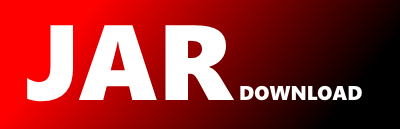
org.kiwiproject.consul.option.ImmutableTransactionOptions Maven / Gradle / Ivy
package org.kiwiproject.consul.option;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import jakarta.annotation.Nullable;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link TransactionOptions}.
*
* Use the builder to create immutable instances:
* {@code ImmutableTransactionOptions.builder()}.
*/
@Generated(from = "TransactionOptions", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableTransactionOptions extends TransactionOptions {
private final ImmutableList toQueryParameters;
private final @Nullable String datacenter;
private final ConsistencyMode consistencyMode;
private ImmutableTransactionOptions(ImmutableTransactionOptions.Builder builder) {
this.toQueryParameters = builder.toQueryParameters.build();
this.datacenter = builder.datacenter;
this.consistencyMode = builder.consistencyMode != null
? builder.consistencyMode
: Objects.requireNonNull(super.getConsistencyMode(), "consistencyMode");
}
private ImmutableTransactionOptions(
ImmutableList toQueryParameters,
@Nullable String datacenter,
ConsistencyMode consistencyMode) {
this.toQueryParameters = toQueryParameters;
this.datacenter = datacenter;
this.consistencyMode = consistencyMode;
}
/**
* @return The value of the {@code toQueryParameters} attribute
*/
@Override
public ImmutableList toQueryParameters() {
return toQueryParameters;
}
/**
* @return The value of the {@code datacenter} attribute
*/
@Override
public Optional getDatacenter() {
return Optional.ofNullable(datacenter);
}
/**
* @return The value of the {@code consistencyMode} attribute
*/
@Override
public ConsistencyMode getConsistencyMode() {
return consistencyMode;
}
/**
* Copy the current immutable object with elements that replace the content of {@link TransactionOptions#toQueryParameters() toQueryParameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableTransactionOptions withToQueryParameters(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableTransactionOptions(newValue, this.datacenter, this.consistencyMode);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TransactionOptions#toQueryParameters() toQueryParameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of toQueryParameters elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableTransactionOptions withToQueryParameters(Iterable elements) {
if (this.toQueryParameters == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableTransactionOptions(newValue, this.datacenter, this.consistencyMode);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TransactionOptions#getDatacenter() datacenter} attribute.
* @param value The value for datacenter
* @return A modified copy of {@code this} object
*/
public final ImmutableTransactionOptions withDatacenter(String value) {
String newValue = Objects.requireNonNull(value, "datacenter");
if (Objects.equals(this.datacenter, newValue)) return this;
return new ImmutableTransactionOptions(this.toQueryParameters, newValue, this.consistencyMode);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TransactionOptions#getDatacenter() datacenter} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for datacenter
* @return A modified copy of {@code this} object
*/
public final ImmutableTransactionOptions withDatacenter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.datacenter, value)) return this;
return new ImmutableTransactionOptions(this.toQueryParameters, value, this.consistencyMode);
}
/**
* Copy the current immutable object by setting a value for the {@link TransactionOptions#getConsistencyMode() consistencyMode} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for consistencyMode
* @return A modified copy of the {@code this} object
*/
public final ImmutableTransactionOptions withConsistencyMode(ConsistencyMode value) {
if (this.consistencyMode == value) return this;
ConsistencyMode newValue = Objects.requireNonNull(value, "consistencyMode");
return new ImmutableTransactionOptions(this.toQueryParameters, this.datacenter, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableTransactionOptions} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableTransactionOptions
&& equalTo(0, (ImmutableTransactionOptions) another);
}
private boolean equalTo(int synthetic, ImmutableTransactionOptions another) {
return toQueryParameters.equals(another.toQueryParameters)
&& Objects.equals(datacenter, another.datacenter)
&& consistencyMode.equals(another.consistencyMode);
}
/**
* Computes a hash code from attributes: {@code toQueryParameters}, {@code datacenter}, {@code consistencyMode}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + toQueryParameters.hashCode();
h += (h << 5) + Objects.hashCode(datacenter);
h += (h << 5) + consistencyMode.hashCode();
return h;
}
/**
* Prints the immutable value {@code TransactionOptions} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("TransactionOptions")
.omitNullValues()
.add("toQueryParameters", toQueryParameters)
.add("datacenter", datacenter)
.add("consistencyMode", consistencyMode)
.toString();
}
/**
* Creates an immutable copy of a {@link TransactionOptions} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TransactionOptions instance
*/
public static ImmutableTransactionOptions copyOf(TransactionOptions instance) {
if (instance instanceof ImmutableTransactionOptions) {
return (ImmutableTransactionOptions) instance;
}
return ImmutableTransactionOptions.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableTransactionOptions ImmutableTransactionOptions}.
*
* ImmutableTransactionOptions.builder()
* .addToQueryParameters|addAllToQueryParameters(String) // {@link TransactionOptions#toQueryParameters() toQueryParameters} elements
* .datacenter(String) // optional {@link TransactionOptions#getDatacenter() datacenter}
* .consistencyMode(org.kiwiproject.consul.option.ConsistencyMode) // optional {@link TransactionOptions#getConsistencyMode() consistencyMode}
* .build();
*
* @return A new ImmutableTransactionOptions builder
*/
public static ImmutableTransactionOptions.Builder builder() {
return new ImmutableTransactionOptions.Builder();
}
/**
* Builds instances of type {@link ImmutableTransactionOptions ImmutableTransactionOptions}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "TransactionOptions", generator = "Immutables")
public static final class Builder {
private ImmutableList.Builder toQueryParameters = ImmutableList.builder();
private @Nullable String datacenter;
private @Nullable ConsistencyMode consistencyMode;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.ParamAdder} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ParamAdder instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.kiwiproject.consul.option.TransactionOptions} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(TransactionOptions instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
if (object instanceof ParamAdder) {
ParamAdder instance = (ParamAdder) object;
addAllToQueryParameters(instance.toQueryParameters());
}
if (object instanceof TransactionOptions) {
TransactionOptions instance = (TransactionOptions) object;
Optional datacenterOptional = instance.getDatacenter();
if (datacenterOptional.isPresent()) {
datacenter(datacenterOptional);
}
consistencyMode(instance.getConsistencyMode());
}
}
/**
* Adds one element to {@link TransactionOptions#toQueryParameters() toQueryParameters} list.
* @param element A toQueryParameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String element) {
this.toQueryParameters.add(element);
return this;
}
/**
* Adds elements to {@link TransactionOptions#toQueryParameters() toQueryParameters} list.
* @param elements An array of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addToQueryParameters(String... elements) {
this.toQueryParameters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link TransactionOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toQueryParameters(Iterable elements) {
this.toQueryParameters = ImmutableList.builder();
return addAllToQueryParameters(elements);
}
/**
* Adds elements to {@link TransactionOptions#toQueryParameters() toQueryParameters} list.
* @param elements An iterable of toQueryParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllToQueryParameters(Iterable elements) {
this.toQueryParameters.addAll(elements);
return this;
}
/**
* Initializes the optional value {@link TransactionOptions#getDatacenter() datacenter} to datacenter.
* @param datacenter The value for datacenter
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder datacenter(String datacenter) {
this.datacenter = Objects.requireNonNull(datacenter, "datacenter");
return this;
}
/**
* Initializes the optional value {@link TransactionOptions#getDatacenter() datacenter} to datacenter.
* @param datacenter The value for datacenter
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder datacenter(Optional datacenter) {
this.datacenter = datacenter.orElse(null);
return this;
}
/**
* Initializes the value for the {@link TransactionOptions#getConsistencyMode() consistencyMode} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link TransactionOptions#getConsistencyMode() consistencyMode}.
* @param consistencyMode The value for consistencyMode
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder consistencyMode(ConsistencyMode consistencyMode) {
this.consistencyMode = Objects.requireNonNull(consistencyMode, "consistencyMode");
return this;
}
/**
* Builds a new {@link ImmutableTransactionOptions ImmutableTransactionOptions}.
* @return An immutable instance of TransactionOptions
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableTransactionOptions build() {
return new ImmutableTransactionOptions(this);
}
}
}