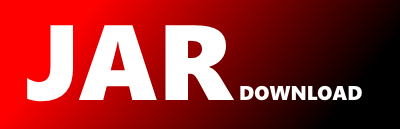
org.killbill.billing.client.model.gen.SimplePlan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of killbill-client-java Show documentation
Show all versions of killbill-client-java Show documentation
Kill Bill Java client library
The newest version!
/*
* Copyright 2010-2014 Ning, Inc.
* Copyright 2014-2020 Groupon, Inc
* Copyright 2020-2021 Equinix, Inc
* Copyright 2014-2021 The Billing Project, LLC
*
* The Billing Project licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package org.killbill.billing.client.model.gen;
import java.util.Objects;
import java.util.Arrays;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import org.killbill.billing.catalog.api.BillingPeriod;
import org.killbill.billing.catalog.api.Currency;
import org.killbill.billing.catalog.api.ProductCategory;
import org.killbill.billing.catalog.api.TimeUnit;
/**
* DO NOT EDIT !!!
*
* This code has been generated by the Kill Bill swagger generator.
* @See https://github.com/killbill/killbill-swagger-coden
*/
import org.killbill.billing.client.model.KillBillObject;
public class SimplePlan {
private String planId = null;
private String productName = null;
private ProductCategory productCategory = null;
private Currency currency = null;
private BigDecimal amount = null;
private BillingPeriod billingPeriod = null;
private Integer trialLength = null;
private TimeUnit trialTimeUnit = null;
private List availableBaseProducts = null;
public SimplePlan() {
}
public SimplePlan(final String planId,
final String productName,
final ProductCategory productCategory,
final Currency currency,
final BigDecimal amount,
final BillingPeriod billingPeriod,
final Integer trialLength,
final TimeUnit trialTimeUnit,
final List availableBaseProducts) {
this.planId = planId;
this.productName = productName;
this.productCategory = productCategory;
this.currency = currency;
this.amount = amount;
this.billingPeriod = billingPeriod;
this.trialLength = trialLength;
this.trialTimeUnit = trialTimeUnit;
this.availableBaseProducts = availableBaseProducts;
}
public SimplePlan setPlanId(final String planId) {
this.planId = planId;
return this;
}
public String getPlanId() {
return planId;
}
public SimplePlan setProductName(final String productName) {
this.productName = productName;
return this;
}
public String getProductName() {
return productName;
}
public SimplePlan setProductCategory(final ProductCategory productCategory) {
this.productCategory = productCategory;
return this;
}
public ProductCategory getProductCategory() {
return productCategory;
}
public SimplePlan setCurrency(final Currency currency) {
this.currency = currency;
return this;
}
public Currency getCurrency() {
return currency;
}
public SimplePlan setAmount(final BigDecimal amount) {
this.amount = amount;
return this;
}
public BigDecimal getAmount() {
return amount;
}
public SimplePlan setBillingPeriod(final BillingPeriod billingPeriod) {
this.billingPeriod = billingPeriod;
return this;
}
public BillingPeriod getBillingPeriod() {
return billingPeriod;
}
public SimplePlan setTrialLength(final Integer trialLength) {
this.trialLength = trialLength;
return this;
}
public Integer getTrialLength() {
return trialLength;
}
public SimplePlan setTrialTimeUnit(final TimeUnit trialTimeUnit) {
this.trialTimeUnit = trialTimeUnit;
return this;
}
public TimeUnit getTrialTimeUnit() {
return trialTimeUnit;
}
public SimplePlan setAvailableBaseProducts(final List availableBaseProducts) {
this.availableBaseProducts = availableBaseProducts;
return this;
}
public SimplePlan addAvailableBaseProductsItem(final String availableBaseProductsItem) {
if (this.availableBaseProducts == null) {
this.availableBaseProducts = new ArrayList();
}
this.availableBaseProducts.add(availableBaseProductsItem);
return this;
}
public List getAvailableBaseProducts() {
return availableBaseProducts;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SimplePlan simplePlan = (SimplePlan) o;
return Objects.equals(this.planId, simplePlan.planId) &&
Objects.equals(this.productName, simplePlan.productName) &&
Objects.equals(this.productCategory, simplePlan.productCategory) &&
Objects.equals(this.currency, simplePlan.currency) &&
Objects.equals(this.amount, simplePlan.amount) &&
Objects.equals(this.billingPeriod, simplePlan.billingPeriod) &&
Objects.equals(this.trialLength, simplePlan.trialLength) &&
Objects.equals(this.trialTimeUnit, simplePlan.trialTimeUnit) &&
Objects.equals(this.availableBaseProducts, simplePlan.availableBaseProducts);
}
@Override
public int hashCode() {
return Objects.hash(planId,
productName,
productCategory,
currency,
amount,
billingPeriod,
trialLength,
trialTimeUnit,
availableBaseProducts);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SimplePlan {\n");
sb.append(" planId: ").append(toIndentedString(planId)).append("\n");
sb.append(" productName: ").append(toIndentedString(productName)).append("\n");
sb.append(" productCategory: ").append(toIndentedString(productCategory)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" billingPeriod: ").append(toIndentedString(billingPeriod)).append("\n");
sb.append(" trialLength: ").append(toIndentedString(trialLength)).append("\n");
sb.append(" trialTimeUnit: ").append(toIndentedString(trialTimeUnit)).append("\n");
sb.append(" availableBaseProducts: ").append(toIndentedString(availableBaseProducts)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy