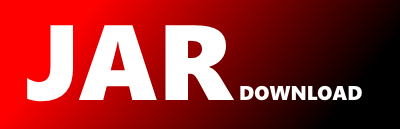
org.kohsuke.github.GHUser Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright (c) 2010, Kohsuke Kawaguchi
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.kohsuke.github;
import com.infradna.tool.bridge_method_injector.WithBridgeMethods;
import java.io.IOException;
import java.util.*;
import static org.kohsuke.github.internal.Previews.INERTIA;
// TODO: Auto-generated Javadoc
/**
* Represents an user of GitHub.
*
* @author Kohsuke Kawaguchi
*/
public class GHUser extends GHPerson {
/** The ldap dn. */
protected String ldap_dn;
/**
* Gets keys.
*
* @return the keys
* @throws IOException
* the io exception
*/
public List getKeys() throws IOException {
return root().createRequest().withUrlPath(getApiTailUrl("keys")).toIterable(GHKey[].class, null).toList();
}
/**
* Follow this user.
*
* @throws IOException
* the io exception
*/
public void follow() throws IOException {
root().createRequest().method("PUT").withUrlPath("/user/following/" + login).send();
}
/**
* Unfollow this user.
*
* @throws IOException
* the io exception
*/
public void unfollow() throws IOException {
root().createRequest().method("DELETE").withUrlPath("/user/following/" + login).send();
}
/**
* Lists the users that this user is following.
*
* @return the follows
* @throws IOException
* the io exception
*/
@WithBridgeMethods(Set.class)
public GHPersonSet getFollows() throws IOException {
return new GHPersonSet(listFollows().toList());
}
/**
* Lists the users that this user is following.
*
* @return the paged iterable
*/
public PagedIterable listFollows() {
return listUser("following");
}
/**
* Lists the users who are following this user.
*
* @return the followers
* @throws IOException
* the io exception
*/
@WithBridgeMethods(Set.class)
public GHPersonSet getFollowers() throws IOException {
return new GHPersonSet(listFollowers().toList());
}
/**
* Lists the users who are following this user.
*
* @return the paged iterable
*/
public PagedIterable listFollowers() {
return listUser("followers");
}
private PagedIterable listUser(final String suffix) {
return root().createRequest().withUrlPath(getApiTailUrl(suffix)).toIterable(GHUser[].class, null);
}
/**
* Lists all the subscribed (aka watched) repositories.
*
* https://developer.github.com/v3/activity/watching/
*
* @return the paged iterable
*/
public PagedIterable listSubscriptions() {
return listRepositories("subscriptions");
}
/**
* Lists all the repositories that this user has starred.
*
* @return the paged iterable
*/
public PagedIterable listStarredRepositories() {
return listRepositories("starred");
}
/**
* Lists all the projects.
*
* https://docs.github.com/en/rest/reference/projects#list-user-projects
*
* @return the paged iterable
*/
@Preview(INERTIA)
public PagedIterable listProjects() {
return root().createRequest()
.withPreview(INERTIA)
.withUrlPath(getApiTailUrl("projects"))
.toIterable(GHProject[].class, null);
}
private PagedIterable listRepositories(final String suffix) {
return root().createRequest().withUrlPath(getApiTailUrl(suffix)).toIterable(GHRepository[].class, null);
}
/**
* Returns true if this user belongs to the specified organization.
*
* @param org
* the org
* @return the boolean
*/
public boolean isMemberOf(GHOrganization org) {
return org.hasMember(this);
}
/**
* Returns true if this user belongs to the specified team.
*
* @param team
* the team
* @return the boolean
*/
public boolean isMemberOf(GHTeam team) {
return team.hasMember(this);
}
/**
* Returns true if this user belongs to the specified organization as a public member.
*
* @param org
* the org
* @return the boolean
*/
public boolean isPublicMemberOf(GHOrganization org) {
return org.hasPublicMember(this);
}
/**
* Returns true if this user is marked as hireable, false otherwise.
*
* @return if the user is marked as hireable
*/
public boolean isHireable() {
return hireable;
}
/**
* Gets the bio.
*
* @return the bio
*/
public String getBio() {
return bio;
}
/**
* Gets the organization that this user belongs to publicly.
*
* @return the organizations
* @throws IOException
* the io exception
*/
@WithBridgeMethods(Set.class)
public GHPersonSet getOrganizations() throws IOException {
GHPersonSet orgs = new GHPersonSet();
Set names = new HashSet();
for (GHOrganization o : root().createRequest()
.withUrlPath("/users/" + login + "/orgs")
.toIterable(GHOrganization[].class, null)
.toArray()) {
if (names.add(o.getLogin())) // I've seen some duplicates in the data
orgs.add(root().getOrganization(o.getLogin()));
}
return orgs;
}
/**
* Lists events performed by a user (this includes private events if the caller is authenticated.
*
* @return the paged iterable
* @throws IOException
* Signals that an I/O exception has occurred.
*/
public PagedIterable listEvents() throws IOException {
return root().createRequest()
.withUrlPath(String.format("/users/%s/events", login))
.toIterable(GHEventInfo[].class, null);
}
/**
* Lists Gists created by this user.
*
* @return the paged iterable
* @throws IOException
* the io exception
*/
public PagedIterable listGists() throws IOException {
return root().createRequest()
.withUrlPath(String.format("/users/%s/gists", login))
.toIterable(GHGist[].class, null);
}
/**
* Gets LDAP information for user.
*
* @return The LDAP information
* @throws IOException
* the io exception
* @see Github
* LDAP
*/
public Optional getLdapDn() throws IOException {
super.populate();
return Optional.ofNullable(ldap_dn);
}
/**
* Hash code.
*
* @return the int
*/
@Override
public int hashCode() {
return login.hashCode();
}
/**
* Equals.
*
* @param obj
* the obj
* @return true, if successful
*/
@Override
public boolean equals(Object obj) {
if (obj instanceof GHUser) {
GHUser that = (GHUser) obj;
return this.login.equals(that.login);
}
return false;
}
/**
* Gets the api tail url.
*
* @param tail
* the tail
* @return the api tail url
*/
String getApiTailUrl(String tail) {
if (tail.length() > 0 && !tail.startsWith("/"))
tail = '/' + tail;
return "/users/" + login + tail;
}
}