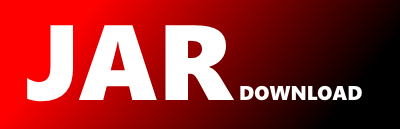
org.kiwiproject.consul.model.kv.ImmutableTxResponse Maven / Gradle / Ivy
package org.kiwiproject.consul.model.kv;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link TxResponse}.
*
* Use the builder to create immutable instances:
* {@code ImmutableTxResponse.builder()}.
*/
@Generated(from = "TxResponse", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableTxResponse extends TxResponse {
private final ImmutableList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy