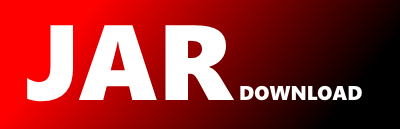
org.kiwiproject.consul.option.ImmutableEventOptions Maven / Gradle / Ivy
package org.kiwiproject.consul.option;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link EventOptions}.
*
* Use the builder to create immutable instances:
* {@code ImmutableEventOptions.builder()}.
*/
@Generated(from = "EventOptions", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableEventOptions extends EventOptions {
private final ImmutableList toQueryParameters;
private final ImmutableMap toHeaders;
private final @Nullable String datacenter;
private final @Nullable String nodeFilter;
private final @Nullable String serviceFilter;
private final @Nullable String tagFilter;
private ImmutableEventOptions(
ImmutableList toQueryParameters,
ImmutableMap toHeaders,
@Nullable String datacenter,
@Nullable String nodeFilter,
@Nullable String serviceFilter,
@Nullable String tagFilter) {
this.toQueryParameters = toQueryParameters;
this.toHeaders = toHeaders;
this.datacenter = datacenter;
this.nodeFilter = nodeFilter;
this.serviceFilter = serviceFilter;
this.tagFilter = tagFilter;
}
/**
* @return The value of the {@code toQueryParameters} attribute
*/
@Override
public ImmutableList toQueryParameters() {
return toQueryParameters;
}
/**
* @return The value of the {@code toHeaders} attribute
*/
@Override
public ImmutableMap toHeaders() {
return toHeaders;
}
/**
* @return The value of the {@code datacenter} attribute
*/
@Override
public Optional getDatacenter() {
return Optional.ofNullable(datacenter);
}
/**
* @return The value of the {@code nodeFilter} attribute
*/
@Override
public Optional getNodeFilter() {
return Optional.ofNullable(nodeFilter);
}
/**
* @return The value of the {@code serviceFilter} attribute
*/
@Override
public Optional getServiceFilter() {
return Optional.ofNullable(serviceFilter);
}
/**
* @return The value of the {@code tagFilter} attribute
*/
@Override
public Optional getTagFilter() {
return Optional.ofNullable(tagFilter);
}
/**
* Copy the current immutable object with elements that replace the content of {@link EventOptions#toQueryParameters() toQueryParameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withToQueryParameters(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableEventOptions(newValue, this.toHeaders, this.datacenter, this.nodeFilter, this.serviceFilter, this.tagFilter);
}
/**
* Copy the current immutable object with elements that replace the content of {@link EventOptions#toQueryParameters() toQueryParameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of toQueryParameters elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withToQueryParameters(Iterable elements) {
if (this.toQueryParameters == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableEventOptions(newValue, this.toHeaders, this.datacenter, this.nodeFilter, this.serviceFilter, this.tagFilter);
}
/**
* Copy the current immutable object by replacing the {@link EventOptions#toHeaders() toHeaders} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the toHeaders map
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withToHeaders(Map entries) {
if (this.toHeaders == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableEventOptions(
this.toQueryParameters,
newValue,
this.datacenter,
this.nodeFilter,
this.serviceFilter,
this.tagFilter);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EventOptions#getDatacenter() datacenter} attribute.
* @param value The value for datacenter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withDatacenter(String value) {
String newValue = Objects.requireNonNull(value, "datacenter");
if (Objects.equals(this.datacenter, newValue)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
newValue,
this.nodeFilter,
this.serviceFilter,
this.tagFilter);
}
/**
* Copy the current immutable object by setting an optional value for the {@link EventOptions#getDatacenter() datacenter} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for datacenter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withDatacenter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.datacenter, value)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
value,
this.nodeFilter,
this.serviceFilter,
this.tagFilter);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EventOptions#getNodeFilter() nodeFilter} attribute.
* @param value The value for nodeFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withNodeFilter(String value) {
String newValue = Objects.requireNonNull(value, "nodeFilter");
if (Objects.equals(this.nodeFilter, newValue)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
this.datacenter,
newValue,
this.serviceFilter,
this.tagFilter);
}
/**
* Copy the current immutable object by setting an optional value for the {@link EventOptions#getNodeFilter() nodeFilter} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for nodeFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withNodeFilter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.nodeFilter, value)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
this.datacenter,
value,
this.serviceFilter,
this.tagFilter);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EventOptions#getServiceFilter() serviceFilter} attribute.
* @param value The value for serviceFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withServiceFilter(String value) {
String newValue = Objects.requireNonNull(value, "serviceFilter");
if (Objects.equals(this.serviceFilter, newValue)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
this.datacenter,
this.nodeFilter,
newValue,
this.tagFilter);
}
/**
* Copy the current immutable object by setting an optional value for the {@link EventOptions#getServiceFilter() serviceFilter} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for serviceFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withServiceFilter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.serviceFilter, value)) return this;
return new ImmutableEventOptions(this.toQueryParameters, this.toHeaders, this.datacenter, this.nodeFilter, value, this.tagFilter);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link EventOptions#getTagFilter() tagFilter} attribute.
* @param value The value for tagFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withTagFilter(String value) {
String newValue = Objects.requireNonNull(value, "tagFilter");
if (Objects.equals(this.tagFilter, newValue)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
this.datacenter,
this.nodeFilter,
this.serviceFilter,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link EventOptions#getTagFilter() tagFilter} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for tagFilter
* @return A modified copy of {@code this} object
*/
public final ImmutableEventOptions withTagFilter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.tagFilter, value)) return this;
return new ImmutableEventOptions(
this.toQueryParameters,
this.toHeaders,
this.datacenter,
this.nodeFilter,
this.serviceFilter,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableEventOptions} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableEventOptions
&& equalTo(0, (ImmutableEventOptions) another);
}
private boolean equalTo(int synthetic, ImmutableEventOptions another) {
return toQueryParameters.equals(another.toQueryParameters)
&& toHeaders.equals(another.toHeaders)
&& Objects.equals(datacenter, another.datacenter)
&& Objects.equals(nodeFilter, another.nodeFilter)
&& Objects.equals(serviceFilter, another.serviceFilter)
&& Objects.equals(tagFilter, another.tagFilter);
}
/**
* Computes a hash code from attributes: {@code toQueryParameters}, {@code toHeaders}, {@code datacenter}, {@code nodeFilter}, {@code serviceFilter}, {@code tagFilter}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + toQueryParameters.hashCode();
h += (h << 5) + toHeaders.hashCode();
h += (h << 5) + Objects.hashCode(datacenter);
h += (h << 5) + Objects.hashCode(nodeFilter);
h += (h << 5) + Objects.hashCode(serviceFilter);
h += (h << 5) + Objects.hashCode(tagFilter);
return h;
}
/**
* Prints the immutable value {@code EventOptions} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("EventOptions")
.omitNullValues()
.add("toQueryParameters", toQueryParameters)
.add("toHeaders", toHeaders)
.add("datacenter", datacenter)
.add("nodeFilter", nodeFilter)
.add("serviceFilter", serviceFilter)
.add("tagFilter", tagFilter)
.toString();
}
/**
* Creates an immutable copy of a {@link EventOptions} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable EventOptions instance
*/
public static ImmutableEventOptions copyOf(EventOptions instance) {
if (instance instanceof ImmutableEventOptions) {
return (ImmutableEventOptions) instance;
}
return ImmutableEventOptions.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableEventOptions ImmutableEventOptions}.
*
* ImmutableEventOptions.builder()
* .addToQueryParameters|addAllToQueryParameters(String) // {@link EventOptions#toQueryParameters() toQueryParameters} elements
* .putToHeaders|putAllToHeaders(String => String) // {@link EventOptions#toHeaders() toHeaders} mappings
* .datacenter(String) // optional {@link EventOptions#getDatacenter() datacenter}
* .nodeFilter(String) // optional {@link EventOptions#getNodeFilter() nodeFilter}
* .serviceFilter(String) // optional {@link EventOptions#getServiceFilter() serviceFilter}
* .tagFilter(String) // optional {@link EventOptions#getTagFilter() tagFilter}
* .build();
*
* @return A new ImmutableEventOptions builder
*/
public static ImmutableEventOptions.Builder builder() {
return new ImmutableEventOptions.Builder();
}
/**
* Builds instances of type {@link ImmutableEventOptions ImmutableEventOptions}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "EventOptions", generator = "Immutables")
public static final class Builder {
private ImmutableList.Builder toQueryParameters = ImmutableList.builder();
private ImmutableMap.Builder toHeaders = ImmutableMap.builder();
private @Nullable String datacenter;
private @Nullable String nodeFilter;
private @Nullable String serviceFilter;
private @Nullable String tagFilter;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.EventOptions} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(EventOptions instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.ParamAdder} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ParamAdder instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
if (object instanceof EventOptions) {
EventOptions instance = (EventOptions) object;
Optional serviceFilterOptional = instance.getServiceFilter();
if (serviceFilterOptional.isPresent()) {
serviceFilter(serviceFilterOptional);
}
Optional nodeFilterOptional = instance.getNodeFilter();
if (nodeFilterOptional.isPresent()) {
nodeFilter(nodeFilterOptional);
}
Optional tagFilterOptional = instance.getTagFilter();
if (tagFilterOptional.isPresent()) {
tagFilter(tagFilterOptional);
}
Optional datacenterOptional = instance.getDatacenter();
if (datacenterOptional.isPresent()) {
datacenter(datacenterOptional);
}
}
if (object instanceof ParamAdder) {
ParamAdder instance = (ParamAdder) object;
addAllToQueryParameters(instance.toQueryParameters());
putAllToHeaders(instance.toHeaders());
}
}
/**
* Adds one element to {@link EventOptions#toQueryParameters() toQueryParameters} list.
* @param element A toQueryParameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String element) {
this.toQueryParameters.add(element);
return this;
}
/**
* Adds elements to {@link EventOptions#toQueryParameters() toQueryParameters} list.
* @param elements An array of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String... elements) {
this.toQueryParameters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link EventOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toQueryParameters(Iterable elements) {
this.toQueryParameters = ImmutableList.builder();
return addAllToQueryParameters(elements);
}
/**
* Adds elements to {@link EventOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllToQueryParameters(Iterable elements) {
this.toQueryParameters.addAll(elements);
return this;
}
/**
* Put one entry to the {@link EventOptions#toHeaders() toHeaders} map.
* @param key The key in the toHeaders map
* @param value The associated value in the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(String key, String value) {
this.toHeaders.put(key, value);
return this;
}
/**
* Put one entry to the {@link EventOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(Map.Entry entry) {
this.toHeaders.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link EventOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toHeaders(Map entries) {
this.toHeaders = ImmutableMap.builder();
return putAllToHeaders(entries);
}
/**
* Put all mappings from the specified map as entries to {@link EventOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllToHeaders(Map entries) {
this.toHeaders.putAll(entries);
return this;
}
/**
* Initializes the optional value {@link EventOptions#getDatacenter() datacenter} to datacenter.
* @param datacenter The value for datacenter
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder datacenter(String datacenter) {
this.datacenter = Objects.requireNonNull(datacenter, "datacenter");
return this;
}
/**
* Initializes the optional value {@link EventOptions#getDatacenter() datacenter} to datacenter.
* @param datacenter The value for datacenter
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder datacenter(Optional datacenter) {
this.datacenter = datacenter.orElse(null);
return this;
}
/**
* Initializes the optional value {@link EventOptions#getNodeFilter() nodeFilter} to nodeFilter.
* @param nodeFilter The value for nodeFilter
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder nodeFilter(String nodeFilter) {
this.nodeFilter = Objects.requireNonNull(nodeFilter, "nodeFilter");
return this;
}
/**
* Initializes the optional value {@link EventOptions#getNodeFilter() nodeFilter} to nodeFilter.
* @param nodeFilter The value for nodeFilter
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder nodeFilter(Optional nodeFilter) {
this.nodeFilter = nodeFilter.orElse(null);
return this;
}
/**
* Initializes the optional value {@link EventOptions#getServiceFilter() serviceFilter} to serviceFilter.
* @param serviceFilter The value for serviceFilter
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder serviceFilter(String serviceFilter) {
this.serviceFilter = Objects.requireNonNull(serviceFilter, "serviceFilter");
return this;
}
/**
* Initializes the optional value {@link EventOptions#getServiceFilter() serviceFilter} to serviceFilter.
* @param serviceFilter The value for serviceFilter
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder serviceFilter(Optional serviceFilter) {
this.serviceFilter = serviceFilter.orElse(null);
return this;
}
/**
* Initializes the optional value {@link EventOptions#getTagFilter() tagFilter} to tagFilter.
* @param tagFilter The value for tagFilter
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder tagFilter(String tagFilter) {
this.tagFilter = Objects.requireNonNull(tagFilter, "tagFilter");
return this;
}
/**
* Initializes the optional value {@link EventOptions#getTagFilter() tagFilter} to tagFilter.
* @param tagFilter The value for tagFilter
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder tagFilter(Optional tagFilter) {
this.tagFilter = tagFilter.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableEventOptions ImmutableEventOptions}.
* @return An immutable instance of EventOptions
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableEventOptions build() {
return new ImmutableEventOptions(
toQueryParameters.build(),
toHeaders.build(),
datacenter,
nodeFilter,
serviceFilter,
tagFilter);
}
}
}