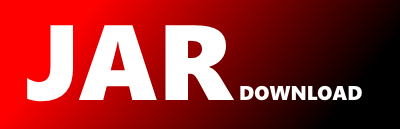
org.kiwiproject.consul.model.agent.ImmutableRegistration Maven / Gradle / Ivy
package org.kiwiproject.consul.model.agent;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
import org.kiwiproject.consul.model.catalog.ServiceWeights;
/**
* Immutable implementation of {@link Registration}.
*
* Use the builder to create immutable instances:
* {@code ImmutableRegistration.builder()}.
*/
@Generated(from = "Registration", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableRegistration extends Registration {
private final String name;
private final String id;
private final @Nullable String address;
private final @Nullable Integer port;
private final @Nullable Registration.RegCheck check;
private final ImmutableList checks;
private final ImmutableList tags;
private final ImmutableMap meta;
private final @Nullable Boolean enableTagOverride;
private final @Nullable ServiceWeights serviceWeights;
private ImmutableRegistration(
String name,
String id,
@Nullable String address,
@Nullable Integer port,
@Nullable Registration.RegCheck check,
ImmutableList checks,
ImmutableList tags,
ImmutableMap meta,
@Nullable Boolean enableTagOverride,
@Nullable ServiceWeights serviceWeights) {
this.name = name;
this.id = id;
this.address = address;
this.port = port;
this.check = check;
this.checks = checks;
this.tags = tags;
this.meta = meta;
this.enableTagOverride = enableTagOverride;
this.serviceWeights = serviceWeights;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("Name")
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("Id")
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code address} attribute
*/
@JsonProperty("Address")
@Override
public Optional getAddress() {
return Optional.ofNullable(address);
}
/**
* @return The value of the {@code port} attribute
*/
@JsonProperty("Port")
@Override
public Optional getPort() {
return Optional.ofNullable(port);
}
/**
* @return The value of the {@code check} attribute
*/
@JsonProperty("Check")
@Override
public Optional getCheck() {
return Optional.ofNullable(check);
}
/**
* @return The value of the {@code checks} attribute
*/
@JsonProperty("Checks")
@Override
public ImmutableList getChecks() {
return checks;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("Tags")
@Override
public ImmutableList getTags() {
return tags;
}
/**
* @return The value of the {@code meta} attribute
*/
@JsonProperty("Meta")
@Override
public ImmutableMap getMeta() {
return meta;
}
/**
* @return The value of the {@code enableTagOverride} attribute
*/
@JsonProperty("EnableTagOverride")
@Override
public Optional getEnableTagOverride() {
return Optional.ofNullable(enableTagOverride);
}
/**
* @return The value of the {@code serviceWeights} attribute
*/
@JsonProperty("Weights")
@Override
public Optional getServiceWeights() {
return Optional.ofNullable(serviceWeights);
}
/**
* Copy the current immutable object by setting a value for the {@link Registration#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableRegistration withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableRegistration(
newValue,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a value for the {@link Registration#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableRegistration withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new ImmutableRegistration(
this.name,
newValue,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Registration#getAddress() address} attribute.
* @param value The value for address
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withAddress(String value) {
String newValue = Objects.requireNonNull(value, "address");
if (Objects.equals(this.address, newValue)) return this;
return new ImmutableRegistration(
this.name,
this.id,
newValue,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Registration#getAddress() address} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for address
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withAddress(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.address, value)) return this;
return new ImmutableRegistration(
this.name,
this.id,
value,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Registration#getPort() port} attribute.
* @param value The value for port
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withPort(int value) {
@Nullable Integer newValue = value;
if (Objects.equals(this.port, newValue)) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
newValue,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Registration#getPort() port} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for port
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withPort(Optional optional) {
@Nullable Integer value = optional.orElse(null);
if (Objects.equals(this.port, value)) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
value,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Registration#getCheck() check} attribute.
* @param value The value for check
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withCheck(Registration.RegCheck value) {
Registration.RegCheck newValue = Objects.requireNonNull(value, "check");
if (this.check == newValue) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
newValue,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Registration#getCheck() check} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for check
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableRegistration withCheck(Optional extends Registration.RegCheck> optional) {
@Nullable Registration.RegCheck value = optional.orElse(null);
if (this.check == value) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
value,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Registration#getChecks() checks}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withChecks(Registration.RegCheck... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
newValue,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Registration#getChecks() checks}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of checks elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withChecks(Iterable extends Registration.RegCheck> elements) {
if (this.checks == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
newValue,
this.tags,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Registration#getTags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withTags(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
newValue,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Registration#getTags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withTags(Iterable elements) {
if (this.tags == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
newValue,
this.meta,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by replacing the {@link Registration#getMeta() meta} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the meta map
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withMeta(Map entries) {
if (this.meta == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
newValue,
this.enableTagOverride,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Registration#getEnableTagOverride() enableTagOverride} attribute.
* @param value The value for enableTagOverride
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withEnableTagOverride(boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.enableTagOverride, newValue)) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
newValue,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Registration#getEnableTagOverride() enableTagOverride} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for enableTagOverride
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withEnableTagOverride(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.enableTagOverride, value)) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
value,
this.serviceWeights);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Registration#getServiceWeights() serviceWeights} attribute.
* @param value The value for serviceWeights
* @return A modified copy of {@code this} object
*/
public final ImmutableRegistration withServiceWeights(ServiceWeights value) {
ServiceWeights newValue = Objects.requireNonNull(value, "serviceWeights");
if (this.serviceWeights == newValue) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Registration#getServiceWeights() serviceWeights} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for serviceWeights
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableRegistration withServiceWeights(Optional extends ServiceWeights> optional) {
@Nullable ServiceWeights value = optional.orElse(null);
if (this.serviceWeights == value) return this;
return new ImmutableRegistration(
this.name,
this.id,
this.address,
this.port,
this.check,
this.checks,
this.tags,
this.meta,
this.enableTagOverride,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableRegistration} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableRegistration
&& equalTo(0, (ImmutableRegistration) another);
}
private boolean equalTo(int synthetic, ImmutableRegistration another) {
return name.equals(another.name)
&& id.equals(another.id)
&& Objects.equals(address, another.address)
&& Objects.equals(port, another.port)
&& Objects.equals(check, another.check)
&& checks.equals(another.checks)
&& tags.equals(another.tags)
&& meta.equals(another.meta)
&& Objects.equals(enableTagOverride, another.enableTagOverride)
&& Objects.equals(serviceWeights, another.serviceWeights);
}
/**
* Computes a hash code from attributes: {@code name}, {@code id}, {@code address}, {@code port}, {@code check}, {@code checks}, {@code tags}, {@code meta}, {@code enableTagOverride}, {@code serviceWeights}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + id.hashCode();
h += (h << 5) + Objects.hashCode(address);
h += (h << 5) + Objects.hashCode(port);
h += (h << 5) + Objects.hashCode(check);
h += (h << 5) + checks.hashCode();
h += (h << 5) + tags.hashCode();
h += (h << 5) + meta.hashCode();
h += (h << 5) + Objects.hashCode(enableTagOverride);
h += (h << 5) + Objects.hashCode(serviceWeights);
return h;
}
/**
* Prints the immutable value {@code Registration} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Registration")
.omitNullValues()
.add("name", name)
.add("id", id)
.add("address", address)
.add("port", port)
.add("check", check)
.add("checks", checks)
.add("tags", tags)
.add("meta", meta)
.add("enableTagOverride", enableTagOverride)
.add("serviceWeights", serviceWeights)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "Registration", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends Registration {
@Nullable String name;
@Nullable String id;
@Nullable Optional address = Optional.empty();
@Nullable Optional port = Optional.empty();
@Nullable Optional check = Optional.empty();
@Nullable List checks = ImmutableList.of();
@Nullable List tags = ImmutableList.of();
@Nullable Map meta = ImmutableMap.of();
@Nullable Optional enableTagOverride = Optional.empty();
@Nullable Optional serviceWeights = Optional.empty();
@JsonProperty("Name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("Id")
public void setId(String id) {
this.id = id;
}
@JsonProperty("Address")
public void setAddress(Optional address) {
this.address = address;
}
@JsonProperty("Port")
public void setPort(Optional port) {
this.port = port;
}
@JsonProperty("Check")
public void setCheck(Optional check) {
this.check = check;
}
@JsonProperty("Checks")
public void setChecks(List checks) {
this.checks = checks;
}
@JsonProperty("Tags")
public void setTags(List tags) {
this.tags = tags;
}
@JsonProperty("Meta")
public void setMeta(Map meta) {
this.meta = meta;
}
@JsonProperty("EnableTagOverride")
public void setEnableTagOverride(Optional enableTagOverride) {
this.enableTagOverride = enableTagOverride;
}
@JsonProperty("Weights")
public void setServiceWeights(Optional serviceWeights) {
this.serviceWeights = serviceWeights;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public Optional getAddress() { throw new UnsupportedOperationException(); }
@Override
public Optional getPort() { throw new UnsupportedOperationException(); }
@Override
public Optional getCheck() { throw new UnsupportedOperationException(); }
@Override
public List getChecks() { throw new UnsupportedOperationException(); }
@Override
public List getTags() { throw new UnsupportedOperationException(); }
@Override
public Map getMeta() { throw new UnsupportedOperationException(); }
@Override
public Optional getEnableTagOverride() { throw new UnsupportedOperationException(); }
@Override
public Optional getServiceWeights() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableRegistration fromJson(Json json) {
ImmutableRegistration.Builder builder = ImmutableRegistration.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.id != null) {
builder.id(json.id);
}
if (json.address != null) {
builder.address(json.address);
}
if (json.port != null) {
builder.port(json.port);
}
if (json.check != null) {
builder.check(json.check);
}
if (json.checks != null) {
builder.addAllChecks(json.checks);
}
if (json.tags != null) {
builder.addAllTags(json.tags);
}
if (json.meta != null) {
builder.putAllMeta(json.meta);
}
if (json.enableTagOverride != null) {
builder.enableTagOverride(json.enableTagOverride);
}
if (json.serviceWeights != null) {
builder.serviceWeights(json.serviceWeights);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Registration} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Registration instance
*/
public static ImmutableRegistration copyOf(Registration instance) {
if (instance instanceof ImmutableRegistration) {
return (ImmutableRegistration) instance;
}
return ImmutableRegistration.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableRegistration ImmutableRegistration}.
*
* ImmutableRegistration.builder()
* .name(String) // required {@link Registration#getName() name}
* .id(String) // required {@link Registration#getId() id}
* .address(String) // optional {@link Registration#getAddress() address}
* .port(Integer) // optional {@link Registration#getPort() port}
* .check(org.kiwiproject.consul.model.agent.Registration.RegCheck) // optional {@link Registration#getCheck() check}
* .addChecks|addAllChecks(org.kiwiproject.consul.model.agent.Registration.RegCheck) // {@link Registration#getChecks() checks} elements
* .addTags|addAllTags(String) // {@link Registration#getTags() tags} elements
* .putMeta|putAllMeta(String => String) // {@link Registration#getMeta() meta} mappings
* .enableTagOverride(Boolean) // optional {@link Registration#getEnableTagOverride() enableTagOverride}
* .serviceWeights(org.kiwiproject.consul.model.catalog.ServiceWeights) // optional {@link Registration#getServiceWeights() serviceWeights}
* .build();
*
* @return A new ImmutableRegistration builder
*/
public static ImmutableRegistration.Builder builder() {
return new ImmutableRegistration.Builder();
}
/**
* Builds instances of type {@link ImmutableRegistration ImmutableRegistration}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Registration", generator = "Immutables")
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_ID = 0x2L;
private long initBits = 0x3L;
private @Nullable String name;
private @Nullable String id;
private @Nullable String address;
private @Nullable Integer port;
private @Nullable Registration.RegCheck check;
private ImmutableList.Builder checks = ImmutableList.builder();
private ImmutableList.Builder tags = ImmutableList.builder();
private ImmutableMap.Builder meta = ImmutableMap.builder();
private @Nullable Boolean enableTagOverride;
private @Nullable ServiceWeights serviceWeights;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Registration} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Registration instance) {
Objects.requireNonNull(instance, "instance");
name(instance.getName());
id(instance.getId());
Optional addressOptional = instance.getAddress();
if (addressOptional.isPresent()) {
address(addressOptional);
}
Optional portOptional = instance.getPort();
if (portOptional.isPresent()) {
port(portOptional);
}
Optional checkOptional = instance.getCheck();
if (checkOptional.isPresent()) {
check(checkOptional);
}
addAllChecks(instance.getChecks());
addAllTags(instance.getTags());
putAllMeta(instance.getMeta());
Optional enableTagOverrideOptional = instance.getEnableTagOverride();
if (enableTagOverrideOptional.isPresent()) {
enableTagOverride(enableTagOverrideOptional);
}
Optional serviceWeightsOptional = instance.getServiceWeights();
if (serviceWeightsOptional.isPresent()) {
serviceWeights(serviceWeightsOptional);
}
return this;
}
/**
* Initializes the value for the {@link Registration#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Name")
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link Registration#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Id")
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the optional value {@link Registration#getAddress() address} to address.
* @param address The value for address
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder address(String address) {
this.address = Objects.requireNonNull(address, "address");
return this;
}
/**
* Initializes the optional value {@link Registration#getAddress() address} to address.
* @param address The value for address
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Address")
public final Builder address(Optional address) {
this.address = address.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Registration#getPort() port} to port.
* @param port The value for port
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder port(int port) {
this.port = port;
return this;
}
/**
* Initializes the optional value {@link Registration#getPort() port} to port.
* @param port The value for port
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Port")
public final Builder port(Optional port) {
this.port = port.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Registration#getCheck() check} to check.
* @param check The value for check
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder check(Registration.RegCheck check) {
this.check = Objects.requireNonNull(check, "check");
return this;
}
/**
* Initializes the optional value {@link Registration#getCheck() check} to check.
* @param check The value for check
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Check")
public final Builder check(Optional extends Registration.RegCheck> check) {
this.check = check.orElse(null);
return this;
}
/**
* Adds one element to {@link Registration#getChecks() checks} list.
* @param element A checks element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addChecks(Registration.RegCheck element) {
this.checks.add(element);
return this;
}
/**
* Adds elements to {@link Registration#getChecks() checks} list.
* @param elements An array of checks elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addChecks(Registration.RegCheck... elements) {
this.checks.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Registration#getChecks() checks} list.
* @param elements An iterable of checks elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Checks")
public final Builder checks(Iterable extends Registration.RegCheck> elements) {
this.checks = ImmutableList.builder();
return addAllChecks(elements);
}
/**
* Adds elements to {@link Registration#getChecks() checks} list.
* @param elements An iterable of checks elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllChecks(Iterable extends Registration.RegCheck> elements) {
this.checks.addAll(elements);
return this;
}
/**
* Adds one element to {@link Registration#getTags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(element);
return this;
}
/**
* Adds elements to {@link Registration#getTags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
this.tags.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link Registration#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Tags")
public final Builder tags(Iterable elements) {
this.tags = ImmutableList.builder();
return addAllTags(elements);
}
/**
* Adds elements to {@link Registration#getTags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
this.tags.addAll(elements);
return this;
}
/**
* Put one entry to the {@link Registration#getMeta() meta} map.
* @param key The key in the meta map
* @param value The associated value in the meta map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putMeta(String key, String value) {
this.meta.put(key, value);
return this;
}
/**
* Put one entry to the {@link Registration#getMeta() meta} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putMeta(Map.Entry entry) {
this.meta.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link Registration#getMeta() meta} map. Nulls are not permitted
* @param entries The entries that will be added to the meta map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Meta")
public final Builder meta(Map entries) {
this.meta = ImmutableMap.builder();
return putAllMeta(entries);
}
/**
* Put all mappings from the specified map as entries to {@link Registration#getMeta() meta} map. Nulls are not permitted
* @param entries The entries that will be added to the meta map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllMeta(Map entries) {
this.meta.putAll(entries);
return this;
}
/**
* Initializes the optional value {@link Registration#getEnableTagOverride() enableTagOverride} to enableTagOverride.
* @param enableTagOverride The value for enableTagOverride
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder enableTagOverride(boolean enableTagOverride) {
this.enableTagOverride = enableTagOverride;
return this;
}
/**
* Initializes the optional value {@link Registration#getEnableTagOverride() enableTagOverride} to enableTagOverride.
* @param enableTagOverride The value for enableTagOverride
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("EnableTagOverride")
public final Builder enableTagOverride(Optional enableTagOverride) {
this.enableTagOverride = enableTagOverride.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Registration#getServiceWeights() serviceWeights} to serviceWeights.
* @param serviceWeights The value for serviceWeights
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder serviceWeights(ServiceWeights serviceWeights) {
this.serviceWeights = Objects.requireNonNull(serviceWeights, "serviceWeights");
return this;
}
/**
* Initializes the optional value {@link Registration#getServiceWeights() serviceWeights} to serviceWeights.
* @param serviceWeights The value for serviceWeights
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Weights")
public final Builder serviceWeights(Optional extends ServiceWeights> serviceWeights) {
this.serviceWeights = serviceWeights.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableRegistration ImmutableRegistration}.
* @return An immutable instance of Registration
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableRegistration build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableRegistration(
name,
id,
address,
port,
check,
checks.build(),
tags.build(),
meta.build(),
enableTagOverride,
serviceWeights);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
return "Cannot build Registration, some of required attributes are not set " + attributes;
}
}
}