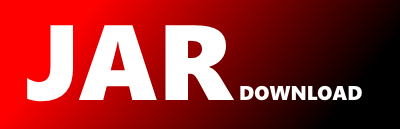
commonMain.org.kodein.di.DIBuilder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kodein-di-js Show documentation
Show all versions of kodein-di-js Show documentation
KODEIN Dependency Injection Core
package org.kodein.di
import org.kodein.di.bindings.*
import org.kodein.type.generic
//region Straight bindings
/**
* Binds a singleton: will create an instance on first request and will subsequently always return the same instance.
*
* T generics will be erased!
*
* @param T The created type.
* @param creator The function that will be called the first time an instance is requested. Guaranteed to be called only once. Should create a new instance.
*/
public inline fun DI.Builder.bindSingleton(tag: Any? = null, overrides: Boolean? = null, sync: Boolean = true, noinline creator: DirectDI.() -> T): Unit = Bind(tag = tag, overrides = overrides, binding = singleton(sync = sync, creator = creator))
/**
* Binds an eager singleton: will create an instance as soon as kodein is ready (all bindings are set) and will always return this instance.
*
* T generics will be erased!
*
* @param T The created type.
* @param creator The function that will be called as soon as DI is ready. Guaranteed to be called only once. Should create a new instance.
*/
public inline fun DI.Builder.bindEagerSingleton(tag: Any? = null, overrides: Boolean? = null, noinline creator: DirectDI.() -> T): Unit = Bind(tag = tag, overrides = overrides, binding = eagerSingleton(creator = creator))
/**
* Binds a multiton: will create an instance on first request and will subsequently always return the same instance.
*
* T generics will be erased!
*
* @param T The created type.
* @param creator The function that will be called the first time an instance is requested. Guaranteed to be called only once. Should create a new instance.
*/
public inline fun DI.Builder.bindMultiton(tag: Any? = null, overrides: Boolean? = null, sync: Boolean = true, noinline creator: DirectDI.(A) -> T): Unit = Bind(tag = tag, overrides = overrides, binding = multiton(sync = sync, creator = creator))
/**
* Creates a factory: each time an instance is needed, the function [creator] function will be called.
*
* T generics will be erased!
*
* A provider is like a [factory], but without argument.
*
* @param T The created type.
* @param creator The function that will be called each time an instance is requested. Should create a new instance.
*/
public inline fun DI.Builder.bindProvider(tag: Any? = null, overrides: Boolean? = null, noinline creator: DirectDI.() -> T): Unit = Bind(tag = tag, overrides = overrides, binding = provider(creator = creator))
/**
* Binds a factory: each time an instance is needed, the function [creator] function will be called.
*
* A & T generics will be erased!
*
* @param A The argument type.
* @param T The created type.
* @param creator The function that will be called each time an instance is requested. Should create a new instance.
*/
public inline fun DI.Builder.bindFactory(tag: Any? = null, overrides: Boolean? = null, noinline creator: DirectDI.(A) -> T): Unit = Bind(tag = tag, overrides = overrides, binding = factory(creator = creator))
/**
* Binds an instance provider: will always return the given instance.
*
* T generics will be erased!
*
* @param T The type of the instance.
* @param creator A function that must the instance of type T.
*/
public inline fun DI.Builder.bindInstance(tag: Any? = null, overrides: Boolean? = null, creator: () -> T): Unit = Bind(tag = tag, overrides = overrides, binding = instance(creator()))
/**
* Binds a constant provider: will always return the given instance.
*
* T generics will be erased!
*
* @param T The type of the constant.
* @param creator A function that must the constant of type T.
*/
public inline fun DI.Builder.bindConstant(tag: Any, overrides: Boolean? = null, creator: () -> T): Unit = Bind(tag = tag, overrides = overrides, binding = instance(creator()))
/**
* Attaches a binding to the DI container
*
* @param T The type of value to bind.
* @param tag The tag to bind.
* @param overrides Whether this bind **must** or **must not** override an existing binding.
*/
public inline fun DI.Builder.bind(tag: Any? = null, overrides: Boolean? = null, noinline createBinding: () -> DIBinding<*, *, T>): Unit = Bind(tag, overrides, createBinding())
//endregion
//region Advanced bindings
/**
* Starts the binding of a given type with a given tag.
*
* T generics will be erased!
*
* @param T The type to bind.
* @param tag The tag to bind.
* @param overrides Whether this bind **must**, **may** or **must not** override an existing binding.
* @return The binder: call `DI.Builder.TypeBinder.with` on it to finish the binding syntax and register the binding.
*/
public inline fun DI.Builder.bind(tag: Any? = null, overrides: Boolean? = null): DI.Builder.TypeBinder = Bind(generic(), tag, overrides)
/**
* Starts a direct binding with a given tag. A direct bind does not define the type to be bound, the type will be defined according to the bound factory.
*
* @param tag The tag to bind.
* @param overrides Whether this bind **must**, **may** or **must not** override an existing binding.
* @return The binder: call [DI.Builder.DirectBinder.from]) on it to finish the binding syntax and register the binding.
*/
@Deprecated(
message = "'bind() from [BINDING]' might be replaced by 'bind { [BINDING] }' (This will be removed in Kodein-DI 8.0)",
level = DeprecationLevel.ERROR
)
public fun DI.Builder.bind(tag: Any? = null, overrides: Boolean? = null): DI.Builder.DirectBinder = Bind(tag, overrides)
/**
* Binds the previously given tag to the given instance.
*
* T generics will be erased!
*
* @param T The type of value to bind.
* @param value The instance to bind.
*/
public inline infix fun DI.Builder.ConstantBinder.with(value: T): Unit = With(generic(), value)
//endregion
//region SearchDSL
/**
* Creates a return type constrained spec.
*
* @property tag An optional tag constraint.
*/
@Suppress("unused")
public inline fun SearchDSL.binding(tag: Any? = null): SearchDSL.Binding = SearchDSL.Binding(generic(), tag)
/**
* Creates a context constrained spec.
*/
public inline fun SearchDSL.context(): SearchDSL.Spec = Context(generic())
/**
* Creates an argument constrained spec.
*/
public inline fun SearchDSL.argument(): SearchDSL.Spec = Argument(generic())
//endregion
//region Context / Scopes
/**
* Used to define bindings with a scope: `bind() with scoped(myScope).singleton { /*...*/ }`
*
* @param EC The scope's environment context type.
* @param BC The scope's Binding context type.
*/
public inline fun DI.Builder.scoped(scope: Scope): DI.BindBuilder.WithScope = DI.BindBuilder.ImplWithScope(generic(), scope)
/**
* Used to define bindings with a context: `bind() with contexted().provider { /*...*/ }`
*
* @param C The context type.
*/
public inline fun DI.Builder.contexted(): DI.BindBuilder = DI.BindBuilder.ImplWithContext(generic())
/**
* Creates a factory: each time an instance is needed, the function [creator] function will be called.
*
* A & T generics will be erased!
*
* @param A The argument type.
* @param T The created type.
* @param creator The function that will be called each time an instance is requested. Should create a new instance.
* @return A factory ready to be bound.
*/
public inline fun DI.BindBuilder.factory(noinline creator: BindingDI.(A) -> T): Factory = Factory(contextType, generic(), generic(), creator)
/**
* Creates a factory: each time an instance is needed, the function [creator] function will be called.
*
* T generics will be erased!
*
* A provider is like a [factory], but without argument.
*
* @param T The created type.
* @param creator The function that will be called each time an instance is requested. Should create a new instance.
* @return A provider ready to be bound.
*/
public inline fun DI.BindBuilder.provider(noinline creator: NoArgBindingDI.() -> T): Provider = Provider(contextType, generic(), creator)
/**
* Creates a singleton: will create an instance on first request and will subsequently always return the same instance.
*
* T generics will be erased!
*
* @param T The created type.
* @param creator The function that will be called the first time an instance is requested. Guaranteed to be called only once. Should create a new instance.
* @return A singleton ready to be bound.
*/
public inline fun DI.BindBuilder.WithScope.singleton(ref: RefMaker? = null, sync: Boolean = true, noinline creator: NoArgBindingDI.() -> T): Singleton = Singleton(scope, contextType, explicitContext, generic(), ref, sync, creator)
/**
* Creates a multiton: will create an instance on first request for each different argument and will subsequently always return the same instance for the same argument.
*
* A & T generics will be erased!
*
* @param A The argument type.
* @param T The created type.
* @param creator The function that will be called the first time an instance is requested with a new argument. Guaranteed to be called only once per argument. Should create a new instance.
* @return A multiton ready to be bound.
*/
public inline fun DI.BindBuilder.WithScope.multiton(ref: RefMaker? = null, sync: Boolean = true, noinline creator: BindingDI.(A) -> T): Multiton = Multiton(scope, contextType, explicitContext, generic(), generic(), ref, sync, creator)
/**
* Creates an eager singleton: will create an instance as soon as kodein is ready (all bindings are set) and will always return this instance.
*
* T generics will be erased!
*
* @param T The created type.
* @param creator The function that will be called as soon as DI is ready. Guaranteed to be called only once. Should create a new instance.
* @return An eager singleton ready to be bound.
*/
public inline fun DI.Builder.eagerSingleton(noinline creator: NoArgBindingDI.() -> T): EagerSingleton = EagerSingleton(containerBuilder, generic(), creator)
/**
* Creates an instance provider: will always return the given instance.
*
* T generics will be erased!
*
* @param T The type of the instance.
* @param instance The object that will always be returned.
* @return An instance provider ready to be bound.
*/
public inline fun DI.Builder.instance(instance: T): InstanceBinding = InstanceBinding(generic(), instance)
//endregion
//region ContextTranslator
public inline fun contextTranslator(noinline t: DirectDI.(C) -> S?): ContextTranslator = SimpleContextTranslator(generic(), generic(), t)
public inline fun DI.Builder.registerContextTranslator(noinline t: DirectDI.(C) -> S?): Unit = RegisterContextTranslator(contextTranslator(t))
public inline fun contextFinder(noinline t: DirectDI.() -> S) : ContextTranslator = SimpleAutoContextTranslator(generic(), t)
public inline fun DI.Builder.registerContextFinder(noinline t: DirectDI.() -> S): Unit = RegisterContextTranslator(contextFinder(t))
//endregion
© 2015 - 2024 Weber Informatics LLC | Privacy Policy