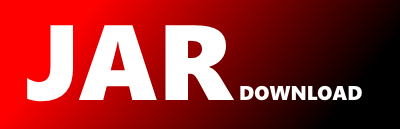
org.kohsuke.youdebug.BundledWatchpointRequest Maven / Gradle / Ivy
Show all versions of youdebug Show documentation
//
// Generated stub from file:/home/kohsuke/ws/youdebug/target/checkout/src/main/groovy/org/kohsuke/youdebug/BundledWatchpointRequest.groovy
//
package org.kohsuke.youdebug;
import java.lang.*;
import java.io.*;
import java.net.*;
import java.util.*;
import groovy.lang.*;
import groovy.util.*;
import java.math.BigDecimal;
import java.math.BigInteger;
import com.sun.jdi.request.WatchpointRequest;
import com.sun.jdi.request.InvalidRequestStateException;
import com.sun.jdi.request.ClassPrepareRequest;
import com.sun.jdi.ThreadReference;
import com.sun.jdi.ReferenceType;
import com.sun.jdi.VirtualMachine;
import com.sun.jdi.ObjectReference;
import java.util.List;
/**
* Multiple {@link WatchpointRequest}s bundled to behave as one {@link WatchpointRequest}.
*
* @author Kohsuke Kawaguchi
*/
public class BundledWatchpointRequest
extends BundledEventRequestWithClassPrepare
implements groovy.lang.GroovyObject
{
/**
* Magic constructor
*/
private BundledWatchpointRequest(java.lang.Void void0, java.lang.Void void1, java.lang.Void void2) {
super((ClassPrepareRequest)null, (List)null);
throw new InternalError("Stubbed method");
}
public BundledWatchpointRequest(ClassPrepareRequest req, List requests) {
this((java.lang.Void)null, (java.lang.Void)null, (java.lang.Void)null);
throw new InternalError("Stubbed method");
}
/**
* Restricts the events generated by this request to those in
* the given thread.
*
* @param thread the thread to filter on.
* @throws InvalidRequestStateException if this request is currently
enabled or has been deleted.
Filters may be added only to disabled requests.
*/
public void addThreadFilter(ThreadReference thread) {
throw new InternalError("Stubbed method");
}
/**
* Restricts the events generated by this request to those whose
* location is in the given reference type or any of its subtypes.
* An event will be generated for any location in a reference type
* that can be safely cast to the given reference type.
*
* @param refType the reference type to filter on.
* @throws InvalidRequestStateException if this request is currently
enabled or has been deleted.
Filters may be added only to disabled requests.
*/
public void addClassFilter(ReferenceType refType) {
throw new InternalError("Stubbed method");
}
/**
* Restricts the events generated by this request to those
* whose location is in a class whose name matches a restricted
* regular expression. Regular expressions are limited
* to exact matches and patterns that begin with '*' or end with '*';
* for example, "*.Foo" or "java.*".
*
* @param classPattern the pattern String to filter for.
* @throws InvalidRequestStateException if this request is currently
enabled or has been deleted.
Filters may be added only to disabled requests.
*/
public void addClassFilter(java.lang.String classPattern) {
throw new InternalError("Stubbed method");
}
/**
* Restricts the events generated by this request to those
* whose location is in a class whose name does not match this
* restricted regular expression. Regular expressions are limited
* to exact matches and patterns that begin with '*' or end with '*';
* for example, "*.Foo" or "java.*".
*
* @param classPattern the pattern String to filter against.
* @throws InvalidRequestStateException if this request is currently
enabled or has been deleted.
Filters may be added only to disabled requests.
*/
public void addClassExclusionFilter(java.lang.String classPattern) {
throw new InternalError("Stubbed method");
}
/**
* Restricts the events generated by this request to those in
* which the currently executing instance ("this") is the object
* specified.
*
* Not all targets support this operation.
* Use {@link VirtualMachine#canUseInstanceFilters()}
* to determine if the operation is supported.
*
* @since 1.4
* @param instance the object which must be the current instance
in order to pass this filter.
* @throws java.lang.UnsupportedOperationException if
the target virtual machine does not support this
operation.
* @throws InvalidRequestStateException if this request is currently
enabled or has been deleted.
Filters may be added only to disabled requests.
*/
public void addInstanceFilter(ObjectReference instance) {
throw new InternalError("Stubbed method");
}
public groovy.lang.MetaClass getMetaClass() {
throw new InternalError("Stubbed method");
}
public void setMetaClass(groovy.lang.MetaClass metaClass) {
throw new InternalError("Stubbed method");
}
public java.lang.Object invokeMethod(java.lang.String name, java.lang.Object args) {
throw new InternalError("Stubbed method");
}
public java.lang.Object getProperty(java.lang.String name) {
throw new InternalError("Stubbed method");
}
public void setProperty(java.lang.String name, java.lang.Object value) {
throw new InternalError("Stubbed method");
}
}