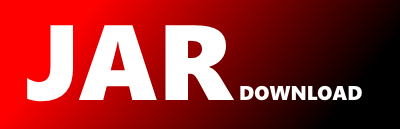
org.kohsuke.stapler.jelly.groovy.SimpleTemplateParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stapler-groovy Show documentation
Show all versions of stapler-groovy Show documentation
Groovy binding for Stapler
The newest version!
/*
* Copyright 2003-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kohsuke.stapler.jelly.groovy;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
import java.io.StringWriter;
import java.net.URL;
/**
* @author Kohsuke Kawaguchi
*/
class SimpleTemplateParser {
@SuppressFBWarnings(value = "URLCONNECTION_SSRF_FD", justification = "Not relevant in this situation.")
protected String parse(URL res) throws IOException {
return parse(new InputStreamReader(res.openStream(),"UTF-8"));
}
/**
* Parse the text document looking for <% or <%= and then call out to the appropriate handler, otherwise copy the text directly
* into the script while escaping quotes.
*
* @param reader a reader for the template text
* @return the parsed text
* @throws IOException if something goes wrong
*/
protected String parse(Reader reader) throws IOException {
if (!reader.markSupported()) {
reader = new BufferedReader(reader);
}
StringWriter sw = new StringWriter();
startScript(sw);
int c;
while ((c = reader.read()) != -1) {
if (c == '<') {
reader.mark(1);
c = reader.read();
if (c != '%') {
sw.write('<');
reader.reset();
} else {
reader.mark(1);
c = reader.read();
if (c == '=') {
groovyExpression(reader, sw);
} else {
reader.reset();
groovySection(reader, sw);
}
}
continue; // at least '<' is consumed ... read next chars.
}
if (c == '$') {
reader.mark(1);
c = reader.read();
if (c != '{') {
sw.write('$');
reader.reset();
} else {
reader.mark(1);
sw.write("${");
processGSstring(reader, sw);
}
continue; // at least '$' is consumed ... read next chars.
}
if (c == '\"') {
sw.write('\\');
}
/*
* Handle raw new line characters.
*/
if (c == '\n' || c == '\r') {
if (c == '\r') { // on Windows, "\r\n" is a new line.
reader.mark(1);
c = reader.read();
if (c != '\n') {
reader.reset();
}
}
sw.write("\n");
continue;
}
sw.write(c);
}
endScript(sw);
return sw.toString();
}
protected String printCommand() {
return "out.print";
}
private void startScript(StringWriter sw) {
sw.write(printCommand()+"(\"\"\"");
}
private void endScript(StringWriter sw) {
sw.write("\"\"\");\n");
sw.write("\n/* Generated by SimpleTemplateEngine */");
}
private void processGSstring(Reader reader, StringWriter sw) throws IOException {
int c;
while ((c = reader.read()) != -1) {
if (c != '\n' && c != '\r') {
sw.write(c);
}
if (c == '}') {
break;
}
}
}
/**
* Closes the currently open write and writes out the following text as a GString expression until it reaches an end %>.
*
* @param reader a reader for the template text
* @param sw a StringWriter to write expression content
* @throws IOException if something goes wrong
*/
private void groovyExpression(Reader reader, StringWriter sw) throws IOException {
sw.write("${");
int c;
while ((c = reader.read()) != -1) {
if (c == '%') {
c = reader.read();
if (c != '>') {
sw.write('%');
} else {
break;
}
}
if (c != '\n' && c != '\r') {
sw.write(c);
}
}
sw.write("}");
}
/**
* Closes the currently open write and writes the following text as normal Groovy script code until it reaches an end %>.
*
* @param reader a reader for the template text
* @param sw a StringWriter to write expression content
* @throws IOException if something goes wrong
*/
private void groovySection(Reader reader, StringWriter sw) throws IOException {
sw.write("\"\"\");");
int c;
while ((c = reader.read()) != -1) {
if (c == '%') {
c = reader.read();
if (c != '>') {
sw.write('%');
} else {
break;
}
}
/* Don't eat EOL chars in sections - as they are valid instruction separators.
* See http://jira.codehaus.org/browse/GROOVY-980
*/
// if (c != '\n' && c != '\r') {
sw.write(c);
//}
}
sw.write(";\n"+printCommand()+"(\"\"\"");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy