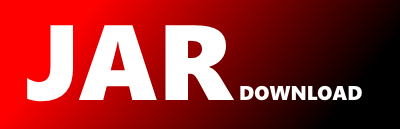
org.killbill.billing.util.entity.dao.EntityDaoBase Maven / Gradle / Ivy
/*
* Copyright 2010-2012 Ning, Inc.
*
* Ning licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package org.killbill.billing.util.entity.dao;
import java.util.Iterator;
import java.util.UUID;
import org.killbill.billing.BillingExceptionBase;
import org.killbill.billing.callcontext.InternalCallContext;
import org.killbill.billing.callcontext.InternalTenantContext;
import org.killbill.billing.util.audit.ChangeType;
import org.killbill.billing.util.entity.DefaultPagination;
import org.killbill.billing.util.entity.Entity;
import org.killbill.billing.util.entity.Pagination;
import org.killbill.billing.util.entity.dao.DefaultPaginationSqlDaoHelper.PaginationIteratorBuilder;
public abstract class EntityDaoBase, E extends Entity, U extends BillingExceptionBase> implements EntityDao {
protected final EntitySqlDaoTransactionalJdbiWrapper transactionalSqlDao;
protected final DefaultPaginationSqlDaoHelper paginationHelper;
private final Class extends EntitySqlDao> realSqlDao;
public EntityDaoBase(final EntitySqlDaoTransactionalJdbiWrapper transactionalSqlDao, final Class extends EntitySqlDao> realSqlDao) {
this.transactionalSqlDao = transactionalSqlDao;
this.realSqlDao = realSqlDao;
this.paginationHelper = new DefaultPaginationSqlDaoHelper(transactionalSqlDao);
}
@Override
public void create(final M entity, final InternalCallContext context) throws U {
transactionalSqlDao.execute(getCreateEntitySqlDaoTransactionWrapper(entity, context));
}
protected EntitySqlDaoTransactionWrapper getCreateEntitySqlDaoTransactionWrapper(final M entity, final InternalCallContext context) {
return new EntitySqlDaoTransactionWrapper() {
@Override
public Void inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
if (checkEntityAlreadyExists(transactional, entity, context)) {
throw generateAlreadyExistsException(entity, context);
}
transactional.create(entity, context);
final M refreshedEntity = transactional.getById(entity.getId().toString(), context);
postBusEventFromTransaction(entity, refreshedEntity, ChangeType.INSERT, entitySqlDaoWrapperFactory, context);
return null;
}
};
}
protected boolean checkEntityAlreadyExists(final EntitySqlDao transactional, final M entity, final InternalCallContext context) {
return transactional.getById(entity.getId().toString(), context) != null;
}
protected void postBusEventFromTransaction(final M entity, final M savedEntity, final ChangeType changeType,
final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory,
final InternalCallContext context) throws BillingExceptionBase {
}
protected abstract U generateAlreadyExistsException(final M entity, final InternalCallContext context);
protected String getNaturalOrderingColumns() {
return "record_id";
}
@Override
public Long getRecordId(final UUID id, final InternalTenantContext context) {
return transactionalSqlDao.execute(new EntitySqlDaoTransactionWrapper() {
@Override
public Long inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
return transactional.getRecordId(id.toString(), context);
}
});
}
@Override
public M getByRecordId(final Long recordId, final InternalTenantContext context) {
return transactionalSqlDao.execute(new EntitySqlDaoTransactionWrapper() {
@Override
public M inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
return transactional.getByRecordId(recordId, context);
}
});
}
@Override
public M getById(final UUID id, final InternalTenantContext context) {
return transactionalSqlDao.execute(new EntitySqlDaoTransactionWrapper() {
@Override
public M inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
return transactional.getById(id.toString(), context);
}
});
}
@Override
public Pagination getAll(final InternalTenantContext context) {
// We usually always want to wrap our queries in an EntitySqlDaoTransactionWrapper... except here.
// Since we want to stream the results out, we don't want to auto-commit when this method returns.
final EntitySqlDao sqlDao = transactionalSqlDao.onDemandForStreamingResults(realSqlDao);
// Note: we need to perform the count before streaming the results, as the connection
// will be busy as we stream the results out. This is also why we cannot use
// SQL_CALC_FOUND_ROWS / FOUND_ROWS (which may not be faster anyways).
final Long count = sqlDao.getCount(context);
final Iterator results = sqlDao.getAll(context);
return new DefaultPagination(count, results);
}
@Override
public Pagination get(final Long offset, final Long limit, final InternalTenantContext context) {
return paginationHelper.getPagination(realSqlDao,
new PaginationIteratorBuilder>() {
@Override
public Long getCount(final EntitySqlDao sqlDao, final InternalTenantContext context) {
return sqlDao.getCount(context);
}
@Override
public Iterator build(final EntitySqlDao sqlDao, final Long limit, final InternalTenantContext context) {
return sqlDao.get(offset, limit, getNaturalOrderingColumns(), context);
}
},
offset,
limit,
context);
}
@Override
public Long getCount(final InternalTenantContext context) {
return transactionalSqlDao.execute(new EntitySqlDaoTransactionWrapper() {
@Override
public Long inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
return transactional.getCount(context);
}
});
}
@Override
public void test(final InternalTenantContext context) {
transactionalSqlDao.execute(new EntitySqlDaoTransactionWrapper() {
@Override
public Void inTransaction(final EntitySqlDaoWrapperFactory entitySqlDaoWrapperFactory) throws Exception {
final EntitySqlDao transactional = entitySqlDaoWrapperFactory.become(realSqlDao);
transactional.test(context);
return null;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy