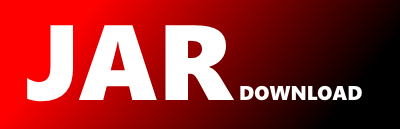
org.kiwiproject.test.assertj.KiwiAssertJ Maven / Gradle / Ivy
Show all versions of kiwi-test Show documentation
package org.kiwiproject.test.assertj;
import static org.assertj.core.api.Assertions.assertThat;
import lombok.experimental.UtilityClass;
/**
* Some AssertJ test utilities that you might or might find useful.
*/
@UtilityClass
public class KiwiAssertJ {
/**
* Assert the given object has exactly the given type.
*
* Returns the object cast to an instance of that type if the assertion passes, otherwise fails the test.
*
* @param object the object to assert
* @param type the expected type
* @param the expected type parameter
* @return the object cast to an instance of T
*/
public static T assertIsExactType(Object object, Class type) {
assertThat(object).isExactlyInstanceOf(type);
return type.cast(object);
}
/**
* Assert the given object is an instance of the given type, i.e. is the same type or a subtype of the given type.ª
*
* Returns the object cast to an instance of that type if the assertion passes, otherwise fails the test.
*
* @param object the object to assert
* @param type the expected type
* @param the expected type parameter
* @return the object cast to an instance of T
*/
public static T assertIsTypeOrSubtype(Object object, Class type) {
assertThat(object).isInstanceOf(type);
return type.cast(object);
}
}