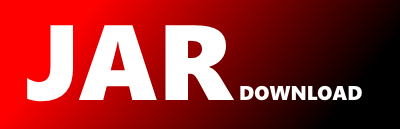
org.kiwiproject.consul.model.acl.ImmutableToken Maven / Gradle / Ivy
package org.kiwiproject.consul.model.acl;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Token}.
*
* Use the builder to create immutable instances:
* {@code ImmutableToken.builder()}.
*/
@Generated(from = "Token", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableToken extends Token {
private final @Nullable String id;
private final @Nullable String secretId;
private final @Nullable String description;
private final ImmutableList policies;
private final ImmutableList roles;
private final ImmutableList serviceIdentities;
private final ImmutableList nodeIdentities;
private final @Nullable Boolean local;
private final @Nullable String expirationTime;
private final @Nullable String expirationTTL;
private final @Nullable String namespace;
private ImmutableToken(
@Nullable String id,
@Nullable String secretId,
@Nullable String description,
ImmutableList policies,
ImmutableList roles,
ImmutableList serviceIdentities,
ImmutableList nodeIdentities,
@Nullable Boolean local,
@Nullable String expirationTime,
@Nullable String expirationTTL,
@Nullable String namespace) {
this.id = id;
this.secretId = secretId;
this.description = description;
this.policies = policies;
this.roles = roles;
this.serviceIdentities = serviceIdentities;
this.nodeIdentities = nodeIdentities;
this.local = local;
this.expirationTime = expirationTime;
this.expirationTTL = expirationTTL;
this.namespace = namespace;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("AccessorID")
@Override
public Optional id() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code secretId} attribute
*/
@JsonProperty("SecretID")
@Override
public Optional secretId() {
return Optional.ofNullable(secretId);
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("Description")
@Override
public Optional description() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code policies} attribute
*/
@JsonProperty("Policies")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.PolicyLink.class)
@Override
public ImmutableList policies() {
return policies;
}
/**
* @return The value of the {@code roles} attribute
*/
@JsonProperty("Roles")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.RoleLink.class)
@Override
public ImmutableList roles() {
return roles;
}
/**
* @return The value of the {@code serviceIdentities} attribute
*/
@JsonProperty("ServiceIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.ServiceIdentity.class)
@Override
public ImmutableList serviceIdentities() {
return serviceIdentities;
}
/**
* @return The value of the {@code nodeIdentities} attribute
*/
@JsonProperty("NodeIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.NodeIdentity.class)
@Override
public ImmutableList nodeIdentities() {
return nodeIdentities;
}
/**
* @return The value of the {@code local} attribute
*/
@JsonProperty("Local")
@Override
public Optional local() {
return Optional.ofNullable(local);
}
/**
* @return The value of the {@code expirationTime} attribute
*/
@JsonProperty("ExpirationTime")
@Override
public Optional expirationTime() {
return Optional.ofNullable(expirationTime);
}
/**
* @return The value of the {@code expirationTTL} attribute
*/
@JsonProperty("ExpirationTTL")
@Override
public Optional expirationTTL() {
return Optional.ofNullable(expirationTTL);
}
/**
* @return The value of the {@code namespace} attribute
*/
@JsonProperty("Namespace")
@Override
public Optional namespace() {
return Optional.ofNullable(namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#id() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return new ImmutableToken(
newValue,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#id() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return new ImmutableToken(
value,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#secretId() secretId} attribute.
* @param value The value for secretId
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withSecretId(String value) {
String newValue = Objects.requireNonNull(value, "secretId");
if (Objects.equals(this.secretId, newValue)) return this;
return new ImmutableToken(
this.id,
newValue,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#secretId() secretId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for secretId
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withSecretId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.secretId, value)) return this;
return new ImmutableToken(
this.id,
value,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#description() description} attribute.
* @param value The value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withDescription(String value) {
String newValue = Objects.requireNonNull(value, "description");
if (Objects.equals(this.description, newValue)) return this;
return new ImmutableToken(
this.id,
this.secretId,
newValue,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#description() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return new ImmutableToken(
this.id,
this.secretId,
value,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#policies() policies}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withPolicies(Token.PolicyLink... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
newValue,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#policies() policies}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of policies elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withPolicies(Iterable extends Token.PolicyLink> elements) {
if (this.policies == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
newValue,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#roles() roles}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withRoles(Token.RoleLink... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
newValue,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#roles() roles}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of roles elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withRoles(Iterable extends Token.RoleLink> elements) {
if (this.roles == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
newValue,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#serviceIdentities() serviceIdentities}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withServiceIdentities(Token.ServiceIdentity... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
newValue,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#serviceIdentities() serviceIdentities}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of serviceIdentities elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withServiceIdentities(Iterable extends Token.ServiceIdentity> elements) {
if (this.serviceIdentities == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
newValue,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#nodeIdentities() nodeIdentities}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withNodeIdentities(Token.NodeIdentity... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
newValue,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Token#nodeIdentities() nodeIdentities}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of nodeIdentities elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withNodeIdentities(Iterable extends Token.NodeIdentity> elements) {
if (this.nodeIdentities == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
newValue,
this.local,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#local() local} attribute.
* @param value The value for local
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withLocal(boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.local, newValue)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
newValue,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#local() local} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for local
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withLocal(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.local, value)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
value,
this.expirationTime,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#expirationTime() expirationTime} attribute.
* @param value The value for expirationTime
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withExpirationTime(String value) {
String newValue = Objects.requireNonNull(value, "expirationTime");
if (Objects.equals(this.expirationTime, newValue)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
newValue,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#expirationTime() expirationTime} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for expirationTime
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withExpirationTime(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.expirationTime, value)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
value,
this.expirationTTL,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#expirationTTL() expirationTTL} attribute.
* @param value The value for expirationTTL
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withExpirationTTL(String value) {
String newValue = Objects.requireNonNull(value, "expirationTTL");
if (Objects.equals(this.expirationTTL, newValue)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
newValue,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#expirationTTL() expirationTTL} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for expirationTTL
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withExpirationTTL(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.expirationTTL, value)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
value,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Token#namespace() namespace} attribute.
* @param value The value for namespace
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withNamespace(String value) {
String newValue = Objects.requireNonNull(value, "namespace");
if (Objects.equals(this.namespace, newValue)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Token#namespace() namespace} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for namespace
* @return A modified copy of {@code this} object
*/
public final ImmutableToken withNamespace(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.namespace, value)) return this;
return new ImmutableToken(
this.id,
this.secretId,
this.description,
this.policies,
this.roles,
this.serviceIdentities,
this.nodeIdentities,
this.local,
this.expirationTime,
this.expirationTTL,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableToken} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableToken
&& equalTo(0, (ImmutableToken) another);
}
private boolean equalTo(int synthetic, ImmutableToken another) {
return Objects.equals(id, another.id)
&& Objects.equals(secretId, another.secretId)
&& Objects.equals(description, another.description)
&& policies.equals(another.policies)
&& roles.equals(another.roles)
&& serviceIdentities.equals(another.serviceIdentities)
&& nodeIdentities.equals(another.nodeIdentities)
&& Objects.equals(local, another.local)
&& Objects.equals(expirationTime, another.expirationTime)
&& Objects.equals(expirationTTL, another.expirationTTL)
&& Objects.equals(namespace, another.namespace);
}
/**
* Computes a hash code from attributes: {@code id}, {@code secretId}, {@code description}, {@code policies}, {@code roles}, {@code serviceIdentities}, {@code nodeIdentities}, {@code local}, {@code expirationTime}, {@code expirationTTL}, {@code namespace}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + Objects.hashCode(secretId);
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + policies.hashCode();
h += (h << 5) + roles.hashCode();
h += (h << 5) + serviceIdentities.hashCode();
h += (h << 5) + nodeIdentities.hashCode();
h += (h << 5) + Objects.hashCode(local);
h += (h << 5) + Objects.hashCode(expirationTime);
h += (h << 5) + Objects.hashCode(expirationTTL);
h += (h << 5) + Objects.hashCode(namespace);
return h;
}
/**
* Prints the immutable value {@code Token} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Token")
.omitNullValues()
.add("id", id)
.add("secretId", secretId)
.add("description", description)
.add("policies", policies)
.add("roles", roles)
.add("serviceIdentities", serviceIdentities)
.add("nodeIdentities", nodeIdentities)
.add("local", local)
.add("expirationTime", expirationTime)
.add("expirationTTL", expirationTTL)
.add("namespace", namespace)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "Token", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends Token {
@Nullable Optional id = Optional.empty();
@Nullable Optional secretId = Optional.empty();
@Nullable Optional description = Optional.empty();
@Nullable List policies = ImmutableList.of();
@Nullable List roles = ImmutableList.of();
@Nullable List serviceIdentities = ImmutableList.of();
@Nullable List nodeIdentities = ImmutableList.of();
@Nullable Optional local = Optional.empty();
@Nullable Optional expirationTime = Optional.empty();
@Nullable Optional expirationTTL = Optional.empty();
@Nullable Optional namespace = Optional.empty();
@JsonProperty("AccessorID")
public void setId(Optional id) {
this.id = id;
}
@JsonProperty("SecretID")
public void setSecretId(Optional secretId) {
this.secretId = secretId;
}
@JsonProperty("Description")
public void setDescription(Optional description) {
this.description = description;
}
@JsonProperty("Policies")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.PolicyLink.class)
public void setPolicies(List policies) {
this.policies = policies;
}
@JsonProperty("Roles")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.RoleLink.class)
public void setRoles(List roles) {
this.roles = roles;
}
@JsonProperty("ServiceIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.ServiceIdentity.class)
public void setServiceIdentities(List serviceIdentities) {
this.serviceIdentities = serviceIdentities;
}
@JsonProperty("NodeIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.NodeIdentity.class)
public void setNodeIdentities(List nodeIdentities) {
this.nodeIdentities = nodeIdentities;
}
@JsonProperty("Local")
public void setLocal(Optional local) {
this.local = local;
}
@JsonProperty("ExpirationTime")
public void setExpirationTime(Optional expirationTime) {
this.expirationTime = expirationTime;
}
@JsonProperty("ExpirationTTL")
public void setExpirationTTL(Optional expirationTTL) {
this.expirationTTL = expirationTTL;
}
@JsonProperty("Namespace")
public void setNamespace(Optional namespace) {
this.namespace = namespace;
}
@Override
public Optional id() { throw new UnsupportedOperationException(); }
@Override
public Optional secretId() { throw new UnsupportedOperationException(); }
@Override
public Optional description() { throw new UnsupportedOperationException(); }
@Override
public List policies() { throw new UnsupportedOperationException(); }
@Override
public List roles() { throw new UnsupportedOperationException(); }
@Override
public List serviceIdentities() { throw new UnsupportedOperationException(); }
@Override
public List nodeIdentities() { throw new UnsupportedOperationException(); }
@Override
public Optional local() { throw new UnsupportedOperationException(); }
@Override
public Optional expirationTime() { throw new UnsupportedOperationException(); }
@Override
public Optional expirationTTL() { throw new UnsupportedOperationException(); }
@Override
public Optional namespace() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableToken fromJson(Json json) {
ImmutableToken.Builder builder = ImmutableToken.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.secretId != null) {
builder.secretId(json.secretId);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.policies != null) {
builder.addAllPolicies(json.policies);
}
if (json.roles != null) {
builder.addAllRoles(json.roles);
}
if (json.serviceIdentities != null) {
builder.addAllServiceIdentities(json.serviceIdentities);
}
if (json.nodeIdentities != null) {
builder.addAllNodeIdentities(json.nodeIdentities);
}
if (json.local != null) {
builder.local(json.local);
}
if (json.expirationTime != null) {
builder.expirationTime(json.expirationTime);
}
if (json.expirationTTL != null) {
builder.expirationTTL(json.expirationTTL);
}
if (json.namespace != null) {
builder.namespace(json.namespace);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Token} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Token instance
*/
public static ImmutableToken copyOf(Token instance) {
if (instance instanceof ImmutableToken) {
return (ImmutableToken) instance;
}
return ImmutableToken.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableToken ImmutableToken}.
*
* ImmutableToken.builder()
* .id(String) // optional {@link Token#id() id}
* .secretId(String) // optional {@link Token#secretId() secretId}
* .description(String) // optional {@link Token#description() description}
* .addPolicies|addAllPolicies(org.kiwiproject.consul.model.acl.Token.PolicyLink) // {@link Token#policies() policies} elements
* .addRoles|addAllRoles(org.kiwiproject.consul.model.acl.Token.RoleLink) // {@link Token#roles() roles} elements
* .addServiceIdentities|addAllServiceIdentities(org.kiwiproject.consul.model.acl.Token.ServiceIdentity) // {@link Token#serviceIdentities() serviceIdentities} elements
* .addNodeIdentities|addAllNodeIdentities(org.kiwiproject.consul.model.acl.Token.NodeIdentity) // {@link Token#nodeIdentities() nodeIdentities} elements
* .local(Boolean) // optional {@link Token#local() local}
* .expirationTime(String) // optional {@link Token#expirationTime() expirationTime}
* .expirationTTL(String) // optional {@link Token#expirationTTL() expirationTTL}
* .namespace(String) // optional {@link Token#namespace() namespace}
* .build();
*
* @return A new ImmutableToken builder
*/
public static ImmutableToken.Builder builder() {
return new ImmutableToken.Builder();
}
/**
* Builds instances of type {@link ImmutableToken ImmutableToken}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Token", generator = "Immutables")
public static final class Builder {
private @Nullable String id;
private @Nullable String secretId;
private @Nullable String description;
private ImmutableList.Builder policies = ImmutableList.builder();
private ImmutableList.Builder roles = ImmutableList.builder();
private ImmutableList.Builder serviceIdentities = ImmutableList.builder();
private ImmutableList.Builder nodeIdentities = ImmutableList.builder();
private @Nullable Boolean local;
private @Nullable String expirationTime;
private @Nullable String expirationTTL;
private @Nullable String namespace;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Token} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Token instance) {
Objects.requireNonNull(instance, "instance");
Optional idOptional = instance.id();
if (idOptional.isPresent()) {
id(idOptional);
}
Optional secretIdOptional = instance.secretId();
if (secretIdOptional.isPresent()) {
secretId(secretIdOptional);
}
Optional descriptionOptional = instance.description();
if (descriptionOptional.isPresent()) {
description(descriptionOptional);
}
addAllPolicies(instance.policies());
addAllRoles(instance.roles());
addAllServiceIdentities(instance.serviceIdentities());
addAllNodeIdentities(instance.nodeIdentities());
Optional localOptional = instance.local();
if (localOptional.isPresent()) {
local(localOptional);
}
Optional expirationTimeOptional = instance.expirationTime();
if (expirationTimeOptional.isPresent()) {
expirationTime(expirationTimeOptional);
}
Optional expirationTTLOptional = instance.expirationTTL();
if (expirationTTLOptional.isPresent()) {
expirationTTL(expirationTTLOptional);
}
Optional namespaceOptional = instance.namespace();
if (namespaceOptional.isPresent()) {
namespace(namespaceOptional);
}
return this;
}
/**
* Initializes the optional value {@link Token#id() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the optional value {@link Token#id() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("AccessorID")
public final Builder id(Optional id) {
this.id = id.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Token#secretId() secretId} to secretId.
* @param secretId The value for secretId
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder secretId(String secretId) {
this.secretId = Objects.requireNonNull(secretId, "secretId");
return this;
}
/**
* Initializes the optional value {@link Token#secretId() secretId} to secretId.
* @param secretId The value for secretId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("SecretID")
public final Builder secretId(Optional secretId) {
this.secretId = secretId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Token#description() description} to description.
* @param description The value for description
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder description(String description) {
this.description = Objects.requireNonNull(description, "description");
return this;
}
/**
* Initializes the optional value {@link Token#description() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Description")
public final Builder description(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Adds one element to {@link Token#policies() policies} list.
* @param element A policies element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addPolicies(Token.PolicyLink element) {
this.policies.add(element);
return this;
}
/**
* Adds elements to {@link Token#policies() policies} list.
* @param elements An array of policies elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addPolicies(Token.PolicyLink... elements) {
this.policies.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Token#policies() policies} list.
* @param elements An iterable of policies elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Policies")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.PolicyLink.class)
public final Builder policies(Iterable extends Token.PolicyLink> elements) {
this.policies = ImmutableList.builder();
return addAllPolicies(elements);
}
/**
* Adds elements to {@link Token#policies() policies} list.
* @param elements An iterable of policies elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllPolicies(Iterable extends Token.PolicyLink> elements) {
this.policies.addAll(elements);
return this;
}
/**
* Adds one element to {@link Token#roles() roles} list.
* @param element A roles element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addRoles(Token.RoleLink element) {
this.roles.add(element);
return this;
}
/**
* Adds elements to {@link Token#roles() roles} list.
* @param elements An array of roles elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addRoles(Token.RoleLink... elements) {
this.roles.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Token#roles() roles} list.
* @param elements An iterable of roles elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Roles")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.RoleLink.class)
public final Builder roles(Iterable extends Token.RoleLink> elements) {
this.roles = ImmutableList.builder();
return addAllRoles(elements);
}
/**
* Adds elements to {@link Token#roles() roles} list.
* @param elements An iterable of roles elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllRoles(Iterable extends Token.RoleLink> elements) {
this.roles.addAll(elements);
return this;
}
/**
* Adds one element to {@link Token#serviceIdentities() serviceIdentities} list.
* @param element A serviceIdentities element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServiceIdentities(Token.ServiceIdentity element) {
this.serviceIdentities.add(element);
return this;
}
/**
* Adds elements to {@link Token#serviceIdentities() serviceIdentities} list.
* @param elements An array of serviceIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addServiceIdentities(Token.ServiceIdentity... elements) {
this.serviceIdentities.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Token#serviceIdentities() serviceIdentities} list.
* @param elements An iterable of serviceIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("ServiceIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.ServiceIdentity.class)
public final Builder serviceIdentities(Iterable extends Token.ServiceIdentity> elements) {
this.serviceIdentities = ImmutableList.builder();
return addAllServiceIdentities(elements);
}
/**
* Adds elements to {@link Token#serviceIdentities() serviceIdentities} list.
* @param elements An iterable of serviceIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllServiceIdentities(Iterable extends Token.ServiceIdentity> elements) {
this.serviceIdentities.addAll(elements);
return this;
}
/**
* Adds one element to {@link Token#nodeIdentities() nodeIdentities} list.
* @param element A nodeIdentities element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addNodeIdentities(Token.NodeIdentity element) {
this.nodeIdentities.add(element);
return this;
}
/**
* Adds elements to {@link Token#nodeIdentities() nodeIdentities} list.
* @param elements An array of nodeIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addNodeIdentities(Token.NodeIdentity... elements) {
this.nodeIdentities.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Token#nodeIdentities() nodeIdentities} list.
* @param elements An iterable of nodeIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("NodeIdentities")
@JsonDeserialize(as = ImmutableList.class, contentAs = Token.NodeIdentity.class)
public final Builder nodeIdentities(Iterable extends Token.NodeIdentity> elements) {
this.nodeIdentities = ImmutableList.builder();
return addAllNodeIdentities(elements);
}
/**
* Adds elements to {@link Token#nodeIdentities() nodeIdentities} list.
* @param elements An iterable of nodeIdentities elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllNodeIdentities(Iterable extends Token.NodeIdentity> elements) {
this.nodeIdentities.addAll(elements);
return this;
}
/**
* Initializes the optional value {@link Token#local() local} to local.
* @param local The value for local
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder local(boolean local) {
this.local = local;
return this;
}
/**
* Initializes the optional value {@link Token#local() local} to local.
* @param local The value for local
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Local")
public final Builder local(Optional local) {
this.local = local.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Token#expirationTime() expirationTime} to expirationTime.
* @param expirationTime The value for expirationTime
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder expirationTime(String expirationTime) {
this.expirationTime = Objects.requireNonNull(expirationTime, "expirationTime");
return this;
}
/**
* Initializes the optional value {@link Token#expirationTime() expirationTime} to expirationTime.
* @param expirationTime The value for expirationTime
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("ExpirationTime")
public final Builder expirationTime(Optional expirationTime) {
this.expirationTime = expirationTime.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Token#expirationTTL() expirationTTL} to expirationTTL.
* @param expirationTTL The value for expirationTTL
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder expirationTTL(String expirationTTL) {
this.expirationTTL = Objects.requireNonNull(expirationTTL, "expirationTTL");
return this;
}
/**
* Initializes the optional value {@link Token#expirationTTL() expirationTTL} to expirationTTL.
* @param expirationTTL The value for expirationTTL
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("ExpirationTTL")
public final Builder expirationTTL(Optional expirationTTL) {
this.expirationTTL = expirationTTL.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Token#namespace() namespace} to namespace.
* @param namespace The value for namespace
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder namespace(String namespace) {
this.namespace = Objects.requireNonNull(namespace, "namespace");
return this;
}
/**
* Initializes the optional value {@link Token#namespace() namespace} to namespace.
* @param namespace The value for namespace
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Namespace")
public final Builder namespace(Optional namespace) {
this.namespace = namespace.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableToken ImmutableToken}.
* @return An immutable instance of Token
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableToken build() {
return new ImmutableToken(
id,
secretId,
description,
policies.build(),
roles.build(),
serviceIdentities.build(),
nodeIdentities.build(),
local,
expirationTime,
expirationTTL,
namespace);
}
}
}