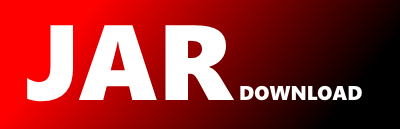
org.kiwiproject.consul.option.ImmutableTokenQueryOptions Maven / Gradle / Ivy
package org.kiwiproject.consul.option;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link TokenQueryOptions}.
*
* Use the builder to create immutable instances:
* {@code ImmutableTokenQueryOptions.builder()}.
*/
@Generated(from = "TokenQueryOptions", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableTokenQueryOptions extends TokenQueryOptions {
private final ImmutableList toQueryParameters;
private final ImmutableMap toHeaders;
private final @Nullable String policy;
private final @Nullable String role;
private final @Nullable String authMethod;
private final @Nullable String authMethodNamespace;
private final @Nullable String namespace;
private ImmutableTokenQueryOptions(
ImmutableList toQueryParameters,
ImmutableMap toHeaders,
@Nullable String policy,
@Nullable String role,
@Nullable String authMethod,
@Nullable String authMethodNamespace,
@Nullable String namespace) {
this.toQueryParameters = toQueryParameters;
this.toHeaders = toHeaders;
this.policy = policy;
this.role = role;
this.authMethod = authMethod;
this.authMethodNamespace = authMethodNamespace;
this.namespace = namespace;
}
/**
* @return The value of the {@code toQueryParameters} attribute
*/
@Override
public ImmutableList toQueryParameters() {
return toQueryParameters;
}
/**
* @return The value of the {@code toHeaders} attribute
*/
@Override
public ImmutableMap toHeaders() {
return toHeaders;
}
/**
* @return The value of the {@code policy} attribute
*/
@Override
public Optional getPolicy() {
return Optional.ofNullable(policy);
}
/**
* @return The value of the {@code role} attribute
*/
@Override
public Optional getRole() {
return Optional.ofNullable(role);
}
/**
* @return The value of the {@code authMethod} attribute
*/
@Override
public Optional getAuthMethod() {
return Optional.ofNullable(authMethod);
}
/**
* @return The value of the {@code authMethodNamespace} attribute
*/
@Override
public Optional getAuthMethodNamespace() {
return Optional.ofNullable(authMethodNamespace);
}
/**
* @return The value of the {@code namespace} attribute
*/
@Override
public Optional getNamespace() {
return Optional.ofNullable(namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TokenQueryOptions#toQueryParameters() toQueryParameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withToQueryParameters(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableTokenQueryOptions(
newValue,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TokenQueryOptions#toQueryParameters() toQueryParameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of toQueryParameters elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withToQueryParameters(Iterable elements) {
if (this.toQueryParameters == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableTokenQueryOptions(
newValue,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by replacing the {@link TokenQueryOptions#toHeaders() toHeaders} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the toHeaders map
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withToHeaders(Map entries) {
if (this.toHeaders == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
newValue,
this.policy,
this.role,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TokenQueryOptions#getPolicy() policy} attribute.
* @param value The value for policy
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withPolicy(String value) {
String newValue = Objects.requireNonNull(value, "policy");
if (Objects.equals(this.policy, newValue)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
newValue,
this.role,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TokenQueryOptions#getPolicy() policy} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for policy
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withPolicy(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.policy, value)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
value,
this.role,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TokenQueryOptions#getRole() role} attribute.
* @param value The value for role
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withRole(String value) {
String newValue = Objects.requireNonNull(value, "role");
if (Objects.equals(this.role, newValue)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
newValue,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TokenQueryOptions#getRole() role} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for role
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withRole(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.role, value)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
value,
this.authMethod,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TokenQueryOptions#getAuthMethod() authMethod} attribute.
* @param value The value for authMethod
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withAuthMethod(String value) {
String newValue = Objects.requireNonNull(value, "authMethod");
if (Objects.equals(this.authMethod, newValue)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
newValue,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TokenQueryOptions#getAuthMethod() authMethod} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for authMethod
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withAuthMethod(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.authMethod, value)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
value,
this.authMethodNamespace,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TokenQueryOptions#getAuthMethodNamespace() authMethodNamespace} attribute.
* @param value The value for authMethodNamespace
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withAuthMethodNamespace(String value) {
String newValue = Objects.requireNonNull(value, "authMethodNamespace");
if (Objects.equals(this.authMethodNamespace, newValue)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
newValue,
this.namespace);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TokenQueryOptions#getAuthMethodNamespace() authMethodNamespace} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for authMethodNamespace
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withAuthMethodNamespace(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.authMethodNamespace, value)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
value,
this.namespace);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TokenQueryOptions#getNamespace() namespace} attribute.
* @param value The value for namespace
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withNamespace(String value) {
String newValue = Objects.requireNonNull(value, "namespace");
if (Objects.equals(this.namespace, newValue)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
this.authMethodNamespace,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TokenQueryOptions#getNamespace() namespace} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for namespace
* @return A modified copy of {@code this} object
*/
public final ImmutableTokenQueryOptions withNamespace(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.namespace, value)) return this;
return new ImmutableTokenQueryOptions(
this.toQueryParameters,
this.toHeaders,
this.policy,
this.role,
this.authMethod,
this.authMethodNamespace,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableTokenQueryOptions} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableTokenQueryOptions
&& equalTo(0, (ImmutableTokenQueryOptions) another);
}
private boolean equalTo(int synthetic, ImmutableTokenQueryOptions another) {
return toQueryParameters.equals(another.toQueryParameters)
&& toHeaders.equals(another.toHeaders)
&& Objects.equals(policy, another.policy)
&& Objects.equals(role, another.role)
&& Objects.equals(authMethod, another.authMethod)
&& Objects.equals(authMethodNamespace, another.authMethodNamespace)
&& Objects.equals(namespace, another.namespace);
}
/**
* Computes a hash code from attributes: {@code toQueryParameters}, {@code toHeaders}, {@code policy}, {@code role}, {@code authMethod}, {@code authMethodNamespace}, {@code namespace}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + toQueryParameters.hashCode();
h += (h << 5) + toHeaders.hashCode();
h += (h << 5) + Objects.hashCode(policy);
h += (h << 5) + Objects.hashCode(role);
h += (h << 5) + Objects.hashCode(authMethod);
h += (h << 5) + Objects.hashCode(authMethodNamespace);
h += (h << 5) + Objects.hashCode(namespace);
return h;
}
/**
* Prints the immutable value {@code TokenQueryOptions} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("TokenQueryOptions")
.omitNullValues()
.add("toQueryParameters", toQueryParameters)
.add("toHeaders", toHeaders)
.add("policy", policy)
.add("role", role)
.add("authMethod", authMethod)
.add("authMethodNamespace", authMethodNamespace)
.add("namespace", namespace)
.toString();
}
/**
* Creates an immutable copy of a {@link TokenQueryOptions} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TokenQueryOptions instance
*/
public static ImmutableTokenQueryOptions copyOf(TokenQueryOptions instance) {
if (instance instanceof ImmutableTokenQueryOptions) {
return (ImmutableTokenQueryOptions) instance;
}
return ImmutableTokenQueryOptions.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableTokenQueryOptions ImmutableTokenQueryOptions}.
*
* ImmutableTokenQueryOptions.builder()
* .addToQueryParameters|addAllToQueryParameters(String) // {@link TokenQueryOptions#toQueryParameters() toQueryParameters} elements
* .putToHeaders|putAllToHeaders(String => String) // {@link TokenQueryOptions#toHeaders() toHeaders} mappings
* .policy(String) // optional {@link TokenQueryOptions#getPolicy() policy}
* .role(String) // optional {@link TokenQueryOptions#getRole() role}
* .authMethod(String) // optional {@link TokenQueryOptions#getAuthMethod() authMethod}
* .authMethodNamespace(String) // optional {@link TokenQueryOptions#getAuthMethodNamespace() authMethodNamespace}
* .namespace(String) // optional {@link TokenQueryOptions#getNamespace() namespace}
* .build();
*
* @return A new ImmutableTokenQueryOptions builder
*/
public static ImmutableTokenQueryOptions.Builder builder() {
return new ImmutableTokenQueryOptions.Builder();
}
/**
* Builds instances of type {@link ImmutableTokenQueryOptions ImmutableTokenQueryOptions}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "TokenQueryOptions", generator = "Immutables")
public static final class Builder {
private ImmutableList.Builder toQueryParameters = ImmutableList.builder();
private ImmutableMap.Builder toHeaders = ImmutableMap.builder();
private @Nullable String policy;
private @Nullable String role;
private @Nullable String authMethod;
private @Nullable String authMethodNamespace;
private @Nullable String namespace;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.TokenQueryOptions} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(TokenQueryOptions instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.ParamAdder} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ParamAdder instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
if (object instanceof TokenQueryOptions) {
TokenQueryOptions instance = (TokenQueryOptions) object;
Optional namespaceOptional = instance.getNamespace();
if (namespaceOptional.isPresent()) {
namespace(namespaceOptional);
}
Optional roleOptional = instance.getRole();
if (roleOptional.isPresent()) {
role(roleOptional);
}
Optional authMethodOptional = instance.getAuthMethod();
if (authMethodOptional.isPresent()) {
authMethod(authMethodOptional);
}
Optional authMethodNamespaceOptional = instance.getAuthMethodNamespace();
if (authMethodNamespaceOptional.isPresent()) {
authMethodNamespace(authMethodNamespaceOptional);
}
Optional policyOptional = instance.getPolicy();
if (policyOptional.isPresent()) {
policy(policyOptional);
}
}
if (object instanceof ParamAdder) {
ParamAdder instance = (ParamAdder) object;
addAllToQueryParameters(instance.toQueryParameters());
putAllToHeaders(instance.toHeaders());
}
}
/**
* Adds one element to {@link TokenQueryOptions#toQueryParameters() toQueryParameters} list.
* @param element A toQueryParameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String element) {
this.toQueryParameters.add(element);
return this;
}
/**
* Adds elements to {@link TokenQueryOptions#toQueryParameters() toQueryParameters} list.
* @param elements An array of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String... elements) {
this.toQueryParameters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link TokenQueryOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toQueryParameters(Iterable elements) {
this.toQueryParameters = ImmutableList.builder();
return addAllToQueryParameters(elements);
}
/**
* Adds elements to {@link TokenQueryOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllToQueryParameters(Iterable elements) {
this.toQueryParameters.addAll(elements);
return this;
}
/**
* Put one entry to the {@link TokenQueryOptions#toHeaders() toHeaders} map.
* @param key The key in the toHeaders map
* @param value The associated value in the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(String key, String value) {
this.toHeaders.put(key, value);
return this;
}
/**
* Put one entry to the {@link TokenQueryOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(Map.Entry entry) {
this.toHeaders.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link TokenQueryOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toHeaders(Map entries) {
this.toHeaders = ImmutableMap.builder();
return putAllToHeaders(entries);
}
/**
* Put all mappings from the specified map as entries to {@link TokenQueryOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllToHeaders(Map entries) {
this.toHeaders.putAll(entries);
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getPolicy() policy} to policy.
* @param policy The value for policy
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder policy(String policy) {
this.policy = Objects.requireNonNull(policy, "policy");
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getPolicy() policy} to policy.
* @param policy The value for policy
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder policy(Optional policy) {
this.policy = policy.orElse(null);
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getRole() role} to role.
* @param role The value for role
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder role(String role) {
this.role = Objects.requireNonNull(role, "role");
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getRole() role} to role.
* @param role The value for role
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder role(Optional role) {
this.role = role.orElse(null);
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getAuthMethod() authMethod} to authMethod.
* @param authMethod The value for authMethod
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder authMethod(String authMethod) {
this.authMethod = Objects.requireNonNull(authMethod, "authMethod");
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getAuthMethod() authMethod} to authMethod.
* @param authMethod The value for authMethod
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder authMethod(Optional authMethod) {
this.authMethod = authMethod.orElse(null);
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getAuthMethodNamespace() authMethodNamespace} to authMethodNamespace.
* @param authMethodNamespace The value for authMethodNamespace
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder authMethodNamespace(String authMethodNamespace) {
this.authMethodNamespace = Objects.requireNonNull(authMethodNamespace, "authMethodNamespace");
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getAuthMethodNamespace() authMethodNamespace} to authMethodNamespace.
* @param authMethodNamespace The value for authMethodNamespace
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder authMethodNamespace(Optional authMethodNamespace) {
this.authMethodNamespace = authMethodNamespace.orElse(null);
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getNamespace() namespace} to namespace.
* @param namespace The value for namespace
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder namespace(String namespace) {
this.namespace = Objects.requireNonNull(namespace, "namespace");
return this;
}
/**
* Initializes the optional value {@link TokenQueryOptions#getNamespace() namespace} to namespace.
* @param namespace The value for namespace
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder namespace(Optional namespace) {
this.namespace = namespace.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableTokenQueryOptions ImmutableTokenQueryOptions}.
* @return An immutable instance of TokenQueryOptions
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableTokenQueryOptions build() {
return new ImmutableTokenQueryOptions(
toQueryParameters.build(),
toHeaders.build(),
policy,
role,
authMethod,
authMethodNamespace,
namespace);
}
}
}