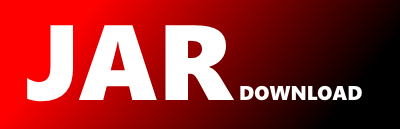
org.kiwiproject.json.KiwiJacksonSerializers Maven / Gradle / Ivy
Show all versions of kiwi Show documentation
package org.kiwiproject.json;
import static java.util.stream.Collectors.toList;
import com.fasterxml.jackson.databind.BeanDescription;
import com.fasterxml.jackson.databind.SerializationConfig;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.ser.BeanPropertyWriter;
import com.fasterxml.jackson.databind.ser.BeanSerializerModifier;
import lombok.experimental.UtilityClass;
import java.util.List;
/**
* Custom Jackson serializers.
*
* Jackson databind must be available at runtime.
*/
@UtilityClass
public class KiwiJacksonSerializers {
/**
* Build a new {@link SimpleModule} that will replace the values of specific fields with a "masked" value
* and will replace any exceptions with a message indicating the field could not be serialized.
*
* Uses the default replacement text provided by {@link PropertyMaskingOptions}.
*
* @param maskedFieldRegexps list containing regular expressions that define the properties to mask
* @return a new {@link SimpleModule}
*/
public static SimpleModule buildPropertyMaskingSafeSerializerModule(List maskedFieldRegexps) {
var options = PropertyMaskingOptions.builder()
.maskedFieldRegexps(maskedFieldRegexps)
.build();
return buildPropertyMaskingSafeSerializerModule(options);
}
/**
* Build a new {@link SimpleModule} that will replace the values of specific fields with a "masked" value
* and will replace any exceptions with a message indicating the field could not be serialized.
*
* @param options the specific masking and serialization error options to use
* @return a new {@link SimpleModule}
* @implNote Per the docs for {@link BeanSerializerModifier#changeProperties(SerializationConfig, BeanDescription, List)}
* the returned list is mutable.
*/
public static SimpleModule buildPropertyMaskingSafeSerializerModule(PropertyMaskingOptions options) {
var modifier = new BeanSerializerModifier() {
@Override
public List changeProperties(SerializationConfig config,
BeanDescription beanDesc,
List beanProperties) {
return beanProperties.stream()
.map(beanPropertyWriter ->
new PropertyMaskingSafePropertyWriter(beanPropertyWriter, options))
.collect(toList());
}
};
return new SimpleModule().setSerializerModifier(modifier);
}
}