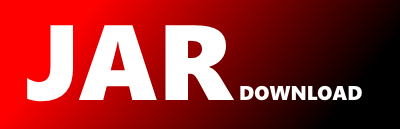
org.killbill.billing.entitlement.api.EntitlementApi Maven / Gradle / Ivy
/*
* Copyright 2010-2013 Ning, Inc.
*
* Ning licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package org.killbill.billing.entitlement.api;
import java.util.List;
import java.util.UUID;
import org.joda.time.LocalDate;
import org.killbill.billing.KillbillApi;
import org.killbill.billing.catalog.api.BillingActionPolicy;
import org.killbill.billing.catalog.api.PlanPhasePriceOverride;
import org.killbill.billing.catalog.api.PlanPhaseSpecifier;
import org.killbill.billing.payment.api.PluginProperty;
import org.killbill.billing.security.RequiresPermissions;
import org.killbill.billing.util.callcontext.CallContext;
import org.killbill.billing.util.callcontext.TenantContext;
import static org.killbill.billing.security.Permission.ENTITLEMENT_CAN_CREATE;
import static org.killbill.billing.security.Permission.ENTITLEMENT_CAN_TRANSFER;
import static org.killbill.billing.security.Permission.ENTITLEMENT_CAN_PAUSE_RESUME;
/**
* Primary API to manage the creation and retrieval of Entitlement
.
*/
public interface EntitlementApi extends KillbillApi {
/**
* Create a new entitlement for that account.
*
* The PlanPhaseSpecifier
should refer to a ProductCategory.BASE
of
* ProductCategory.STANDALONE
.
*
* @param accountId the account id
* @param spec the product specification for that new entitlement
* @param externalKey the external key for that entitlement-- which must be unique in the system
* @param overrides the price override for each phase and for a specific currency
* @param entitlementEffectiveDate the date at which the entitlement should start. if this is null this is assumed now
* @param billingEffectiveDate the date at which the billing for the subscription should start. if this is null this is assumed now
* @param isMigrated whether this subscription comes from a different system (migrated into Kill Bill)
* @param properties plugin specific properties
* @param context the context
* @return a new entitlement
* @throws EntitlementApiException if the system fail to create the Entitlement
.
*/
@RequiresPermissions(ENTITLEMENT_CAN_CREATE)
public Entitlement createBaseEntitlement(UUID accountId, PlanPhaseSpecifier spec, String externalKey, List overrides, LocalDate entitlementEffectiveDate, LocalDate billingEffectiveDate, boolean isMigrated, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
* Create a new base entitlement and addOn entitlements for that account.
*
*
* @param accountId the account id
* @param externalKey the bundle external key
* @param entitlementEffectiveDate the date at which the entitlement should start. if this is null this is assumed now
* @param billingEffectiveDate the date at which the billing for the subscription should start. if this is null this is assumed now
* @param entitlementSpecifier a list of entitlement specifier
* @param isMigrated whether this subscription comes from a different system (migrated into Kill Bill)
* @param properties plugin specific properties
* @param context the context
* @return the common bundle created
* @throws EntitlementApiException if the system fail to create the Entitlement
.
*/
@RequiresPermissions(ENTITLEMENT_CAN_CREATE)
Entitlement createBaseEntitlementWithAddOns(UUID accountId, String externalKey, Iterable entitlementSpecifier, LocalDate entitlementEffectiveDate, LocalDate billingEffectiveDate, boolean isMigrated, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
* Adds an ADD_ON entitlement to previously created entitlement.
*
* The PlanPhaseSpecifier
should refer to a ProductCategory.ADD_ON
.
* The new entitlement will be bundled using the externalKey that was specified when creating the
* base entitlement.
*
* @param bundleId the id of the bundle
* @param spec the product specification for that new entitlement
* @param overrides the price override for each phase and for a specific currency
* @param entitlementEffectiveDate the date at which the entitlement should start. if this is null this is assumed now
* @param billingEffectiveDate the date at which the billing for the subscription should start. if this is null this is assumed now
* @param isMigrated whether this subscription comes from a different system (migrated into Kill Bill)
* @param properties plugin specific properties
* @param context the context
* @return a new entitlement
* @throws EntitlementApiException if the system fail to create the Entitlement
*/
@RequiresPermissions(ENTITLEMENT_CAN_CREATE)
public Entitlement addEntitlement(UUID bundleId, PlanPhaseSpecifier spec, List overrides, LocalDate entitlementEffectiveDate, LocalDate billingEffectiveDate, boolean isMigrated, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
* Simulate a change of product for the BP on that bundle and return the effect it would have on the existing ADD_ON-- if any.
*
* @param bundleId the id of the bundle
* @param targetProductName the target product name for the BP
* @param effectiveDate the date at which the change would occur
* @param context the context
* @return the status for the existing ADD_ON Entitlement
* @throws EntitlementApiException if this operation is not carried on a base plan.
*/
public List getDryRunStatusForChange(UUID bundleId, final String targetProductName, final LocalDate effectiveDate, final TenantContext context)
throws EntitlementApiException;
/**
* Will pause all entitlements associated with the base entitlement. If there are no ADD_ONN this is only the base entitlement.
*
* @param bundleId
* @param effectiveDate
* @param properties plugin specific properties
* @param context
* @throws EntitlementApiException if the system fail to find the base Entitlement
*/
@RequiresPermissions(ENTITLEMENT_CAN_PAUSE_RESUME)
public void pause(UUID bundleId, LocalDate effectiveDate, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
* Will resume all entitlements associated with the base entitlement. If there are no ADD_ONN this is only the base entitlement.
*
* @param bundleId
* @param effectiveDate
* @param properties plugin specific properties
* @param context
* @throws EntitlementApiException if the system fail to find the base Entitlement
*/
@RequiresPermissions(ENTITLEMENT_CAN_PAUSE_RESUME)
public void resume(UUID bundleId, LocalDate effectiveDate, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
* Retrieves an Entitlement
using its id.
*
* @param id the id of the entitlement
* @param context the context
* @return the entitlement
* @throws EntitlementApiException if the entitlement does not exist
*/
public Entitlement getEntitlementForId(UUID id, TenantContext context) throws EntitlementApiException;
/**
* Retrieves all the Entitlement
attached to the base entitlement.
*
* @param bundleId the id of the bundle
* @param context the context
* @return a list of entitlements
* @throws EntitlementApiException if the entitlement does not exist
*/
public List getAllEntitlementsForBundle(UUID bundleId, TenantContext context)
throws EntitlementApiException;
/**
* Retrieves all the Entitlement
for a given account and matching an external key.
*
* @param accountId the account id
* @param externalKey the external key
* @param context the context
* @return a list of entitlements
* @throws EntitlementApiException if the account does not exist
*/
public List getAllEntitlementsForAccountIdAndExternalKey(UUID accountId, String externalKey, TenantContext context)
throws EntitlementApiException;
/**
* Retrieves all the Entitlement
for a given account.
*
* @param accountId the account id
* @param context the context
* @return a list of entitlements
* @throws EntitlementApiException if the account does not exist
*/
public List getAllEntitlementsForAccountId(UUID accountId, TenantContext context) throws EntitlementApiException;
/**
* Transfer all the Entitlement
For the source account and matching the external key to the destination account.
*
* The date is interpreted by the system to be in the timezone specified at the destination Account
.
*
* The Entitlement
on the source account will be cancelled at effective date and the Entitlement
* on the destination account will be created at the effectiveDate.
*
* @param sourceAccountId the unique id for the account on which the bundle will be transferred For
* @param destAccountId the unique id for the account on which the bundle will be transferred to
* @param externalKey the externalKey for the bundle
* @param effectiveDate the date at which this transfer should occur
* @param properties plugin specific properties
* @param context the user context
* @return the id of the newly created bundle for the destination account
* @throws EntitlementApiException if the system could not transfer the entitlements
*/
@RequiresPermissions(ENTITLEMENT_CAN_TRANSFER)
public UUID transferEntitlements(final UUID sourceAccountId, final UUID destAccountId, final String externalKey, final LocalDate effectiveDate,
Iterable properties, final CallContext context)
throws EntitlementApiException;
/**
* Transfer all the Entitlement
for the source account and matching the external key to the destination account.
*
* The date is interpreted by the system to be in the timezone specified at the destination Account
.
*
* The Entitlement
on the source account will be cancelled at effective date and the Entitlement
* on the destination account will be created at the effectiveDate. The billingPolicy will be used to override
* the default billing behavior for the cancellation of the subscriptions on the source account.
*
* @param sourceAccountId the unique id for the account on which the bundle will be transferred For
* @param destAccountId the unique id for the account on which the bundle will be transferred to
* @param externalKey the externalKey for the bundle
* @param effectiveDate the date at which this transfer should occur
* @param billingPolicy the override billing policy
* @param properties plugin specific properties
* @param context the user context
* @return the id of the newly created base entitlement for the destination account
* @throws EntitlementApiException if the system could not transfer the entitlements
*/
@RequiresPermissions(ENTITLEMENT_CAN_TRANSFER)
public UUID transferEntitlementsOverrideBillingPolicy(final UUID sourceAccountId, final UUID destAccountId, final String externalKey, final LocalDate effectiveDate,
final BillingActionPolicy billingPolicy, Iterable properties, final CallContext context)
throws EntitlementApiException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy