null;
@SerializedName("prorate_date_start")
private String prorateDateStart = null;
@SerializedName("prorate_amt")
private BigDecimal prorateAmt = null;
@SerializedName("trial_date_start")
private String trialDateStart = null;
@SerializedName("trial_date_end")
private String trialDateEnd = null;
@SerializedName("trial_amt")
private BigDecimal trialAmt = null;
@SerializedName("recur_date_start")
private String recurDateStart = null;
@SerializedName("recur_date_end")
private String recurDateEnd = null;
@SerializedName("recur_amt")
private BigDecimal recurAmt = null;
@SerializedName("response")
private GatewayResponse response = null;
@SerializedName("customer")
private CustomerVault customer = null;
@SerializedName("tran_currency")
private String tranCurrency = null;
public Subscription subscriptionId(Long subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>Qualpay generated ID that identifies a subscription. Save this id to manage the subscription.
* @return subscriptionId
**/
@ApiModelProperty(example = "1111", value = "Format: Variable length, up to 10 N
Description: Qualpay generated ID that identifies a subscription. Save this id to manage the subscription. ")
public Long getSubscriptionId() {
return subscriptionId;
}
public void setSubscriptionId(Long subscriptionId) {
this.subscriptionId = subscriptionId;
}
public Subscription merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 N<br><strong>Description: </strong>Unique ID assigned by Qualpay to a merchant.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Format: Variable length, up to 16 N
Description: Unique ID assigned by Qualpay to a merchant.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public Subscription customerId(String customerId) {
this.customerId = customerId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Customer id of the subscriber.
* @return customerId
**/
@ApiModelProperty(example = "JOHNDOE", value = "Format: Variable length, up to 32 AN
Description: Customer id of the subscriber.")
public String getCustomerId() {
return customerId;
}
public void setCustomerId(String customerId) {
this.customerId = customerId;
}
public Subscription cardId(String cardId) {
this.cardId = cardId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>The payment method that will be used for the recurring charge. If empty, the recurring charges will be made using the customer's primary payment method.
* @return cardId
**/
@ApiModelProperty(example = "86e1b00d9b0811e68df3069d8f743581", value = "Format: Fixed length, 32 AN
Description: The payment method that will be used for the recurring charge. If empty, the recurring charges will be made using the customer's primary payment method. ")
public String getCardId() {
return cardId;
}
public void setCardId(String cardId) {
this.cardId = cardId;
}
public Subscription status(StatusEnum status) {
this.status = status;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong> Status of the subscription. Following are possible statuses: <ul> <li>A - Active</li> <li>D - Complete</li> <li>P - Paused</li> <li>C - Cancelled</li> <li>S - Suspended</li> </ul>
* @return status
**/
@ApiModelProperty(example = "A", value = "Format: Fixed length, 1 AN
Description: Status of the subscription. Following are possible statuses: - A - Active
- D - Complete
- P - Paused
- C - Cancelled
- S - Suspended
")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public Subscription profileId(String profileId) {
this.profileId = profileId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 20 AN<br><strong>Description: </strong>The profile ID to be used in payment gateway requests.
* @return profileId
**/
@ApiModelProperty(example = "21200000000100840", value = "Format: Variable length, up to 20 AN
Description: The profile ID to be used in payment gateway requests.")
public String getProfileId() {
return profileId;
}
public void setProfileId(String profileId) {
this.profileId = profileId;
}
public Subscription subscriptionOnPlan(Boolean subscriptionOnPlan) {
this.subscriptionOnPlan = subscriptionOnPlan;
return this;
}
/**
* <br><strong>Description: </strong>True for on-plan subscriptions, false for off-plan subscriptions.
* @return subscriptionOnPlan
**/
@ApiModelProperty(example = "true", value = "
Description: True for on-plan subscriptions, false for off-plan subscriptions.")
public Boolean isSubscriptionOnPlan() {
return subscriptionOnPlan;
}
public void setSubscriptionOnPlan(Boolean subscriptionOnPlan) {
this.subscriptionOnPlan = subscriptionOnPlan;
}
public Subscription planId(Long planId) {
this.planId = planId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 N<br><strong>Description: </strong>The plan id of the recurring plan associated with this subscription. 0 if this is an off-plan subscription.
* @return planId
**/
@ApiModelProperty(example = "1345", value = "Format: Variable length, up to 10 N
Description: The plan id of the recurring plan associated with this subscription. 0 if this is an off-plan subscription. ")
public Long getPlanId() {
return planId;
}
public void setPlanId(Long planId) {
this.planId = planId;
}
public Subscription planName(String planName) {
this.planName = planName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>A name assigned by merchant to the plan.
* @return planName
**/
@ApiModelProperty(example = "Qualpay Plan", value = "Format: Variable length, up to 64 AN
Description: A name assigned by merchant to the plan.")
public String getPlanName() {
return planName;
}
public void setPlanName(String planName) {
this.planName = planName;
}
public Subscription planCode(String planCode) {
this.planCode = planCode;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>A code assigned by merchant to the plan.
* @return planCode
**/
@ApiModelProperty(example = "SKU1234", value = "Format: Variable length, up to 16 AN
Description: A code assigned by merchant to the plan.")
public String getPlanCode() {
return planCode;
}
public void setPlanCode(String planCode) {
this.planCode = planCode;
}
public Subscription planDesc(String planDesc) {
this.planDesc = planDesc;
return this;
}
/**
* <strong>Format: </strong>Variable length AN<br><strong>Description: </strong>A short description of the plan.
* @return planDesc
**/
@ApiModelProperty(example = "ABC Monthly Billing Plan", value = "Format: Variable length AN
Description: A short description of the plan. ")
public String getPlanDesc() {
return planDesc;
}
public void setPlanDesc(String planDesc) {
this.planDesc = planDesc;
}
public Subscription planFrequency(PlanFrequencyEnum planFrequency) {
this.planFrequency = planFrequency;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 1 N<br><strong>Description: </strong>This field identifies the frequency of billing. Use one of the following codes for frequency. <ul> <li>0 - Weekly</li> <li>1 - Bi-Weekly</li> <li>3 - Monthly</li> <li>4 - Quarterly</li> <li>5 - Bi-Annually</li> <li>6 - Annually</li> <li>7 - Daily</li> </ul>
* @return planFrequency
**/
@ApiModelProperty(example = "0", value = "Format: Variable length, up to 1 N
Description: This field identifies the frequency of billing. Use one of the following codes for frequency. - 0 - Weekly
- 1 - Bi-Weekly
- 3 - Monthly
- 4 - Quarterly
- 5 - Bi-Annually
- 6 - Annually
- 7 - Daily
")
public PlanFrequencyEnum getPlanFrequency() {
return planFrequency;
}
public void setPlanFrequency(PlanFrequencyEnum planFrequency) {
this.planFrequency = planFrequency;
}
public Subscription planDuration(Integer planDuration) {
this.planDuration = planDuration;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 4 N<br><strong>Description: </strong> Number of billing cycles in the recurring transaction, -1 indicates bill until cancelled.
* @return planDuration
**/
@ApiModelProperty(example = "10", value = "Format: Variable length, up to 4 N
Description: Number of billing cycles in the recurring transaction, -1 indicates bill until cancelled.")
public Integer getPlanDuration() {
return planDuration;
}
public void setPlanDuration(Integer planDuration) {
this.planDuration = planDuration;
}
public Subscription interval(Integer interval) {
this.interval = interval;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 2 N<br><strong>Description: </strong> Applicable only for monthly frequency. Number of months in a subscription cycle.
* @return interval
**/
@ApiModelProperty(example = "3", value = "Format: Variable length, up to 2 N
Description: Applicable only for monthly frequency. Number of months in a subscription cycle.")
public Integer getInterval() {
return interval;
}
public void setInterval(Integer interval) {
this.interval = interval;
}
public Subscription customerFirstName(String customerFirstName) {
this.customerFirstName = customerFirstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>First name of the subscriber.
* @return customerFirstName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: First name of the subscriber.")
public String getCustomerFirstName() {
return customerFirstName;
}
public void setCustomerFirstName(String customerFirstName) {
this.customerFirstName = customerFirstName;
}
public Subscription customerLastName(String customerLastName) {
this.customerLastName = customerLastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Last name of the subscriber.
* @return customerLastName
**/
@ApiModelProperty(example = "Doe", value = "Format: Variable length, up to 32 AN
Description: Last name of the subscriber.")
public String getCustomerLastName() {
return customerLastName;
}
public void setCustomerLastName(String customerLastName) {
this.customerLastName = customerLastName;
}
public Subscription dateStart(String dateStart) {
this.dateStart = dateStart;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>Start date of subscription.
* @return dateStart
**/
@ApiModelProperty(example = "2016-07-14", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: Start date of subscription. ")
public String getDateStart() {
return dateStart;
}
public void setDateStart(String dateStart) {
this.dateStart = dateStart;
}
public Subscription dateNext(String dateNext) {
this.dateNext = dateNext;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>Next billing date of subscription. This field will be empty for cancelled and completed subscriptions.
* @return dateNext
**/
@ApiModelProperty(example = "2016-07-21", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: Next billing date of subscription. This field will be empty for cancelled and completed subscriptions.")
public String getDateNext() {
return dateNext;
}
public void setDateNext(String dateNext) {
this.dateNext = dateNext;
}
public Subscription dateEnd(String dateEnd) {
this.dateEnd = dateEnd;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>Date the subscription will end.
* @return dateEnd
**/
@ApiModelProperty(example = "2016-09-21", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: Date the subscription will end. ")
public String getDateEnd() {
return dateEnd;
}
public void setDateEnd(String dateEnd) {
this.dateEnd = dateEnd;
}
public Subscription amtSetup(BigDecimal amtSetup) {
this.amtSetup = amtSetup;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>One-time fee amount. This fee will be charged when a subscription is added.
* @return amtSetup
**/
@ApiModelProperty(example = "50.0", value = "Format: Variable length, up to 10,2 N
Description: One-time fee amount. This fee will be charged when a subscription is added.")
public BigDecimal getAmtSetup() {
return amtSetup;
}
public void setAmtSetup(BigDecimal amtSetup) {
this.amtSetup = amtSetup;
}
public Subscription prorateDateStart(String prorateDateStart) {
this.prorateDateStart = prorateDateStart;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>The date the customer will be billed the prorate amount. (for pro-rated subscriptions).
* @return prorateDateStart
**/
@ApiModelProperty(example = "2016-07-14", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: The date the customer will be billed the prorate amount. (for pro-rated subscriptions). ")
public String getProrateDateStart() {
return prorateDateStart;
}
public void setProrateDateStart(String prorateDateStart) {
this.prorateDateStart = prorateDateStart;
}
public Subscription prorateAmt(BigDecimal prorateAmt) {
this.prorateAmt = prorateAmt;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>The prorate amount (for pro-rated subscriptions).
* @return prorateAmt
**/
@ApiModelProperty(example = "11.13", value = "Format: Variable length, up to 10,2 N
Description: The prorate amount (for pro-rated subscriptions).")
public BigDecimal getProrateAmt() {
return prorateAmt;
}
public void setProrateAmt(BigDecimal prorateAmt) {
this.prorateAmt = prorateAmt;
}
public Subscription trialDateStart(String trialDateStart) {
this.trialDateStart = trialDateStart;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>The start date of the trial period. Applicable only for subscriptions that include a trail period.
* @return trialDateStart
**/
@ApiModelProperty(example = "2016-07-20", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: The start date of the trial period. Applicable only for subscriptions that include a trail period.")
public String getTrialDateStart() {
return trialDateStart;
}
public void setTrialDateStart(String trialDateStart) {
this.trialDateStart = trialDateStart;
}
public Subscription trialDateEnd(String trialDateEnd) {
this.trialDateEnd = trialDateEnd;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>The end date of the trial period. Applicable only for subscriptions that include a trail period.
* @return trialDateEnd
**/
@ApiModelProperty(example = "2016-07-20", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: The end date of the trial period. Applicable only for subscriptions that include a trail period.")
public String getTrialDateEnd() {
return trialDateEnd;
}
public void setTrialDateEnd(String trialDateEnd) {
this.trialDateEnd = trialDateEnd;
}
public Subscription trialAmt(BigDecimal trialAmt) {
this.trialAmt = trialAmt;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>The amount billed during the trial period. Applicable only for subscriptions that include a trail period.
* @return trialAmt
**/
@ApiModelProperty(example = "10.45", value = "Format: Variable length, up to 10,2 N
Description: The amount billed during the trial period. Applicable only for subscriptions that include a trail period.")
public BigDecimal getTrialAmt() {
return trialAmt;
}
public void setTrialAmt(BigDecimal trialAmt) {
this.trialAmt = trialAmt;
}
public Subscription recurDateStart(String recurDateStart) {
this.recurDateStart = recurDateStart;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>Date regular billing cycle will start.
* @return recurDateStart
**/
@ApiModelProperty(example = "2016-07-30", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: Date regular billing cycle will start.")
public String getRecurDateStart() {
return recurDateStart;
}
public void setRecurDateStart(String recurDateStart) {
this.recurDateStart = recurDateStart;
}
public Subscription recurDateEnd(String recurDateEnd) {
this.recurDateEnd = recurDateEnd;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>Date regular billing cycle will end.
* @return recurDateEnd
**/
@ApiModelProperty(example = "2016-12-30", value = "Format: Variable length, up to 10 AN, YYYY-MM-DD format
Description: Date regular billing cycle will end. ")
public String getRecurDateEnd() {
return recurDateEnd;
}
public void setRecurDateEnd(String recurDateEnd) {
this.recurDateEnd = recurDateEnd;
}
public Subscription recurAmt(BigDecimal recurAmt) {
this.recurAmt = recurAmt;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>Regular billing amount.
* @return recurAmt
**/
@ApiModelProperty(example = "25.99", value = "Format: Variable length, up to 10,2 N
Description: Regular billing amount.")
public BigDecimal getRecurAmt() {
return recurAmt;
}
public void setRecurAmt(BigDecimal recurAmt) {
this.recurAmt = recurAmt;
}
public Subscription response(GatewayResponse response) {
this.response = response;
return this;
}
/**
* <br><strong>Description: </strong>Response from payment gateway for amt_setup fee. Applicable only when adding subscriptions with one time fee.
* @return response
**/
@ApiModelProperty(value = "
Description: Response from payment gateway for amt_setup fee. Applicable only when adding subscriptions with one time fee. ")
public GatewayResponse getResponse() {
return response;
}
public void setResponse(GatewayResponse response) {
this.response = response;
}
public Subscription customer(CustomerVault customer) {
this.customer = customer;
return this;
}
/**
* <br><strong>Description: </strong>Customer vault reord. Applicable only when adding subscriptions.
* @return customer
**/
@ApiModelProperty(value = "
Description: Customer vault reord. Applicable only when adding subscriptions. ")
public CustomerVault getCustomer() {
return customer;
}
public void setCustomer(CustomerVault customer) {
this.customer = customer;
}
public Subscription tranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>Numeric Currency Code. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for possible values.<br><strong>Default: </strong>840
* @return tranCurrency
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: Numeric Currency Code. Refer to Country Codes for possible values.
Default: 840")
public String getTranCurrency() {
return tranCurrency;
}
public void setTranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Subscription subscription = (Subscription) o;
return Objects.equals(this.subscriptionId, subscription.subscriptionId) &&
Objects.equals(this.merchantId, subscription.merchantId) &&
Objects.equals(this.customerId, subscription.customerId) &&
Objects.equals(this.cardId, subscription.cardId) &&
Objects.equals(this.status, subscription.status) &&
Objects.equals(this.profileId, subscription.profileId) &&
Objects.equals(this.subscriptionOnPlan, subscription.subscriptionOnPlan) &&
Objects.equals(this.planId, subscription.planId) &&
Objects.equals(this.planName, subscription.planName) &&
Objects.equals(this.planCode, subscription.planCode) &&
Objects.equals(this.planDesc, subscription.planDesc) &&
Objects.equals(this.planFrequency, subscription.planFrequency) &&
Objects.equals(this.planDuration, subscription.planDuration) &&
Objects.equals(this.interval, subscription.interval) &&
Objects.equals(this.customerFirstName, subscription.customerFirstName) &&
Objects.equals(this.customerLastName, subscription.customerLastName) &&
Objects.equals(this.dateStart, subscription.dateStart) &&
Objects.equals(this.dateNext, subscription.dateNext) &&
Objects.equals(this.dateEnd, subscription.dateEnd) &&
Objects.equals(this.amtSetup, subscription.amtSetup) &&
Objects.equals(this.prorateDateStart, subscription.prorateDateStart) &&
Objects.equals(this.prorateAmt, subscription.prorateAmt) &&
Objects.equals(this.trialDateStart, subscription.trialDateStart) &&
Objects.equals(this.trialDateEnd, subscription.trialDateEnd) &&
Objects.equals(this.trialAmt, subscription.trialAmt) &&
Objects.equals(this.recurDateStart, subscription.recurDateStart) &&
Objects.equals(this.recurDateEnd, subscription.recurDateEnd) &&
Objects.equals(this.recurAmt, subscription.recurAmt) &&
Objects.equals(this.response, subscription.response) &&
Objects.equals(this.customer, subscription.customer) &&
Objects.equals(this.tranCurrency, subscription.tranCurrency);
}
@Override
public int hashCode() {
return Objects.hash(subscriptionId, merchantId, customerId, cardId, status, profileId, subscriptionOnPlan, planId, planName, planCode, planDesc, planFrequency, planDuration, interval, customerFirstName, customerLastName, dateStart, dateNext, dateEnd, amtSetup, prorateDateStart, prorateAmt, trialDateStart, trialDateEnd, trialAmt, recurDateStart, recurDateEnd, recurAmt, response, customer, tranCurrency);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Subscription {\n");
sb.append(" subscriptionId: ").append(toIndentedString(subscriptionId)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" customerId: ").append(toIndentedString(customerId)).append("\n");
sb.append(" cardId: ").append(toIndentedString(cardId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" profileId: ").append(toIndentedString(profileId)).append("\n");
sb.append(" subscriptionOnPlan: ").append(toIndentedString(subscriptionOnPlan)).append("\n");
sb.append(" planId: ").append(toIndentedString(planId)).append("\n");
sb.append(" planName: ").append(toIndentedString(planName)).append("\n");
sb.append(" planCode: ").append(toIndentedString(planCode)).append("\n");
sb.append(" planDesc: ").append(toIndentedString(planDesc)).append("\n");
sb.append(" planFrequency: ").append(toIndentedString(planFrequency)).append("\n");
sb.append(" planDuration: ").append(toIndentedString(planDuration)).append("\n");
sb.append(" interval: ").append(toIndentedString(interval)).append("\n");
sb.append(" customerFirstName: ").append(toIndentedString(customerFirstName)).append("\n");
sb.append(" customerLastName: ").append(toIndentedString(customerLastName)).append("\n");
sb.append(" dateStart: ").append(toIndentedString(dateStart)).append("\n");
sb.append(" dateNext: ").append(toIndentedString(dateNext)).append("\n");
sb.append(" dateEnd: ").append(toIndentedString(dateEnd)).append("\n");
sb.append(" amtSetup: ").append(toIndentedString(amtSetup)).append("\n");
sb.append(" prorateDateStart: ").append(toIndentedString(prorateDateStart)).append("\n");
sb.append(" prorateAmt: ").append(toIndentedString(prorateAmt)).append("\n");
sb.append(" trialDateStart: ").append(toIndentedString(trialDateStart)).append("\n");
sb.append(" trialDateEnd: ").append(toIndentedString(trialDateEnd)).append("\n");
sb.append(" trialAmt: ").append(toIndentedString(trialAmt)).append("\n");
sb.append(" recurDateStart: ").append(toIndentedString(recurDateStart)).append("\n");
sb.append(" recurDateEnd: ").append(toIndentedString(recurDateEnd)).append("\n");
sb.append(" recurAmt: ").append(toIndentedString(recurAmt)).append("\n");
sb.append(" response: ").append(toIndentedString(response)).append("\n");
sb.append(" customer: ").append(toIndentedString(customer)).append("\n");
sb.append(" tranCurrency: ").append(toIndentedString(tranCurrency)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy