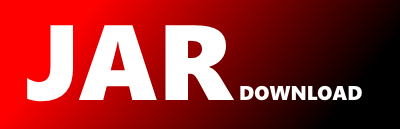
vlsi.utils.CompactHashMapDefaultValues Maven / Gradle / Ivy
/*
* Copyright (c) 2011
*
* This file is part of CompactMap
*
* CompactMap is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* CompactMap is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with CompactMap. If not, see .
*/
package vlsi.utils;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.Map;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class CompactHashMapDefaultValues {
// Key -> Value -> OldMap -> NewMap
private static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy