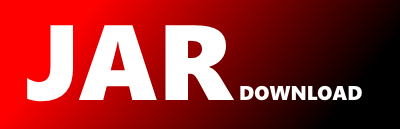
org.killbill.billing.entitlement.api.SubscriptionApi Maven / Gradle / Ivy
/*
* Copyright 2010-2013 Ning, Inc.
*
* Ning licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package org.killbill.billing.entitlement.api;
import java.util.List;
import java.util.UUID;
import org.joda.time.LocalDate;
import org.killbill.billing.KillbillApi;
import org.killbill.billing.OrderingType;
import org.killbill.billing.payment.api.PluginProperty;
import org.killbill.billing.security.RequiresPermissions;
import org.killbill.billing.util.api.AuditLevel;
import org.killbill.billing.util.audit.AuditLogWithHistory;
import org.killbill.billing.util.callcontext.CallContext;
import org.killbill.billing.util.callcontext.TenantContext;
import org.killbill.billing.util.entity.Pagination;
import static org.killbill.billing.security.Permission.ENTITLEMENT_CAN_CREATE;
import static org.killbill.billing.security.Permission.ENTITLEMENT_CAN_PAUSE_RESUME;
/**
* API to manage the retrieval of Subscription
information.
*/
public interface SubscriptionApi extends KillbillApi {
int PAST_EVENTS = 0x1;
int PRESENT_EVENTS = 0x2;
int FUTURE_EVENTS = 0x4;
int PAST_OR_PRESENT_EVENTS = (PAST_EVENTS | PRESENT_EVENTS);
int FUTURE_OR_PRESENT_EVENTS = (PRESENT_EVENTS | FUTURE_EVENTS);
int ALL_EVENTS = (PAST_OR_PRESENT_EVENTS | FUTURE_OR_PRESENT_EVENTS);
/**
* Retrieves a Subscription
for the entitlement id
*
* @param entitlementId the id of the entitlement associated with the subscription
* @param context the context
* @return the subscription
* @throws SubscriptionApiException if it odes not exist
*/
Subscription getSubscriptionForEntitlementId(UUID entitlementId, TenantContext context) throws SubscriptionApiException;
/**
* Retrieves a Subscription
for a given external key
*
* @param externalKey the external key for the subscription
* @param context
* @return
* @throws SubscriptionApiException
*/
Subscription getSubscriptionForExternalKey(String externalKey, TenantContext context) throws SubscriptionApiException;
/**
* Retrieves all the Subscription
attached to the base entitlement.
*
* @param bundleId the id of the bundle
* @param context the context
* @return a list of subscriptions
* @throws SubscriptionApiException if the baseEntitlementId does not exist.
*/
public SubscriptionBundle getSubscriptionBundle(UUID bundleId, TenantContext context) throws SubscriptionApiException;
/**
* Update the externalKey for a given bundle
*
* @param bundleId ; bundle id
* @param newExternalKey : the new value for the externalKey
* @param context : the call context
*/
@RequiresPermissions(ENTITLEMENT_CAN_CREATE)
public void updateExternalKey(UUID bundleId, String newExternalKey, CallContext context) throws EntitlementApiException;
/**
* Retrieves all the SubscriptionBundle
for a given account and matching an external key.
*
* @param accountId the account id
* @param externalKey the external key
* @param context the context
* @return a SubscriptionBundle
* @throws SubscriptionApiException if there is n o such object matching the account and external key
*/
public List getSubscriptionBundlesForAccountIdAndExternalKey(UUID accountId, String externalKey, TenantContext context) throws SubscriptionApiException;
/**
* Retrieves all the SubscriptionBundle
for the given external key.
*
* It is possible to have multiple SubscriptionBundle
for a given external key in the system but only one
* will be active -- i.e. will contain Subscription
in the active state.
*
* @param externalKey the external key
* @param context the context
* @return a SubscriptionBundle
* @throws SubscriptionApiException if there is no such object
*/
public SubscriptionBundle getActiveSubscriptionBundleForExternalKey(String externalKey, TenantContext context) throws SubscriptionApiException;
/**
* Returns an ordered list of all SubscriptionBundle
for a given external key.
*
* @return
*/
public List getSubscriptionBundlesForExternalKey(String externalKey, TenantContext context) throws SubscriptionApiException;
/**
* Retrieves all the SubscriptionBundle
for a given account.
*
* @param accountId the account id
* @param context the context
* @return list of SubscriptionBundle
* @throws SubscriptionApiException if the account does not exist
*/
public List getSubscriptionBundlesForAccountId(UUID accountId, TenantContext context) throws SubscriptionApiException;
/**
* @param context the user context
* @param offset the offset of the first result
* @param limit the maximum number of results to retrieve
* @return the list of SubscriptionBundle
for that tenant
*/
public Pagination getSubscriptionBundles(Long offset, Long limit, TenantContext context);
/**
* Find all SubscriptionBundle
having their id, account id or external key matching the search key
*
* @param searchKey the search key
* @param offset the offset of the first result
* @param limit the maximum number of results to retrieve
* @param context the user context
* @return the list of SubscriptionBundle
matching this search key for that tenant
*/
public Pagination searchSubscriptionBundles(String searchKey, Long offset, Long limit, TenantContext context);
/**
* Add a BlockingState
*
* The date is interpreted by the system to be in the timezone specified at the Account
*
* @param blockingState the blockingState to be added
* @param effectiveDate the date in the account time zone at which the operation should be effective, if null this is interpreted to be immediate
* @param properties plugin specific properties
* @param context the context
* @throws EntitlementApiException if the entitlement was not in ACTIVE state
*/
@RequiresPermissions(ENTITLEMENT_CAN_PAUSE_RESUME)
public void addBlockingState(BlockingState blockingState, LocalDate effectiveDate, Iterable properties, CallContext context)
throws EntitlementApiException;
/**
*
* @param accountId the account id
* @param typeFilter the list of BlockingStateType
filters. All types are returned if null or empty.
* @param svcsFilter the list of service filters. All services are returned if null or empty.
* @param orderingType the ordering direction, that is ASCENDING
or DESCENDING
* @param timeFilter the filtering types constructed as a bitwise operation
* @param context the user context
*
* @throws EntitlementApiException if the account is invalid.
* @return the ordered list of BlockingState
*/
public Iterable getBlockingStates(UUID accountId, List typeFilter, List svcsFilter, OrderingType orderingType, int timeFilter, TenantContext context)
throws EntitlementApiException;
/**
* Get all the audit entries with history for a given bundle.
*
* @param bundleId the bundleId id
* @param auditLevel audit level (verbosity)
* @param context the tenant context
* @return all audit entries with history for a bundle
*/
List getSubscriptionBundleAuditLogsWithHistoryForId(UUID bundleId, AuditLevel auditLevel, TenantContext context);
/**
* Get all the audit entries with history for a given subscription.
*
* @param entitlementId the entitlementId id
* @param auditLevel audit level (verbosity)
* @param context the tenant context
* @return all audit entries with history for a subscription
*/
List getSubscriptionAuditLogsWithHistoryForId(UUID entitlementId, AuditLevel auditLevel, TenantContext context);
/**
* Get all the audit entries with history for a given subscription event.
*
* @param EventId the subscription event id
* @param auditLevel audit level (verbosity)
* @param context the tenant context
* @return all audit entries with history for a subscription event
*/
List getSubscriptionEventAuditLogsWithHistoryForId(UUID EventId, AuditLevel auditLevel, TenantContext context);
/**
* Get all the audit entries with history for a given blocking state.
*
* @param blockingId the blocking id
* @param auditLevel audit level (verbosity)
* @param context the tenant context
* @return all audit entries with history for a blocking state
*/
List getBlockingStateAuditLogsWithHistoryForId(UUID blockingId, AuditLevel auditLevel, TenantContext context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy