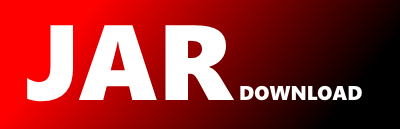
commonMain.org.kodein.di.Retrieving.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kodein-di-js Show documentation
Show all versions of kodein-di-js Show documentation
KODEIN Dependency Injection Core
package org.kodein.di
import org.kodein.type.generic
//region Standard retrieving
/**
* Gets a factory of `T` for the given argument type, return type and tag.
*
* A & T generics will be preserved!
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @param tag The bound tag, if any.
* @return A factory.
* @throws DI.NotFoundException if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun DIAware.factory(tag: Any? = null): LazyDelegate<(A) -> T> =
Factory(generic(), generic(), tag)
/**
* Gets a factory of `T` for the given argument type, return type and tag, or nul if none is found.
*
* A & T generics will be preserved!
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @param tag The bound tag, if any.
* @return A factory, or null if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun DIAware.factoryOrNull(tag: Any? = null): LazyDelegate<((A) -> T)?> =
FactoryOrNull(generic(), generic(), tag)
/**
* Gets a provider of `T` for the given type and tag.
*
* T generics will be preserved!
*
* @param T The type of object the provider returns.
* @param tag The bound tag, if any.
* @return A provider.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
public inline fun DIAware.provider(tag: Any? = null): LazyDelegate<() -> T> =
Provider(generic(), tag)
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.provider(
tag: Any? = null,
arg: A,
): LazyDelegate<() -> T> = Provider(generic(), generic(), tag) { arg }
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.provider(
tag: Any? = null,
arg: Typed,
): LazyDelegate<() -> T> = Provider(arg.type, generic(), tag) { arg.value }
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.provider(
tag: Any? = null,
noinline fArg: () -> A,
): LazyDelegate<() -> T> = Provider(generic(), generic(), tag, fArg)
/**
* Gets a provider of `T` for the given type and tag, or null if none is found.
*
* T generics will be preserved!
*
* @param T The type of object the provider returns.
* @param tag The bound tag, if any.
* @return A provider, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
public inline fun DIAware.providerOrNull(tag: Any? = null): LazyDelegate<(() -> T)?> =
ProviderOrNull(generic(), tag)
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.providerOrNull(
tag: Any? = null,
arg: A,
): LazyDelegate<(() -> T)?> = ProviderOrNull(generic(), generic(), tag, { arg })
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.providerOrNull(
tag: Any? = null,
arg: Typed,
): LazyDelegate<(() -> T)?> = ProviderOrNull(arg.type, generic(), tag, { arg.value })
/**
* Gets a provider of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DIAware.providerOrNull(
tag: Any? = null,
noinline fArg: () -> A,
): LazyDelegate<(() -> T)?> = ProviderOrNull(generic(), generic(), tag, fArg)
/**
* Gets an instance of `T` for the given type and tag.
*
* T generics will be preserved!
*
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @return An instance.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun DIAware.instance(tag: Any? = null): LazyDelegate = Instance(generic(), tag)
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* A & T generics will be preserved!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instance(
tag: Any? = null,
arg: A,
): LazyDelegate = Instance(generic(), generic(), tag) { arg }
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instance(
tag: Any? = null,
arg: Typed,
): LazyDelegate = Instance(arg.type, generic(), tag) { arg.value }
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A].
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instance(
tag: Any? = null,
noinline fArg: () -> A,
): LazyDelegate = Instance(generic(), generic(), tag, fArg)
/**
* Gets an instance of `T` for the given type and tag, or null if none is found.
*
* T generics will be erased!
*
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @return An instance, or null if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun DIAware.instanceOrNull(tag: Any? = null): LazyDelegate =
InstanceOrNull(generic(), tag)
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instanceOrNull(
tag: Any? = null,
arg: A,
): LazyDelegate = InstanceOrNull(generic(), generic(), tag) { arg }
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instanceOrNull(
tag: Any? = null,
arg: Typed,
): LazyDelegate = InstanceOrNull(arg.type, generic(), tag) { arg.value }
/**
* Gets an instance of [T] for the given type and tag, curried from a factory that takes an argument [A], or null if none is found.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.instanceOrNull(
tag: Any? = null,
noinline fArg: () -> A,
): LazyDelegate = InstanceOrNull(generic(), generic(), tag, fArg)
/**
* Defines a context and its type to be used by DI.
*/
public inline fun diContext(context: C): DIContext = DIContext(generic(), context)
/**
* Defines a context and its type to be used by DI.
*/
public inline fun diContext(crossinline getContext: () -> C) : DIContext =
DIContext(generic(), getValue = { getContext() })
/**
* Allows to create a new DI object with a context and/or a trigger set.
*
* @param context The new context of the new DI.
* @param trigger The new trigger of the new DI.
* @return A DI object that uses the same container as this one, but with its context and/or trigger changed.
*/
public inline fun DIAware.on(
context: C,
trigger: DITrigger? = this.diTrigger,
): DI = On(diContext(context), trigger)
/**
* Allows to create a new DI object with a context and/or a trigger set.
*
* @param getContext A function that gets the new context of the new DI.
* @param trigger The new trigger of the new DI.
* @return A DI object that uses the same container as this one, but with its context and/or trigger changed.
*/
public inline fun DIAware.on(
trigger: DITrigger? = this.diTrigger,
crossinline getContext: () -> C,
): DI = On(diContext(getContext), trigger)
/**
* Allows to create a new DI object with a trigger set.
*
* @param trigger The new trigger of the new DI.
* @return A DI object that uses the same container as this one, but with its context and/or trigger changed.
*/
public fun DIAware.on(trigger: DITrigger?): DI = On(diContext, trigger)
//endregion
//region Direct retrieving
/**
* Gets a factory of `T` for the given argument type, return type and tag.
*
* A & T generics will be erased.
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @param tag The bound tag, if any.
* @return A factory.
* @throws DI.NotFoundException if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun DirectDIAware.factory(tag: Any? = null): (A) -> T =
directDI.Factory(generic(), generic(), tag)
/**
* Gets a factory of `T` for the given argument type, return type and tag, or nul if none is found.
*
* A & T generics will be erased.
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @param tag The bound tag, if any.
* @return A factory, or null if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun DirectDIAware.factoryOrNull(tag: Any? = null): ((A) -> T)? =
directDI.FactoryOrNull(generic(), generic(), tag)
/**
* Gets a provider of `T` for the given type and tag.
*
* T generics will be erased.
*
* @param T The type of object the provider returns.
* @param tag The bound tag, if any.
* @return A provider.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
public inline fun DirectDIAware.provider(tag: Any? = null): () -> T =
directDI.Provider(generic(), tag)
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of `T`.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.provider(
tag: Any? = null,
arg: A,
): () -> T = directDI.Provider(generic(), generic(), tag) { arg }
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of `T`.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.provider(
tag: Any? = null,
arg: Typed,
): () -> T = directDI.Provider(arg.type, generic(), tag) { arg.value }
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of `T`.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.provider(
tag: Any? = null,
noinline fArg: () -> A,
): () -> T = directDI.Provider(generic(), generic(), tag, fArg)
/**
* Gets a provider of `T` for the given type and tag, or null if none is found.
*
* T generics will be erased.
*
* @param T The type of object the provider returns.
* @param tag The bound tag, if any.
* @return A provider, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
@Suppress("UNCHECKED_CAST")
public inline fun DirectDIAware.providerOrNull(tag: Any? = null): (() -> T)? =
directDI.ProviderOrNull(generic(), tag)
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument, or null if none is found.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of `T`, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.providerOrNull(
tag: Any? = null,
arg: A,
): (() -> T)? = directDI.ProviderOrNull(generic(), generic(), tag) { arg }
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument, or null if none is found.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of `T`, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.providerOrNull(
tag: Any? = null,
arg: Typed,
): (() -> T)? = directDI.ProviderOrNull(arg.type, generic(), tag) { arg.value }
/**
* Gets a provider of `T` for the given type and tag, curried from a factory for the given argument, or null if none is found.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of `T`, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.providerOrNull(
tag: Any? = null,
noinline fArg: () -> A,
): (() -> T)? = directDI.ProviderOrNull(generic(), generic(), tag, fArg)
/**
* Gets an instance of `T` for the given type and tag.
*
* T generics will be erased.
*
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @return An instance.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instance(tag: Any? = null): T = directDI.Instance(generic(), tag)
/**
* Gets an instance of `T` for the given type and tag, curried from a factory for the given argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of `T`.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instance(tag: Any? = null, arg: A): T =
directDI.Instance(generic(), generic(), tag, arg)
/**
* Gets an instance of `T` for the given type and tag, curried from a factory for the given argument.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of `T`.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instance(tag: Any? = null, arg: Typed): T =
directDI.Instance(arg.type, generic(), tag, arg.value)
/**
* Gets an instance of `T` for the given type and tag, or null if none is found.
*
* T generics will be erased.
*
* @param T The type of object to retrieve.
* @param tag The bound tag, if any.
* @return An instance, or null if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instanceOrNull(tag: Any? = null): T? =
directDI.InstanceOrNull(generic(), tag)
/**
* Gets an instance of `T` for the given type and tag, curried from a factory for the given argument, or null if none is found.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return A instance of `T`, or null if no provider was found.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instanceOrNull(tag: Any? = null, arg: A): T? =
directDI.InstanceOrNull(generic(), generic(), tag, arg)
/**
* Gets an instance of `T` for the given type and tag, curried from a factory for the given argument, or null if none is found.
*
* A & T generics will be erased.
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param tag The bound tag, if any.
* @param arg A function that returns the argument that will be given to the factory when curried.
* @return A instance of `T`, or null if no provider was found.
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun DirectDIAware.instanceOrNull(tag: Any? = null, arg: Typed): T? =
directDI.InstanceOrNull(arg.type, generic(), tag, arg.value)
/**
* Returns a `DirectDI` with its context and/or receiver changed.
*
* @param context The new context for the new DirectDI.
* @param receiver The new receiver for the new DirectDI.
*/
public inline fun DirectDIAware.on(context: C): DirectDI = directDI.On(diContext(context))
//endregion
//region Named retrieving
/**
* Gets a factory of `T` for the given argument type and return type.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @return A factory.
* @throws DI.NotFoundException if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun Named.factory(): LazyDelegate<(A) -> T> =
Factory(generic(), generic())
/**
* Gets a factory of `T` for the given argument type and return type, or nul if none is found.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the factory takes.
* @param T The type of object the factory returns.
* @return A factory, or null if no factory was found.
* @throws DI.DependencyLoopException When calling the factory function, if the instance construction triggered a dependency loop.
*/
public inline fun Named.factoryOrNull(): LazyDelegate<((A) -> T)?> =
FactoryOrNull(generic(), generic())
/**
* Gets a provider of `T` for the given type.
* The name of the receiving property is used as tag.
*
* T generics will be erased!
*
* @param T The type of object the provider returns.
* @return A provider.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
public inline fun Named.provider(): LazyDelegate<() -> T> = Provider(generic())
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.provider(arg: A): LazyDelegate<() -> T> =
Provider(generic(), generic()) { arg }
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.provider(arg: Typed): LazyDelegate<() -> T> =
Provider(arg.type, generic()) { arg.value }
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.provider(noinline fArg: () -> A): LazyDelegate<() -> T> =
Provider(generic(), generic(), fArg)
/**
* Gets a provider of `T` for the given type, or null if none is found.
* The name of the receiving property is used as tag.
*
* T generics will be erased!
*
* @param T The type of object the provider returns.
* @return A provider, or null if no provider was found.
* @throws DI.DependencyLoopException When calling the provider function, if the instance construction triggered a dependency loop.
*/
public inline fun Named.providerOrNull(): LazyDelegate<(() -> T)?> = ProviderOrNull(generic())
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.providerOrNull(arg: A): LazyDelegate<(() -> T)?> =
ProviderOrNull(generic(), generic(), { arg })
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param arg The argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.providerOrNull(arg: Typed): LazyDelegate<(() -> T)?> =
ProviderOrNull(arg.type, generic(), { arg.value })
/**
* Gets a provider of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve with the returned provider.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return A provider of [T], or null if no factory was found.
* @throws DI.DependencyLoopException When calling the provider, if the value construction triggered a dependency loop.
*/
public inline fun Named.providerOrNull(noinline fArg: () -> A): LazyDelegate<(() -> T)?> =
ProviderOrNull(generic(), generic(), fArg)
/**
* Gets an instance of `T` for the given type.
* The name of the receiving property is used as tag.
*
* T generics will be erased!
*
* @param T The type of object to retrieve.
* @return An instance.
* @throws DI.NotFoundException if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun Named.instance(): LazyDelegate = Instance(generic())
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instance(arg: A): LazyDelegate =
Instance(generic(), generic()) { arg }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instance(arg: Typed): LazyDelegate =
Instance(arg.type, generic()) { arg.value }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A].
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instance(noinline fArg: () -> A): LazyDelegate =
Instance(generic(), generic(), fArg)
/**
* Gets an instance of `T` for the given type, or null if none is found.
* The name of the receiving property is used as tag.
*
* T generics will be erased!
*
* @param T The type of object to retrieve.
* @return An instance, or null if no provider was found.
* @throws DI.DependencyLoopException If the instance construction triggered a dependency loop.
*/
public inline fun Named.instanceOrNull(): LazyDelegate = InstanceOrNull(generic())
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instanceOrNull(arg: A): LazyDelegate =
InstanceOrNull(generic(), generic()) { arg }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* The argument type is extracted from the `Typed.type` of the argument.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param arg The argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instanceOrNull(arg: Typed): LazyDelegate =
InstanceOrNull(arg.type, generic()) { arg.value }
/**
* Gets an instance of [T] for the given type, curried from a factory that takes an argument [A], or null if none is found.
* The name of the receiving property is used as tag.
*
* A & T generics will be erased!
*
* @param A The type of argument the curried factory takes.
* @param T The type of object to retrieve.
* @param fArg A function that returns the argument that will be given to the factory when curried.
* @return An instance of [T], or null if no factory was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun Named.instanceOrNull(noinline fArg: () -> A): LazyDelegate =
InstanceOrNull(generic(), generic(), fArg)
/**
* Gets a constant of type [T] and tag whose tag is the name of the receiving property.
*
* T generics will be erased!
*
* @param T The type of object to retrieve.
* @return An instance of [T].
* @throws DI.NotFoundException If no provider was found.
* @throws DI.DependencyLoopException If the value construction triggered a dependency loop.
*/
public inline fun DIAware.constant(): LazyDelegate = Constant(generic())
//endregion
© 2015 - 2024 Weber Informatics LLC | Privacy Policy