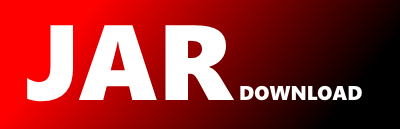
io.swagger.client.model.UpdateBillingCardRequest Maven / Gradle / Ivy
/*
* Qualpay Platform API
* This document describes the Qualpay Platform API.
*
* OpenAPI spec version: 1.1.9
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* UpdateBillingCardRequest
*/
public class UpdateBillingCardRequest {
@SerializedName("exp_date")
private String expDate = null;
@SerializedName("cvv2")
private String cvv2 = null;
@SerializedName("card_id")
private String cardId = null;
@SerializedName("billing_first_name")
private String billingFirstName = null;
@SerializedName("billing_last_name")
private String billingLastName = null;
@SerializedName("billing_firm_name")
private String billingFirmName = null;
@SerializedName("billing_addr1")
private String billingAddr1 = null;
@SerializedName("billing_addr2")
private String billingAddr2 = null;
@SerializedName("billing_city")
private String billingCity = null;
@SerializedName("billing_state")
private String billingState = null;
@SerializedName("billing_zip")
private String billingZip = null;
@SerializedName("billing_zip4")
private String billingZip4 = null;
@SerializedName("billing_country")
private String billingCountry = null;
@SerializedName("billing_country_code")
private String billingCountryCode = null;
@SerializedName("verify")
private Boolean verify = null;
@SerializedName("primary")
private Boolean primary = null;
@SerializedName("merchant_id")
private Long merchantId = null;
public UpdateBillingCardRequest expDate(String expDate) {
this.expDate = expDate;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 4 N, MMYY format<br><strong>Description: </strong>Expiration date of cardholder card number. Use this field if you would like to update the expiration date of the card.
* @return expDate
**/
@ApiModelProperty(example = "0420", value = "Format: Fixed length, 4 N, MMYY format
Description: Expiration date of cardholder card number. Use this field if you would like to update the expiration date of the card.")
public String getExpDate() {
return expDate;
}
public void setExpDate(String expDate) {
this.expDate = expDate;
}
public UpdateBillingCardRequest cvv2(String cvv2) {
this.cvv2 = cvv2;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 4 N<br><strong>Description: </strong>CVV2 or CID value from the signature panel on the back of the cardholder's card. If present during a request where the verify flag is set to true, the value will be sent to the issuer for validation. This field will not be stored in vault.
* @return cvv2
**/
@ApiModelProperty(example = "152", value = "Format: Variable length, up to 4 N
Description: CVV2 or CID value from the signature panel on the back of the cardholder's card. If present during a request where the verify flag is set to true, the value will be sent to the issuer for validation. This field will not be stored in vault. ")
public String getCvv2() {
return cvv2;
}
public void setCvv2(String cvv2) {
this.cvv2 = cvv2;
}
public UpdateBillingCardRequest cardId(String cardId) {
this.cardId = cardId;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 32 AN<br><strong>Description: </strong>Card ID that should be updated.
* @return cardId
**/
@ApiModelProperty(example = "86e1b00d9b0811e68df3069d8f743581", required = true, value = "Format: Fixed length, 32 AN
Description: Card ID that should be updated.")
public String getCardId() {
return cardId;
}
public void setCardId(String cardId) {
this.cardId = cardId;
}
public UpdateBillingCardRequest billingFirstName(String billingFirstName) {
this.billingFirstName = billingFirstName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Billing first name. <br><strong>Conditional Requirement: </strong>Billing first name is required when updating an ACH account number.
* @return billingFirstName
**/
@ApiModelProperty(example = "John", value = "Format: Variable length, up to 32 AN
Description: Billing first name.
Conditional Requirement: Billing first name is required when updating an ACH account number.")
public String getBillingFirstName() {
return billingFirstName;
}
public void setBillingFirstName(String billingFirstName) {
this.billingFirstName = billingFirstName;
}
public UpdateBillingCardRequest billingLastName(String billingLastName) {
this.billingLastName = billingLastName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 32 AN<br><strong>Description: </strong>Billing last name. <br><strong>Conditional Requirement: </strong>Billing last name is required when updating an ACH account number.
* @return billingLastName
**/
@ApiModelProperty(example = "Doe", value = "Format: Variable length, up to 32 AN
Description: Billing last name.
Conditional Requirement: Billing last name is required when updating an ACH account number.")
public String getBillingLastName() {
return billingLastName;
}
public void setBillingLastName(String billingLastName) {
this.billingLastName = billingLastName;
}
public UpdateBillingCardRequest billingFirmName(String billingFirmName) {
this.billingFirmName = billingFirmName;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Business name on billing card, if applicable.
* @return billingFirmName
**/
@ApiModelProperty(example = "Qualpay", value = "Format: Variable length, up to 64 AN
Description: Business name on billing card, if applicable. ")
public String getBillingFirmName() {
return billingFirmName;
}
public void setBillingFirmName(String billingFirmName) {
this.billingFirmName = billingFirmName;
}
public UpdateBillingCardRequest billingAddr1(String billingAddr1) {
this.billingAddr1 = billingAddr1;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Billing street address. This address will also be used for AVS verification if AVS verification is enabled.
* @return billingAddr1
**/
@ApiModelProperty(example = "123 Main Avenue", value = "Format: Variable length, up to 128 AN
Description: Billing street address. This address will also be used for AVS verification if AVS verification is enabled. ")
public String getBillingAddr1() {
return billingAddr1;
}
public void setBillingAddr1(String billingAddr1) {
this.billingAddr1 = billingAddr1;
}
public UpdateBillingCardRequest billingAddr2(String billingAddr2) {
this.billingAddr2 = billingAddr2;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Billing Address line item 2.
* @return billingAddr2
**/
@ApiModelProperty(example = "#1234", value = "Format: Variable length, up to 128 AN
Description: Billing Address line item 2.")
public String getBillingAddr2() {
return billingAddr2;
}
public void setBillingAddr2(String billingAddr2) {
this.billingAddr2 = billingAddr2;
}
public UpdateBillingCardRequest billingCity(String billingCity) {
this.billingCity = billingCity;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 64 AN<br><strong>Description: </strong>Billing city.
* @return billingCity
**/
@ApiModelProperty(example = "San Mateo", value = "Format: Variable length, up to 64 AN
Description: Billing city.")
public String getBillingCity() {
return billingCity;
}
public void setBillingCity(String billingCity) {
this.billingCity = billingCity;
}
public UpdateBillingCardRequest billingState(String billingState) {
this.billingState = billingState;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 3 AN<br><strong>Description: </strong>Billing state.
* @return billingState
**/
@ApiModelProperty(example = "CA", value = "Format: Variable length, up to 3 AN
Description: Billing state.")
public String getBillingState() {
return billingState;
}
public void setBillingState(String billingState) {
this.billingState = billingState;
}
public UpdateBillingCardRequest billingZip(String billingZip) {
this.billingZip = billingZip;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 10 AN<br><strong>Description: </strong>Billing zip. The zipcode will also be used for AVS verification if AVS verification is enabled.
* @return billingZip
**/
@ApiModelProperty(example = "94402", value = "Format: Variable length, up to 10 AN
Description: Billing zip. The zipcode will also be used for AVS verification if AVS verification is enabled.")
public String getBillingZip() {
return billingZip;
}
public void setBillingZip(String billingZip) {
this.billingZip = billingZip;
}
public UpdateBillingCardRequest billingZip4(String billingZip4) {
this.billingZip4 = billingZip4;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 4 N<br><strong>Description: </strong>Billing zip+4 code if applicable.
* @return billingZip4
**/
@ApiModelProperty(example = "1234", value = "Format: Fixed length, 4 N
Description: Billing zip+4 code if applicable.")
public String getBillingZip4() {
return billingZip4;
}
public void setBillingZip4(String billingZip4) {
this.billingZip4 = billingZip4;
}
public UpdateBillingCardRequest billingCountry(String billingCountry) {
this.billingCountry = billingCountry;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 128 AN<br><strong>Description: </strong>Billing country.
* @return billingCountry
**/
@ApiModelProperty(example = "United States", value = "Format: Variable length, up to 128 AN
Description: Billing country.")
public String getBillingCountry() {
return billingCountry;
}
public void setBillingCountry(String billingCountry) {
this.billingCountry = billingCountry;
}
public UpdateBillingCardRequest billingCountryCode(String billingCountryCode) {
this.billingCountryCode = billingCountryCode;
return this;
}
/**
* <strong>Format: </strong>Fixed length, 3 AN<br><strong>Description: </strong>ISO numeric country code for the billing address. Refer to <a href=\"/developer/api/reference#country-codes\"target=\"_blank\">Country Codes</a> for a list of country codes.
* @return billingCountryCode
**/
@ApiModelProperty(example = "840", value = "Format: Fixed length, 3 AN
Description: ISO numeric country code for the billing address. Refer to Country Codes for a list of country codes.")
public String getBillingCountryCode() {
return billingCountryCode;
}
public void setBillingCountryCode(String billingCountryCode) {
this.billingCountryCode = billingCountryCode;
}
public UpdateBillingCardRequest verify(Boolean verify) {
this.verify = verify;
return this;
}
/**
* <br><strong>Description: </strong>Set this field to true if a card_number or card_id should be verified by the issuer before adding to Customer Vault. When this field is set to true, the billing card will be updated either if the card verification is successful or if card verification is not supported by the issuer.<br><strong>Default: </strong>false
* @return verify
**/
@ApiModelProperty(example = "true", value = "
Description: Set this field to true if a card_number or card_id should be verified by the issuer before adding to Customer Vault. When this field is set to true, the billing card will be updated either if the card verification is successful or if card verification is not supported by the issuer.
Default: false")
public Boolean isVerify() {
return verify;
}
public void setVerify(Boolean verify) {
this.verify = verify;
}
public UpdateBillingCardRequest primary(Boolean primary) {
this.primary = primary;
return this;
}
/**
* <br><strong>Description: </strong>Set this field to true if this should be the default card.<br><strong>Default: </strong>false
* @return primary
**/
@ApiModelProperty(example = "true", value = "
Description: Set this field to true if this should be the default card.
Default: false")
public Boolean isPrimary() {
return primary;
}
public void setPrimary(Boolean primary) {
this.primary = primary;
}
public UpdateBillingCardRequest merchantId(Long merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* <strong>Format: </strong>Variable length, up to 16 AN<br><strong>Description: </strong>Identifies the merchant to whom this request applies. Optional field, applicable only if the request is sent on behalf of another merchant.<br><strong>Conditional Requirement: </strong>Required if this request is on behalf of another merchant.
* @return merchantId
**/
@ApiModelProperty(example = "210000000289", value = "Format: Variable length, up to 16 AN
Description: Identifies the merchant to whom this request applies. Optional field, applicable only if the request is sent on behalf of another merchant.
Conditional Requirement: Required if this request is on behalf of another merchant.")
public Long getMerchantId() {
return merchantId;
}
public void setMerchantId(Long merchantId) {
this.merchantId = merchantId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateBillingCardRequest updateBillingCardRequest = (UpdateBillingCardRequest) o;
return Objects.equals(this.expDate, updateBillingCardRequest.expDate) &&
Objects.equals(this.cvv2, updateBillingCardRequest.cvv2) &&
Objects.equals(this.cardId, updateBillingCardRequest.cardId) &&
Objects.equals(this.billingFirstName, updateBillingCardRequest.billingFirstName) &&
Objects.equals(this.billingLastName, updateBillingCardRequest.billingLastName) &&
Objects.equals(this.billingFirmName, updateBillingCardRequest.billingFirmName) &&
Objects.equals(this.billingAddr1, updateBillingCardRequest.billingAddr1) &&
Objects.equals(this.billingAddr2, updateBillingCardRequest.billingAddr2) &&
Objects.equals(this.billingCity, updateBillingCardRequest.billingCity) &&
Objects.equals(this.billingState, updateBillingCardRequest.billingState) &&
Objects.equals(this.billingZip, updateBillingCardRequest.billingZip) &&
Objects.equals(this.billingZip4, updateBillingCardRequest.billingZip4) &&
Objects.equals(this.billingCountry, updateBillingCardRequest.billingCountry) &&
Objects.equals(this.billingCountryCode, updateBillingCardRequest.billingCountryCode) &&
Objects.equals(this.verify, updateBillingCardRequest.verify) &&
Objects.equals(this.primary, updateBillingCardRequest.primary) &&
Objects.equals(this.merchantId, updateBillingCardRequest.merchantId);
}
@Override
public int hashCode() {
return Objects.hash(expDate, cvv2, cardId, billingFirstName, billingLastName, billingFirmName, billingAddr1, billingAddr2, billingCity, billingState, billingZip, billingZip4, billingCountry, billingCountryCode, verify, primary, merchantId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateBillingCardRequest {\n");
sb.append(" expDate: ").append(toIndentedString(expDate)).append("\n");
sb.append(" cvv2: ").append(toIndentedString(cvv2)).append("\n");
sb.append(" cardId: ").append(toIndentedString(cardId)).append("\n");
sb.append(" billingFirstName: ").append(toIndentedString(billingFirstName)).append("\n");
sb.append(" billingLastName: ").append(toIndentedString(billingLastName)).append("\n");
sb.append(" billingFirmName: ").append(toIndentedString(billingFirmName)).append("\n");
sb.append(" billingAddr1: ").append(toIndentedString(billingAddr1)).append("\n");
sb.append(" billingAddr2: ").append(toIndentedString(billingAddr2)).append("\n");
sb.append(" billingCity: ").append(toIndentedString(billingCity)).append("\n");
sb.append(" billingState: ").append(toIndentedString(billingState)).append("\n");
sb.append(" billingZip: ").append(toIndentedString(billingZip)).append("\n");
sb.append(" billingZip4: ").append(toIndentedString(billingZip4)).append("\n");
sb.append(" billingCountry: ").append(toIndentedString(billingCountry)).append("\n");
sb.append(" billingCountryCode: ").append(toIndentedString(billingCountryCode)).append("\n");
sb.append(" verify: ").append(toIndentedString(verify)).append("\n");
sb.append(" primary: ").append(toIndentedString(primary)).append("\n");
sb.append(" merchantId: ").append(toIndentedString(merchantId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy