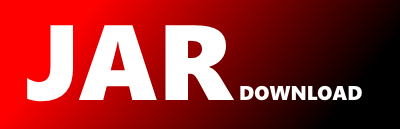
org.kiwiproject.retry.SimpleRetryer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kiwi Show documentation
Show all versions of kiwi Show documentation
Kiwi is a utility library. We really like Google's Guava, and also use Apache Commons.
But if they don't have something we need, and we think it is useful, this is where we put it.
package org.kiwiproject.retry;
import com.google.common.annotations.VisibleForTesting;
import lombok.Builder;
import org.kiwiproject.base.DefaultEnvironment;
import org.kiwiproject.base.KiwiEnvironment;
import org.slf4j.event.Level;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
import java.util.function.Supplier;
/**
* A simple class to retry an operation up to a maximum number of attempts. Uses the same set of initial values for
* maximum number of attempts, delay between attempts, etc. Consider using this rather than {@link SimpleRetries}
* directly.
*
* You can construct a {@link SimpleRetryer} using the builder obtained via {@code SimpleRetryer.builder()}.
*
* Available configuration options for SimpleRetryer:
*
* Name
* Default
* Description
*
*
* environment
* a {@link DefaultEnvironment} instance
* mainly useful for testing, e.g. to supply a mock
*
*
* maxAttempts
* {@link #DEFAULT_MAX_ATTEMPTS}
* the maximum number of attempts to make before giving up
*
*
* retryDelayTime
* {@link #DEFAULT_RETRY_DELAY_TIME}
* the time value to wait between attempts
*
*
* retryDelayUnit
* {@link #DEFAULT_RETRY_DELAY_UNIT}
* the time unit for {@code retryDelayTime}
*
*
* commonType
* {@link #DEFAULT_TYPE}
* use this to specify a common type/description that retryer will get (used in log messages)
*
*
* logLevelForSubsequentAttempts
* {@link Level#TRACE}
* the log level at which retries will be logged (the first attempt is always logged at TRACE)
*
*
*
* @implNote This is basically an instance wrapper around {@link SimpleRetries} to allow for specification of
* common configuration, and thus avoid calling methods with many parameters. It also facilitates easy mocking in
* tests as opposed to the static methods in {@link SimpleRetries}.
*/
@Builder
public class SimpleRetryer {
/**
* Default maximum attempts.
*/
public static final int DEFAULT_MAX_ATTEMPTS = 3;
/**
* Default retry delay time. This is a static value, i.e. there is no fancy exponential or linear backoff.
*/
public static final long DEFAULT_RETRY_DELAY_TIME = 50;
/**
* Default retry delay time unit.
*/
public static final TimeUnit DEFAULT_RETRY_DELAY_UNIT = TimeUnit.MILLISECONDS;
/**
* Default value to include in attempt log messages.
*/
public static final String DEFAULT_TYPE = "object";
/**
* THe {@link KiwiEnvironment} to use when sleeping between retry attempts.
*/
@VisibleForTesting
@Builder.Default
KiwiEnvironment environment = new DefaultEnvironment();
/**
* The maximum number of attempts before giving up.
*/
@VisibleForTesting
@Builder.Default
int maxAttempts = DEFAULT_MAX_ATTEMPTS;
/**
* The time to sleep between retry attempts.
*/
@VisibleForTesting
@Builder.Default
long retryDelayTime = DEFAULT_RETRY_DELAY_TIME;
/**
* The time unit for the time to sleep between retry attempts.
*/
@VisibleForTesting
@Builder.Default
TimeUnit retryDelayUnit = DEFAULT_RETRY_DELAY_UNIT;
/**
* The common type/description to include in log messages for each attempt.
*/
@VisibleForTesting
@Builder.Default
String commonType = DEFAULT_TYPE;
/**
* The SLF4J log level to use when logging retry attempts. The first attempt is always logged at TRACE level.
*/
@VisibleForTesting
@Builder.Default
Level logLevelForSubsequentAttempts = Level.TRACE;
/**
* Try to get an object.
*
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return an Optional which either contains a value, or is empty if all attempts failed
*/
public Optional tryGetObject(Supplier supplier) {
return tryGetObject(commonType, supplier);
}
/**
* Try to get an object.
*
* @param type the type of object to return, used only in logging messages
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return an Optional which either contains a value, or is empty if all attempts failed
*/
public Optional tryGetObject(Class type, Supplier supplier) {
return tryGetObject(type.getSimpleName(), supplier);
}
/**
* Try to get an object.
*
* @param type the type of object to return, used only in logging messages
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return an Optional which either contains a value, or is empty if all attempts failed
*/
public Optional tryGetObject(String type, Supplier supplier) {
return SimpleRetries.tryGetObject(
maxAttempts,
retryDelayTime, retryDelayUnit,
environment,
type,
logLevelForSubsequentAttempts,
supplier);
}
/**
* Try to get an object.
*
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return a {@link RetryResult}
*/
public RetryResult tryGetObjectCollectingErrors(Supplier supplier) {
return tryGetObjectCollectingErrors(commonType, supplier);
}
/**
* Try to get an object.
*
* @param type the type of object to return, used only in logging messages
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return a {@link RetryResult}
*/
public RetryResult tryGetObjectCollectingErrors(Class type, Supplier supplier) {
return tryGetObjectCollectingErrors(type.getSimpleName(), supplier);
}
/**
* Try to get an object.
*
* @param type the type of object to return, used only in logging messages
* @param supplier on success return the object; return {@code null} or throw exception if attempt failed
* @param the type of object
* @return a {@link RetryResult}
*/
public RetryResult tryGetObjectCollectingErrors(String type, Supplier supplier) {
return SimpleRetries.tryGetObjectCollectingErrors(
maxAttempts,
retryDelayTime, retryDelayUnit,
environment,
type,
supplier
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy