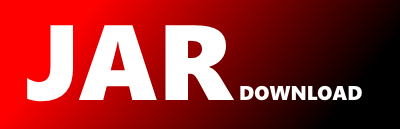
commonMain.org.kodein.di.Named.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kodein-di-js Show documentation
Show all versions of kodein-di-js Show documentation
KODEIN Dependency Injection Core
@file:Suppress("DEPRECATION")
package org.kodein.di
import org.kodein.type.TypeToken
import kotlin.jvm.JvmInline
@JvmInline
public value class Named(public val di: DIAware) {
/**
* Gets a factory of [T] for the given argument type and return type.
* The name of the receiving property is used as tag.
*
* @param A The type of argument the returned factory takes.
* @param T The type of object to retrieve with the returned factory.
* @param argType The type of argument the returned factory takes.
* @param type The type of object to retrieve with the returned factory.
* @return A factory of [T].
* @throws DI.NotFoundException If no factory was found.
* @throws DI.DependencyLoopException When calling the factory, if the value construction triggered a dependency loop.
*/
public fun Factory(argType: TypeToken, type: TypeToken): DIProperty<(A) -> T> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factory(DI.Key(ctx.anyType, argType, type, tag), ctx.value) }
/**
* Gets a factory of [T] for the given argument type and return type, or null if none is found.
* The name of the receiving property is used as tag.
*
* @param A The type of argument the returned factory takes.
* @param T The type of object to retrieve with the returned factory.
* @param argType The type of argument the returned factory takes.
* @param type The type of object to retrieve with the returned factory.
* @return A factory of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the factory, if the value construction triggered a dependency loop.
*/
public fun FactoryOrNull(argType: TypeToken, type: TypeToken): DIProperty<((A) -> T)?> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factoryOrNull(DI.Key(ctx.anyType, argType, type, tag), ctx.value) }
/**
* Gets a provider of [T] for the given type.
* The name of the receiving property is used as tag.
*
* @param T The type of object to retrieve with the returned provider.
* @param type The type of object to retrieve with the returned provider.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public fun Provider(type: TypeToken): DIProperty<() -> T> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.provider(DI.Key(ctx.anyType, TypeToken.Unit, type, tag), ctx.value) }
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param argType The type of argument the curried factory takes.
* @param type The type of object to retrieve with the returned provider.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public fun Provider(argType: TypeToken, type: TypeToken, arg: () -> A): DIProperty<() -> T> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factory(DI.Key(ctx.anyType, argType, type, tag), ctx.value).toProvider(arg) }
/**
* Gets a provider of [T] for the given type, or null if none is found.
* The name of the receiving property is used as tag.
*
* @param T The type of object to retrieve with the returned provider.
* @param type The type of object to retrieve with the returned provider.
* @return A provider of [T], or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public fun ProviderOrNull(type: TypeToken): DIProperty<(() -> T)?> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.providerOrNull(DI.Key(ctx.anyType, TypeToken.Unit, type, tag), ctx.value) }
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param argType The type of argument the curried factory takes.
* @param type The type of object to retrieve with the returned provider.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public fun ProviderOrNull(argType: TypeToken, type: TypeToken, arg: () -> A): DIProperty<(() -> T)?> =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factoryOrNull(DI.Key(ctx.anyType, argType, type, tag), ctx.value)?.toProvider(arg) }
/**
* Gets an instance of [T] for the given type.
* The name of the receiving property is used as tag.
*
* @param T The type of object to retrieve.
* @param type The type of object to retrieve.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public fun Instance(type: TypeToken): DIProperty =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.provider(DI.Key(ctx.anyType, TypeToken.Unit, type, tag), ctx.value).invoke() }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param argType The type of argument the curried factory takes.
* @param type The type of object to retrieve.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public fun Instance(argType: TypeToken, type: TypeToken, arg: () -> A): DIProperty =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factory(DI.Key(ctx.anyType, argType, type, tag), ctx.value).invoke(arg()) }
/**
* Gets an instance of [T] for the given type, or null if none is found.
* The name of the receiving property is used as tag.
*
* @param type The type of object to retrieve.
* @return An instance of [T], or null if no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public fun InstanceOrNull(type: TypeToken): DIProperty =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.providerOrNull(DI.Key(ctx.anyType, TypeToken.Unit, type, tag), ctx.value)?.invoke() }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param type The type of object to retrieve.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public fun InstanceOrNull(argType: TypeToken, type: TypeToken, arg: () -> A): DIProperty =
DIProperty(di.diTrigger, di.diContext) { ctx, tag -> di.di.container.factoryOrNull(DI.Key(ctx.anyType, argType, type, tag), ctx.value)?.invoke(arg()) }
}
/**
* Allows to get factories / providers / instances with a tag set to the name of the receiving property.
*/
public val DIAware.named: Named get() = Named(this)
/**
* Gets a constant of type [T] and tag whose tag is the name of the receiving property.
*
* @param T The type of object to retrieve.
* @param type The type of object to retrieve.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public fun DIAware.Constant(type: TypeToken): DIProperty = named.Instance(type)
© 2015 - 2024 Weber Informatics LLC | Privacy Policy