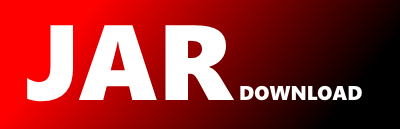
io.swagger.client.model.TransactionRequestReport Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* TransactionRequestReport
*/
public class TransactionRequestReport {
@SerializedName("qp_id")
private Long qpId = null;
@SerializedName("merchant_id")
private Long merchantId = null;
@SerializedName("tran_time")
private String tranTime = null;
@SerializedName("tran_date")
private String tranDate = null;
@SerializedName("request_type")
private String requestType = null;
@SerializedName("tran_status")
private String tranStatus = null;
@SerializedName("dispute_flag")
private Boolean disputeFlag = null;
@SerializedName("amt_refunded")
private Double amtRefunded = null;
@SerializedName("pg_id")
private String pgId = null;
@SerializedName("pg_id_linked")
private String pgIdLinked = null;
@SerializedName("amt_tran")
private Double amtTran = null;
@SerializedName("tran_currency")
private String tranCurrency = null;
@SerializedName("purchase_id")
private String purchaseId = null;
@SerializedName("cardholder_first_name")
private String cardholderFirstName = null;
@SerializedName("cardholder_last_name")
private String cardholderLastName = null;
@SerializedName("card_number")
private String cardNumber = null;
/**
* <strong>Format: </strong>Fixed length, 2 AN<br><strong>Description: </strong>Card type of the billing card used for the transaction. Refer to <a href=\"/developer/api/reference#card-types\"target=\"_blank\">Card Types</a> for possible values.
*/
@JsonAdapter(CardTypeEnum.Adapter.class)
public enum CardTypeEnum {
VS("VS"),
MC("MC"),
AM("AM"),
DS("DS"),
JC("JC"),
PP("PP"),
AP("AP");
private String value;
CardTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static CardTypeEnum fromValue(String text) {
for (CardTypeEnum b : CardTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final CardTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public CardTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return CardTypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("card_type")
private CardTypeEnum cardType = null;
@SerializedName("auth_code")
private String authCode = null;
@SerializedName("acq_reference_number")
private String acqReferenceNumber = null;
@SerializedName("merch_ref_num")
private String merchRefNum = null;
@SerializedName("amt_convenience_fee")
private Double amtConvenienceFee = null;
@SerializedName("amt_tran_fee")
private Double amtTranFee = null;
@SerializedName("dba_name")
private String dbaName = null;
@SerializedName("amt_funded")
private Double amtFunded = null;
@SerializedName("funded_currency")
private String fundedCurrency = null;
@SerializedName("rcode")
private String rcode = null;
@SerializedName("auth_tran_id")
private String authTranId = null;
@SerializedName("auth_avs_result")
private String authAvsResult = null;
@SerializedName("auth_cvv2_result")
private String authCvv2Result = null;
public TransactionRequestReport qpId(Long qpId) {
this.qpId = qpId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 10 N<br><strong>Description: </strong>An internal number generated by qualpay to identify a transaction. Requests belonging to the same transaction will have the same qp_id.
* @return qpId
**/
@ApiModelProperty(example = "56789", value = "Format: Fixed length, 10 N
Description: An internal number generated by qualpay to identify a transaction. Requests belonging to the same transaction will have the same qp_id. ")
public Long getQpId() {
return qpId;
}
public void setQpId(Long qpId) {
this.qpId = qpId;
}
public TransactionRequestReport merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Identifies the merchant to which this transaction belongs.
* @return merchantId
**/
@ApiModelProperty(example = "212000000001", value = "Format: Variable length, up to 16 AN
Description: Identifies the merchant to which this transaction belongs.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
public TransactionRequestReport tranTime(String tranTime) {
this.tranTime = tranTime;
return this;
}
/**
* <strong>Format: </strong>Variable length, in YYYY-MM-DD HH:MM:ss format<br><strong>Description: </strong>Transaction time. All times are Pacific time.
* @return tranTime
**/
@ApiModelProperty(example = "2016-07-01 00:00:03", value = "Format: Variable length, in YYYY-MM-DD HH:MM:ss format
Description: Transaction time. All times are Pacific time. ")
public String getTranTime() {
return tranTime;
}
public void setTranTime(String tranTime) {
this.tranTime = tranTime;
}
public TransactionRequestReport tranDate(String tranDate) {
this.tranDate = tranDate;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 10 AN, YYYY-MM-DD format<br><strong>Description: </strong>The date the transaction was captured by the merchant.
* @return tranDate
**/
@ApiModelProperty(example = "2016-07-01", value = "Format: Fixed length, 10 AN, YYYY-MM-DD format
Description: The date the transaction was captured by the merchant.")
public String getTranDate() {
return tranDate;
}
public void setTranDate(String tranDate) {
this.tranDate = tranDate;
}
public TransactionRequestReport requestType(String requestType) {
this.requestType = requestType;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>The request type of the transaction.
* @return requestType
**/
@ApiModelProperty(example = "sale", value = "Format: Variable length, up to 32 AN
Description: The request type of the transaction.")
public String getRequestType() {
return requestType;
}
public void setRequestType(String requestType) {
this.requestType = requestType;
}
public TransactionRequestReport tranStatus(String tranStatus) {
this.tranStatus = tranStatus;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>Transaction status.<ul><li>A - Transaction is approved</li><li>H - Transaction Held</li><li>C - Transaction is captured</li><li>V - Transaction is voided by Merchant</li><li>v - Transaction is voided by System</li><li>K - Transaction is cancelled</li><li>D - Transaction is declined by issuer</li><li>F - Transaction failures other than Issuer Declines</li><li>S - Transaction Settled</li><li>P - Deposit Sent</li><li>N - Transaction Settled, but will not be funded by Qualpay</li><li>R - Transaction Rejected</li></ul>
* @return tranStatus
**/
@ApiModelProperty(example = "C", value = "Format: Fixed length, 1 AN
Description: Transaction status.- A - Transaction is approved
- H - Transaction Held
- C - Transaction is captured
- V - Transaction is voided by Merchant
- v - Transaction is voided by System
- K - Transaction is cancelled
- D - Transaction is declined by issuer
- F - Transaction failures other than Issuer Declines
- S - Transaction Settled
- P - Deposit Sent
- N - Transaction Settled, but will not be funded by Qualpay
- R - Transaction Rejected
")
public String getTranStatus() {
return tranStatus;
}
public void setTranStatus(String tranStatus) {
this.tranStatus = tranStatus;
}
public TransactionRequestReport disputeFlag(Boolean disputeFlag) {
this.disputeFlag = disputeFlag;
return this;
}
/**
* <br><strong>Description: </strong>Will be set to true if the transaction is disputed.
* @return disputeFlag
**/
@ApiModelProperty(example = "false", value = "
Description: Will be set to true if the transaction is disputed.")
public Boolean isDisputeFlag() {
return disputeFlag;
}
public void setDisputeFlag(Boolean disputeFlag) {
this.disputeFlag = disputeFlag;
}
public TransactionRequestReport amtRefunded(Double amtRefunded) {
this.amtRefunded = amtRefunded;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 12,2 N<br><strong>Description: </strong>Amount refunded if there are any refunds.
* @return amtRefunded
**/
@ApiModelProperty(example = "0.0", value = "Format: Variable length, up to 12,2 N
Description: Amount refunded if there are any refunds.")
public Double getAmtRefunded() {
return amtRefunded;
}
public void setAmtRefunded(Double amtRefunded) {
this.amtRefunded = amtRefunded;
}
public TransactionRequestReport pgId(String pgId) {
this.pgId = pgId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>Qualpay generated payment gateway ID for the transaction.
* @return pgId
**/
@ApiModelProperty(example = "c0a3bead4a5911e6807e0a728c0d49c0", value = "Format: Fixed length, 32 AN
Description: Qualpay generated payment gateway ID for the transaction.")
public String getPgId() {
return pgId;
}
public void setPgId(String pgId) {
this.pgId = pgId;
}
public TransactionRequestReport pgIdLinked(String pgIdLinked) {
this.pgIdLinked = pgIdLinked;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>If this is a refund request, this field will provided the payment gateway ID of the original transaction.
* @return pgIdLinked
**/
@ApiModelProperty(example = "d123abead4a5911e6807e0a728c0d49c0", value = "Format: Fixed length, 32 AN
Description: If this is a refund request, this field will provided the payment gateway ID of the original transaction.")
public String getPgIdLinked() {
return pgIdLinked;
}
public void setPgIdLinked(String pgIdLinked) {
this.pgIdLinked = pgIdLinked;
}
public TransactionRequestReport amtTran(Double amtTran) {
this.amtTran = amtTran;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 12,2 N<br><strong>Description: </strong>Transaction amount.
* @return amtTran
**/
@ApiModelProperty(example = "10.99", value = "Format: Variable length, up to 12,2 N
Description: Transaction amount.")
public Double getAmtTran() {
return amtTran;
}
public void setAmtTran(Double amtTran) {
this.amtTran = amtTran;
}
public TransactionRequestReport tranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>Numeric currency code of the transaction. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of currency codes.
* @return tranCurrency
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: Numeric currency code of the transaction. Refer to Country Codes for a list of currency codes. ")
public String getTranCurrency() {
return tranCurrency;
}
public void setTranCurrency(String tranCurrency) {
this.tranCurrency = tranCurrency;
}
public TransactionRequestReport purchaseId(String purchaseId) {
this.purchaseId = purchaseId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 25 AN<br><strong>Description: </strong>Purchase ID of the transaction.
* @return purchaseId
**/
@ApiModelProperty(example = "QP12345", value = "Format: Variable length, up to 25 AN
Description: Purchase ID of the transaction.")
public String getPurchaseId() {
return purchaseId;
}
public void setPurchaseId(String purchaseId) {
this.purchaseId = purchaseId;
}
public TransactionRequestReport cardholderFirstName(String cardholderFirstName) {
this.cardholderFirstName = cardholderFirstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>First name of card holder.
* @return cardholderFirstName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: First name of card holder.")
public String getCardholderFirstName() {
return cardholderFirstName;
}
public void setCardholderFirstName(String cardholderFirstName) {
this.cardholderFirstName = cardholderFirstName;
}
public TransactionRequestReport cardholderLastName(String cardholderLastName) {
this.cardholderLastName = cardholderLastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Last name of card holder.
* @return cardholderLastName
**/
@ApiModelProperty(example = "Doe", value = "Format: Variable length, up to 32 AN
Description: Last name of card holder.")
public String getCardholderLastName() {
return cardholderLastName;
}
public void setCardholderLastName(String cardholderLastName) {
this.cardholderLastName = cardholderLastName;
}
public TransactionRequestReport cardNumber(String cardNumber) {
this.cardNumber = cardNumber;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 16 AN<br><strong>Description: </strong>Masked card number.
* @return cardNumber
**/
@ApiModelProperty(example = "488888xxxxxx8887", value = "Format: Fixed length, 16 AN
Description: Masked card number. ")
public String getCardNumber() {
return cardNumber;
}
public void setCardNumber(String cardNumber) {
this.cardNumber = cardNumber;
}
public TransactionRequestReport cardType(CardTypeEnum cardType) {
this.cardType = cardType;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 2 AN<br><strong>Description: </strong>Card type of the billing card used for the transaction. Refer to <a href=\"/developer/api/reference#card-types\"target=\"_blank\">Card Types</a> for possible values.
* @return cardType
**/
@ApiModelProperty(example = "VS", value = "Format: Fixed length, 2 AN
Description: Card type of the billing card used for the transaction. Refer to Card Types for possible values. ")
public CardTypeEnum getCardType() {
return cardType;
}
public void setCardType(CardTypeEnum cardType) {
this.cardType = cardType;
}
public TransactionRequestReport authCode(String authCode) {
this.authCode = authCode;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 6 AN<br><strong>Description: </strong>Authorization code from issuer.
* @return authCode
**/
@ApiModelProperty(example = "T12345", value = "Format: Variable length, up to 6 AN
Description: Authorization code from issuer.")
public String getAuthCode() {
return authCode;
}
public void setAuthCode(String authCode) {
this.authCode = authCode;
}
/**
* <strong>Format: </strong>Variable length, up to 11 N<br><strong>Description: </strong>Acquirer reference number is an 11-digit number generated by the product initiating the transaction. It is a unique number that both the acquirer and the issuer can use to identify a transaction. For chargeback adjustments, the acquirer reference number is used as the deposit reference number.
* @return acqReferenceNumber
**/
@ApiModelProperty(example = "8975679", value = "Format: Variable length, up to 11 N
Description: Acquirer reference number is an 11-digit number generated by the product initiating the transaction. It is a unique number that both the acquirer and the issuer can use to identify a transaction. For chargeback adjustments, the acquirer reference number is used as the deposit reference number.")
public String getAcqReferenceNumber() {
return acqReferenceNumber;
}
public TransactionRequestReport merchRefNum(String merchRefNum) {
this.merchRefNum = merchRefNum;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Merchant provided reference number for this transaction.
* @return merchRefNum
**/
@ApiModelProperty(example = "Ref#1234", value = "Format: Variable length, up to 128 AN
Description: Merchant provided reference number for this transaction.")
public String getMerchRefNum() {
return merchRefNum;
}
public void setMerchRefNum(String merchRefNum) {
this.merchRefNum = merchRefNum;
}
public TransactionRequestReport amtConvenienceFee(Double amtConvenienceFee) {
this.amtConvenienceFee = amtConvenienceFee;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 8,2 N<br><strong>Description: </strong>Amount of convenience fee. A convenience fee is a fee charged to your customer for the \"convenience\" of being able to pay using an alternative payment channel outside your merchant's customary payment channel.
* @return amtConvenienceFee
**/
@ApiModelProperty(example = "2.0", value = "Format: Variable length, up to 8,2 N
Description: Amount of convenience fee. A convenience fee is a fee charged to your customer for the \"convenience\" of being able to pay using an alternative payment channel outside your merchant's customary payment channel. ")
public Double getAmtConvenienceFee() {
return amtConvenienceFee;
}
public void setAmtConvenienceFee(Double amtConvenienceFee) {
this.amtConvenienceFee = amtConvenienceFee;
}
public TransactionRequestReport amtTranFee(Double amtTranFee) {
this.amtTranFee = amtTranFee;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 8,2 N<br><strong>Description: </strong>Amount of transaction surcharge fee. A surcharge is a fee added to the cost of a purchase for the \"privilege\" of using a credit card instead of another form of payment,
* @return amtTranFee
**/
@ApiModelProperty(example = "2.35", value = "Format: Variable length, up to 8,2 N
Description: Amount of transaction surcharge fee. A surcharge is a fee added to the cost of a purchase for the \"privilege\" of using a credit card instead of another form of payment, ")
public Double getAmtTranFee() {
return amtTranFee;
}
public void setAmtTranFee(Double amtTranFee) {
this.amtTranFee = amtTranFee;
}
public TransactionRequestReport dbaName(String dbaName) {
this.dbaName = dbaName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 25 AN<br><strong>Description: </strong>DBA name used in the transaction.
* @return dbaName
**/
@ApiModelProperty(example = "Qualpay", value = "Format: Variable length, up to 25 AN
Description: DBA name used in the transaction.")
public String getDbaName() {
return dbaName;
}
public void setDbaName(String dbaName) {
this.dbaName = dbaName;
}
public TransactionRequestReport amtFunded(Double amtFunded) {
this.amtFunded = amtFunded;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10,2 N<br><strong>Description: </strong>The amount funded to the merchant (in funded currency).
* @return amtFunded
**/
@ApiModelProperty(example = "100.0", value = "Format: Variable length, up to 10,2 N
Description: The amount funded to the merchant (in funded currency).")
public Double getAmtFunded() {
return amtFunded;
}
public void setAmtFunded(Double amtFunded) {
this.amtFunded = amtFunded;
}
public TransactionRequestReport fundedCurrency(String fundedCurrency) {
this.fundedCurrency = fundedCurrency;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>Numeric currency code of the funded amount. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for possible values.
* @return fundedCurrency
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: Numeric currency code of the funded amount. Refer to Country Codes for possible values. ")
public String getFundedCurrency() {
return fundedCurrency;
}
public void setFundedCurrency(String fundedCurrency) {
this.fundedCurrency = fundedCurrency;
}
public TransactionRequestReport rcode(String rcode) {
this.rcode = rcode;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>Gateway response code. Refer to <a href=\"/developer/api/reference#gateway-response-codes\"target=\"_blank\">Payment Gateway Response Codes</a> for possible values.
* @return rcode
**/
@ApiModelProperty(example = "000", value = "Format: Fixed length, 3 AN
Description: Gateway response code. Refer to Payment Gateway Response Codes for possible values.")
public String getRcode() {
return rcode;
}
public void setRcode(String rcode) {
this.rcode = rcode;
}
/**
* <strong>Format: </strong>Variable length, up to 15 AN<br><strong>Description: </strong>The Authorization transaction identifier is a unique identifier returned by the issuing bank for an electronically authorized transaction.
* @return authTranId
**/
@ApiModelProperty(example = "9902KUUSCJCP5I", value = "Format: Variable length, up to 15 AN
Description: The Authorization transaction identifier is a unique identifier returned by the issuing bank for an electronically authorized transaction.")
public String getAuthTranId() {
return authTranId;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>AVS result from card issuer (if avs_zip and optionally avs_address were provided in the request). Refer to <a href=\"/developer/api/reference#avs-result-codes\"target=\"_blank\">Payment Result Codes for AVS</a> for possible values.
* @return authAvsResult
**/
@ApiModelProperty(example = "X", value = "Format: Fixed length, 1 AN
Description: AVS result from card issuer (if avs_zip and optionally avs_address were provided in the request). Refer to Payment Result Codes for AVS for possible values.")
public String getAuthAvsResult() {
return authAvsResult;
}
/**
* <strong>Format: </strong>Fixed length, 1 AN<br><strong>Description: </strong>CVV2 result from card issuer (if CVV2 data was sent in the request). Refer to <a href=\"/developer/api/reference#cvv2-result-codes\"target=\"_blank\">Payment Result Codes for CVV2</a> for possible values.
* @return authCvv2Result
**/
@ApiModelProperty(example = "M", value = "Format: Fixed length, 1 AN
Description: CVV2 result from card issuer (if CVV2 data was sent in the request). Refer to Payment Result Codes for CVV2 for possible values.")
public String getAuthCvv2Result() {
return authCvv2Result;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TransactionRequestReport transactionRequestReport = (TransactionRequestReport) o;
return Objects.equals(this.qpId, transactionRequestReport.qpId) &&
Objects.equals(this.merchantId, transactionRequestReport.merchantId) &&
Objects.equals(this.tranTime, transactionRequestReport.tranTime) &&
Objects.equals(this.tranDate, transactionRequestReport.tranDate) &&
Objects.equals(this.requestType, transactionRequestReport.requestType) &&
Objects.equals(this.tranStatus, transactionRequestReport.tranStatus) &&
Objects.equals(this.disputeFlag, transactionRequestReport.disputeFlag) &&
Objects.equals(this.amtRefunded, transactionRequestReport.amtRefunded) &&
Objects.equals(this.pgId, transactionRequestReport.pgId) &&
Objects.equals(this.pgIdLinked, transactionRequestReport.pgIdLinked) &&
Objects.equals(this.amtTran, transactionRequestReport.amtTran) &&
Objects.equals(this.tranCurrency, transactionRequestReport.tranCurrency) &&
Objects.equals(this.purchaseId, transactionRequestReport.purchaseId) &&
Objects.equals(this.cardholderFirstName, transactionRequestReport.cardholderFirstName) &&
Objects.equals(this.cardholderLastName, transactionRequestReport.cardholderLastName) &&
Objects.equals(this.cardNumber, transactionRequestReport.cardNumber) &&
Objects.equals(this.cardType, transactionRequestReport.cardType) &&
Objects.equals(this.authCode, transactionRequestReport.authCode) &&
Objects.equals(this.acqReferenceNumber, transactionRequestReport.acqReferenceNumber) &&
Objects.equals(this.merchRefNum, transactionRequestReport.merchRefNum) &&
Objects.equals(this.amtConvenienceFee, transactionRequestReport.amtConvenienceFee) &&
Objects.equals(this.amtTranFee, transactionRequestReport.amtTranFee) &&
Objects.equals(this.dbaName, transactionRequestReport.dbaName) &&
Objects.equals(this.amtFunded, transactionRequestReport.amtFunded) &&
Objects.equals(this.fundedCurrency, transactionRequestReport.fundedCurrency) &&
Objects.equals(this.rcode, transactionRequestReport.rcode) &&
Objects.equals(this.authTranId, transactionRequestReport.authTranId) &&
Objects.equals(this.authAvsResult, transactionRequestReport.authAvsResult) &&
Objects.equals(this.authCvv2Result, transactionRequestReport.authCvv2Result);
}
@Override
public int hashCode() {
return Objects.hash(qpId, merchantId, tranTime, tranDate, requestType, tranStatus, disputeFlag, amtRefunded, pgId, pgIdLinked, amtTran, tranCurrency, purchaseId, cardholderFirstName, cardholderLastName, cardNumber, cardType, authCode, acqReferenceNumber, merchRefNum, amtConvenienceFee, amtTranFee, dbaName, amtFunded, fundedCurrency, rcode, authTranId, authAvsResult, authCvv2Result);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TransactionRequestReport {\n");
sb.append(" qpId: ").append(toIndentedString(qpId)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append(" tranTime: ").append(toIndentedString(tranTime)).append("\n");
sb.append(" tranDate: ").append(toIndentedString(tranDate)).append("\n");
sb.append(" requestType: ").append(toIndentedString(requestType)).append("\n");
sb.append(" tranStatus: ").append(toIndentedString(tranStatus)).append("\n");
sb.append(" disputeFlag: ").append(toIndentedString(disputeFlag)).append("\n");
sb.append(" amtRefunded: ").append(toIndentedString(amtRefunded)).append("\n");
sb.append(" pgId: ").append(toIndentedString(pgId)).append("\n");
sb.append(" pgIdLinked: ").append(toIndentedString(pgIdLinked)).append("\n");
sb.append(" amtTran: ").append(toIndentedString(amtTran)).append("\n");
sb.append(" tranCurrency: ").append(toIndentedString(tranCurrency)).append("\n");
sb.append(" purchaseId: ").append(toIndentedString(purchaseId)).append("\n");
sb.append(" cardholderFirstName: ").append(toIndentedString(cardholderFirstName)).append("\n");
sb.append(" cardholderLastName: ").append(toIndentedString(cardholderLastName)).append("\n");
sb.append(" cardNumber: ").append(toIndentedString(cardNumber)).append("\n");
sb.append(" cardType: ").append(toIndentedString(cardType)).append("\n");
sb.append(" authCode: ").append(toIndentedString(authCode)).append("\n");
sb.append(" acqReferenceNumber: ").append(toIndentedString(acqReferenceNumber)).append("\n");
sb.append(" merchRefNum: ").append(toIndentedString(merchRefNum)).append("\n");
sb.append(" amtConvenienceFee: ").append(toIndentedString(amtConvenienceFee)).append("\n");
sb.append(" amtTranFee: ").append(toIndentedString(amtTranFee)).append("\n");
sb.append(" dbaName: ").append(toIndentedString(dbaName)).append("\n");
sb.append(" amtFunded: ").append(toIndentedString(amtFunded)).append("\n");
sb.append(" fundedCurrency: ").append(toIndentedString(fundedCurrency)).append("\n");
sb.append(" rcode: ").append(toIndentedString(rcode)).append("\n");
sb.append(" authTranId: ").append(toIndentedString(authTranId)).append("\n");
sb.append(" authAvsResult: ").append(toIndentedString(authAvsResult)).append("\n");
sb.append(" authCvv2Result: ").append(toIndentedString(authCvv2Result)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy