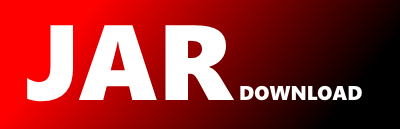
vlsi.utils.CompactHashMapClassEmptyDefaults Maven / Gradle / Ivy
/*
* Copyright (c) 2011
*
* This file is part of CompactMap
*
* CompactMap is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* CompactMap is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with CompactMap. If not, see .
*/
package vlsi.utils;
import com.github.andrewoma.dexx.collection.Pair;
import java.util.Collections;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.Map;
/**
* This map represents CompactHashMapClass that has no default values (it can have nonempty key2slot).
* It is used to determine the right CompactHashMapClass given the desired defaultValues map.
*
* @author Vladimir Sitnikov
* @param the type of keys maintained by this map
* @param the type of mapped values
*/
class CompactHashMapClassEmptyDefaults extends CompactHashMapClass {
private Map> key2newKlass;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy