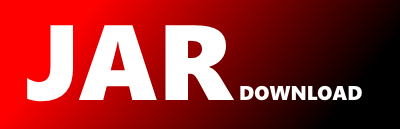
org.kohsuke.youdebug.BundledWatchpointRequest.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of youdebug Show documentation
Show all versions of youdebug Show documentation
YouDebug is a non-interactive debugger scripted by Groovy to assist
remote troubleshooting and data collection to analyze failures.
The newest version!
package org.kohsuke.youdebug;
import com.sun.jdi.request.WatchpointRequest;
import com.sun.jdi.request.InvalidRequestStateException;
import com.sun.jdi.request.ClassPrepareRequest;
import com.sun.jdi.ThreadReference;
import com.sun.jdi.ReferenceType;
import com.sun.jdi.VirtualMachine;
import com.sun.jdi.ObjectReference;
import java.util.List;
/**
* Multiple {@link WatchpointRequest}s bundled to behave as one {@link WatchpointRequest}.
*
* @author Kohsuke Kawaguchi
*/
public class BundledWatchpointRequest extends BundledEventRequestWithClassPrepare {
BundledWatchpointRequest(ClassPrepareRequest req, List requests) {
super(req, requests);
}
/**
* Restricts the events generated by this request to those in
* the given thread.
* @param thread the thread to filter on.
* @throws InvalidRequestStateException if this request is currently
* enabled or has been deleted.
* Filters may be added only to disabled requests.
*/
public void addThreadFilter(ThreadReference thread) {
requests*.addThreadFilter(thread);
}
/**
* Restricts the events generated by this request to those whose
* location is in the given reference type or any of its subtypes.
* An event will be generated for any location in a reference type
* that can be safely cast to the given reference type.
*
* @param refType the reference type to filter on.
* @throws InvalidRequestStateException if this request is currently
* enabled or has been deleted.
* Filters may be added only to disabled requests.
*/
public void addClassFilter(ReferenceType refType) {
requests*.addClassFilter(refType);
}
/**
* Restricts the events generated by this request to those
* whose location is in a class whose name matches a restricted
* regular expression. Regular expressions are limited
* to exact matches and patterns that begin with '*' or end with '*';
* for example, "*.Foo" or "java.*".
*
* @param classPattern the pattern String to filter for.
* @throws InvalidRequestStateException if this request is currently
* enabled or has been deleted.
* Filters may be added only to disabled requests.
*/
public void addClassFilter(String classPattern) {
requests*.addClassFilter(classPattern);
}
/**
* Restricts the events generated by this request to those
* whose location is in a class whose name does not match this
* restricted regular expression. Regular expressions are limited
* to exact matches and patterns that begin with '*' or end with '*';
* for example, "*.Foo" or "java.*".
*
* @param classPattern the pattern String to filter against.
* @throws InvalidRequestStateException if this request is currently
* enabled or has been deleted.
* Filters may be added only to disabled requests.
*/
public void addClassExclusionFilter(String classPattern) {
requests*.addClassExclusionFilter(classPattern);
}
/**
* Restricts the events generated by this request to those in
* which the currently executing instance ("this") is the object
* specified.
*
* Not all targets support this operation.
* Use {@link VirtualMachine#canUseInstanceFilters()}
* to determine if the operation is supported.
* @since 1.4
* @param instance the object which must be the current instance
* in order to pass this filter.
* @throws java.lang.UnsupportedOperationException if
* the target virtual machine does not support this
* operation.
* @throws InvalidRequestStateException if this request is currently
* enabled or has been deleted.
* Filters may be added only to disabled requests.
*/
public void addInstanceFilter(ObjectReference instance) {
requests*.addInstanceFilter(instance);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy