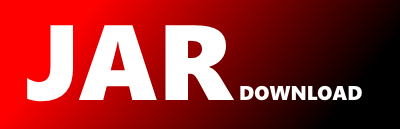
org.kiwiproject.base.KiwiPrimitives Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kiwi Show documentation
Show all versions of kiwi Show documentation
Kiwi is a utility library. We really like Google's Guava, and also use Apache Commons.
But if they don't have something we need, and we think it is useful, this is where we put it.
package org.kiwiproject.base;
import static com.google.common.base.Preconditions.checkArgument;
import lombok.experimental.UtilityClass;
/**
* Static utilities that operate on primitive values, and are not already provided by classes in Guava's
* {@link com.google.common.primitives} package.
*/
@UtilityClass
public class KiwiPrimitives {
/**
* Returns the first non-zero argument, otherwise throws {@link IllegalArgumentException} if both arguments
* are zero.
*
* @param first the first int to check
* @param second the second int to check
* @return the first non-zero value
* @throws IllegalArgumentException if both arguments are zero
*/
public static int firstNonZero(int first, int second) {
return first != 0 ? first : (int) checkNonZero(second);
}
/**
* Returns the first non-zero argument, otherwise throws {@link IllegalArgumentException} if both arguments
* are zero.
*
* @param first the first int to check
* @param second the second int to check
* @return the first non-zero value
* @throws IllegalArgumentException if both arguments are zero
*/
public static long firstNonZero(long first, long second) {
return first != 0 ? first : checkNonZero(second);
}
private static long checkNonZero(long value) {
checkArgument(value != 0, "One of the arguments must be non-zero");
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy