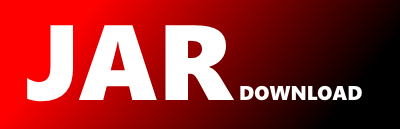
com.thoughtworks.xstream.converters.enums.EnumToStringConverter Maven / Gradle / Ivy
/*
* Copyright (C) 2013, 2016 XStream Committers.
* All rights reserved.
*
* The software in this package is published under the terms of the BSD
* style license a copy of which has been included with this distribution in
* the LICENSE.txt file.
*
* Created on 14. March 2013 by Joerg Schaible
*/
package com.thoughtworks.xstream.converters.enums;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import com.thoughtworks.xstream.InitializationException;
import com.thoughtworks.xstream.converters.ConversionException;
import com.thoughtworks.xstream.converters.basic.AbstractSingleValueConverter;
/**
* A single value converter for a special enum type using its string representation.
*
* @author Jörg Schaible
* @since 1.4.5
*/
public class EnumToStringConverter> extends AbstractSingleValueConverter {
private final Class enumType;
private final Map strings;
private final EnumMap values;
public EnumToStringConverter(Class type) {
this(type, extractStringMap(type), null);
}
public EnumToStringConverter(Class type, Map strings) {
this(type, strings, buildValueMap(type, strings));
}
private EnumToStringConverter(
Class type, Map strings, EnumMap values) {
enumType = type;
this.strings = strings;
this.values = values;
}
private static > Map extractStringMap(Class type) {
checkType(type);
EnumSet values = EnumSet.allOf(type);
Map strings = new HashMap(values.size());
for (T value : values) {
if (strings.put(value.toString(), value) != null) {
throw new InitializationException("Enum type "
+ type.getName()
+ " does not have unique string representations for its values");
}
}
return strings;
}
private static void checkType(Class type) {
if (!Enum.class.isAssignableFrom(type) && type != Enum.class) {
throw new InitializationException("Converter can only handle enum types");
}
}
private static > EnumMap buildValueMap(Class type,
Map strings) {
EnumMap values = new EnumMap(type);
for (Map.Entry entry : strings.entrySet()) {
values.put(entry.getValue(), entry.getKey());
}
return values;
}
@Override
public boolean canConvert(Class type) {
return enumType.isAssignableFrom(type);
}
@Override
public String toString(Object obj) {
Enum value = Enum.class.cast(obj);
return values == null ? value.toString() : values.get(value);
}
@Override
public Object fromString(String str) {
if (str == null) {
return null;
}
T result = strings.get(str);
if (result == null) {
ConversionException exception = new ConversionException("Invalid string representation for enum type");
exception.add("enum-type", enumType.getName());
exception.add("enum-string", str);
throw exception;
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy