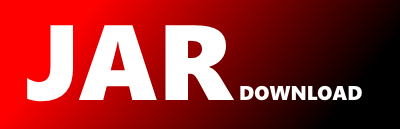
org.kiwiproject.collect.KiwiMaps Maven / Gradle / Ivy
Show all versions of kiwi Show documentation
package org.kiwiproject.collect;
import static java.util.Objects.isNull;
import static org.kiwiproject.base.KiwiPreconditions.checkEvenItemCount;
import lombok.experimental.UtilityClass;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* Utility methods for working with {@link Map} instances
*/
@UtilityClass
public class KiwiMaps {
/**
* Checks whether the specified map is null or empty.
*
* @param map the map
* @param the type of the keys in the map
* @param the type of the values in the map
* @return {@code true} if map is null or empty; {@code false} otherwise
*/
public static boolean isNullOrEmpty(Map map) {
return map == null || map.isEmpty();
}
/**
* Checks whether the specified map is neither null nor empty.
*
* @param map the map
* @param the type of the keys in the map
* @param the type of the values in the map
* @return {@code true} if map is neither null nor empty; {@code false} otherwise
*/
public static boolean isNotNullOrEmpty(Map map) {
return !isNullOrEmpty(map);
}
/**
* Creates a mutable, {@link java.util.HashMap} instance containing key/value pairs as parsed in pairs from
* the items argument. The items argument contains keys and values in the form:
*
* key-1, value-1, key-2, value-2, ... , key-N, value-N
*
* @param items the items containing keys and values, in pairs
* @param the type of the keys in the map
* @param the type of the values in the map
* @return a new HashMap with data from items
*/
public static Map newHashMap(Object... items) {
checkEvenItemCount(items);
var map = new HashMap(items.length);
populate(map, items);
return map;
}
/**
* Creates a mutable, {@link java.util.LinkedHashMap} instance containing key/value pairs as parsed in pairs from
* the items argument. The items argument contains keys and values in the form:
*
* key-1, value-1, key-2, value-2, ... , key-N, value-N
*
* @param items the items containing keys and values, in pairs
* @param the type of the keys in the map
* @param the type of the values in the map
* @return a new LinkedHashMap with data from items
*/
public static Map newLinkedHashMap(Object... items) {
checkEvenItemCount(items);
var map = new LinkedHashMap(items.length);
populate(map, items);
return map;
}
/**
* Creates a mutable, {@link java.util.TreeMap} instance containing key/value pairs as parsed in pairs from
* the items argument. The items argument contains keys and values in the form:
*
* key-1, value-1, key-2, value-2, ... , key-N, value-N
*
* @param items the items containing keys and values, in pairs
* @param the type of the keys in the map
* @param the type of the values in the map
* @return a new TreeMap with data from items
*/
public static , V> SortedMap newTreeMap(Object... items) {
checkEvenItemCount(items);
var map = new TreeMap();
populate(map, items);
return map;
}
/**
* Creates a mutable, {@link ConcurrentHashMap} instance containing key/value pairs as parsed in pairs from
* the items argument. The items argument contains keys and values in the form:
*
* key-1, value-1, key-2, value-2, ... , key-N, value-N
*
* @param items the items containing keys and values, in pairs
* @param the type of the keys in the map
* @param the type of the values in the map
* @return a new ConcurrentHashMap with data from items
*/
public static ConcurrentMap newConcurrentHashMap(Object... items) {
checkEvenItemCount(items);
var map = new ConcurrentHashMap(items.length);
populate(map, items);
return map;
}
@SuppressWarnings("unchecked")
private static void populate(Map map, Object... items) {
for (var i = 0; i < items.length; i += 2) {
var key = (K) items[i];
var value = (V) items[i + 1];
map.put(key, value);
}
}
/**
* Returns {@code true} if and only if (1) {@code map} is not null or empty, (2) {@code map} contains the given
* {@code key}, and (3) the value associated with the given key is {@code null}.
*
* @param map the map
* @param key the key check
* @param the type of the keys in the map
* @param the type of the values in the map
* @return {@code true} if and only if (1) {@code map} is not null or empty, (2) {@code map} contains the given
* {@code key}, and (3) the value associated with the given key is {@code null}
*/
public static boolean keyExistsWithNullValue(Map map, K key) {
return isNotNullOrEmpty(map) && map.containsKey(key) && isNull(map.get(key));
}
}